Hi rani,
Check this example. Now please take its reference and correct your code.
For this example i have used angular-drag-and-drop-lists.js file.
You can can be downloaded from GitHub using the following link.
Download angular-drag-and-drop-lists
Database
I have made use of the following table HolidayLocations with the schema as follows.
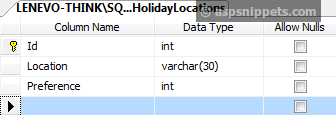
I have already inserted few records in the table.
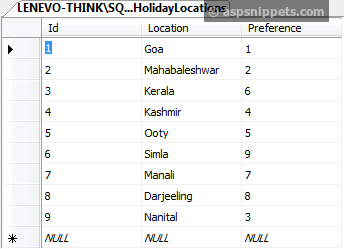
You can download the database table SQL by clicking the download link below.
Download SQL file
HTML
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.2/css/bootstrap.min.css" />
<script type="text/javascript" src="//ajax.googleapis.com/ajax/libs/angularjs/1.3.9/angular.min.js"></script>
<script src="angular-drag-and-drop-lists.js" type="text/javascript"></script>
<script type="text/javascript">
var app = angular.module('MyApp', ['dndLists']);
app.controller('MyController', ['$scope', '$http', function ($scope, $http) {
$scope.Locations = [];
$scope.PopulateLocations = function () {
$http.post('Default.aspx/GetLocations', { headers: { 'Content-Type': 'application/json'} })
.then(function (response) {
$scope.Locations = response.data.d;
});
}
$scope.PopulateLocations();
$scope.Sorting = function (index) {
$scope.Locations.splice(index, 1);
var newOrders = [];
angular.forEach($scope.Locations, function (val, index) {
val.Preference = index + 1;
newOrders.push(val);
});
$http.post('Default.aspx/UpdateLocations', { reorderLocations: newOrders }, { headers: { 'Content-Type': 'application/json'} })
.then(function (response) {
$scope.Locations = response.data.d;
});
}
} ]);
</script>
<div ng-app="MyApp" ng-controller="MyController">
<table dnd-list="Locations" class="table table-striped table-bordered table-hover table-condensed">
<tr class="danger">
<th>Id</th>
<th>Location</th>
<th>Preference</th>
</tr>
<tbody ng-repeat="item in Locations" dnd-list-id="{{item.Id}}" dnd-draggable="item"
dnd-moved="Sorting($index)" dnd-effect-allowed="move" dnd-selected="models.selected = item"
ng-class="{'selected': models.selected === item}" style="cursor: move">
<tr>
<td>{{item.Id}}</td>
<td>{{item.Location}}</td>
<td>{{item.Preference}}</td>
</tr>
</tbody>
</table>
</div>
Code
C#
[System.Web.Services.WebMethod]
public static List<HolidayLocation> GetLocations()
{
TestEntities entities = new TestEntities();
List<HolidayLocation> locations = entities.HolidayLocations.OrderBy(x => x.Preference).ToList();
return locations;
}
[System.Web.Services.WebMethod]
public static List<HolidayLocation> UpdateLocations(List<HolidayLocation> reorderLocations)
{
TestEntities entities = new TestEntities();
foreach (HolidayLocation newLocations in reorderLocations)
{
HolidayLocation oldLocation = entities.HolidayLocations.Where(x => x.Id == newLocations.Id).FirstOrDefault();
// Updating new Preference.
oldLocation.Preference = newLocations.Preference;
entities.SaveChanges();
}
return entities.HolidayLocations.OrderBy(x => x.Preference).ToList();
}
VB.Net
<Services.WebMethod()>
Public Shared Function GetLocations() As List(Of HolidayLocation)
Dim entities As TestEntities = New TestEntities()
Dim locations As List(Of HolidayLocation) = entities.HolidayLocations.OrderBy(Function(x) x.Preference).ToList()
Return locations
End Function
<Services.WebMethod()>
Public Shared Function SaveLocations(ByVal reorderLocations As List(Of HolidayLocation)) As List(Of HolidayLocation)
Dim entities As TestEntities = New TestEntities()
For Each newHolidayLocation As HolidayLocation In reorderLocations
Dim oldHolidayLocation As HolidayLocation = entities.HolidayLocations.Where(Function(x) x.Id = newHolidayLocation.Id).FirstOrDefault()
' Updating new Preference.
oldHolidayLocation.Preference = newHolidayLocation.Preference
entities.SaveChanges()
Next
Return entities.HolidayLocations.OrderBy(Function(x) x.Preference).ToList()
End Function
Screenshots

Updated Database Preference
