Hi micah,
Check this example. Now please take its reference and correct your code.
Database
I have made use of the following table Customers with the schema as follows.
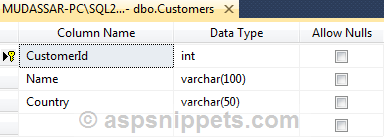
I have already inserted few records in the table.
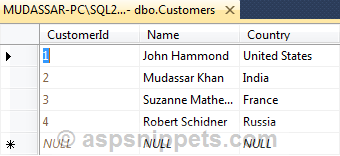
You can download the database table SQL by clicking the download link below.
Download SQL file
HTML
<asp:TextBox ID="TextBox1" runat="server" placeholder="Insert Itemno"></asp:TextBox>
<asp:DropDownList ID="ddlCountries" runat="server" multiple="multiple"
CssClass="multiselect" data-placeholder="Select Country">
</asp:DropDownList>
<asp:HiddenField ID="hfSelected" runat="server" />
<asp:Button Text="Filter" runat="server" CssClass="btn btn-info" OnClick="Filter" />
<script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/jquery/1.8.3/jquery.min.js"></script>
<link rel="stylesheet" type="text/css" href="https://cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/3.0.3/css/bootstrap.min.css" />
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/3.0.3/js/bootstrap.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/bootstrap-multiselect/0.9.13/js/bootstrap-multiselect.js"></script>
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/bootstrap-multiselect/0.9.13/css/bootstrap-multiselect.css" />
<script type="text/javascript">
$(function () {
$('[id*=ddlCountries]').multiselect({ includeSelectAllOption: true });
$('[id*=ddlCountries]').on('change', function () {
var selectedText = [];
$("[id*=ddlCountries] option:selected").each(function (index, item) {
selectedText.push(item.text);
});
$("[id*=hfSelected]").val(selectedText.join(','));
});
});
</script>
Namespaces
C#
using System.Configuration;
using System.Data;
using System.Data.SqlClient;
VB.Net
Imports System.Configuration
Imports System.Data
Imports System.Data.SqlClient
Code
C#
protected void Page_Load(object sender, EventArgs e)
{
if (!this.IsPostBack)
{
string conStr = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
SqlConnection con = new SqlConnection(conStr);
DataSet ds = new DataSet();
try
{
con.Open();
string sql = "SELECT CustomerID, Name FROM Customers";
SqlDataAdapter da = new SqlDataAdapter(sql, con);
da.Fill(ds);
ddlCountries.DataSource = ds;
ddlCountries.DataTextField = "Name";
ddlCountries.DataValueField = "CustomerID";
ddlCountries.DataBind();
}
catch (Exception ex)
{
Console.WriteLine(ex.Message.ToString());
}
finally
{
ds.Dispose();
con.Close();
}
}
}
protected void Filter(object sender, EventArgs e)
{
int inserted = 0;
foreach (string item in hfSelected.Value.Split(','))
{
using (SqlConnection con = new SqlConnection())
{
con.ConnectionString = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
using (SqlCommand cmd = new SqlCommand())
{
cmd.CommandType = CommandType.Text;
cmd.Connection = con;
cmd.CommandText = "INSERT INTO Details (ITEMID,DETAIL) VALUES(@ITEMID,@DETAIL)";
cmd.Parameters.AddWithValue("@ITEMID", TextBox1.Text.Trim());
cmd.Parameters.AddWithValue("@DETAIL", item.Trim());
con.Open();
inserted += cmd.ExecuteNonQuery();
con.Close();
}
}
}
}
VB.Net
Protected Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs) Handles Me.Load
If Not Me.IsPostBack Then
Dim conStr As String = ConfigurationManager.ConnectionStrings("constr").ConnectionString
Dim con As SqlConnection = New SqlConnection(conStr)
Dim ds As DataSet = New DataSet()
Try
con.Open()
Dim sql As String = "SELECT CustomerID, Name FROM Customers"
Dim da As SqlDataAdapter = New SqlDataAdapter(sql, con)
da.Fill(ds)
ddlCountries.DataSource = ds
ddlCountries.DataTextField = "Name"
ddlCountries.DataValueField = "CustomerID"
ddlCountries.DataBind()
Catch ex As Exception
Console.WriteLine(ex.Message.ToString())
Finally
ds.Dispose()
con.Close()
End Try
End If
End Sub
Protected Sub Filter(ByVal sender As Object, ByVal e As EventArgs)
Dim inserted As Integer = 0
For Each item As String In hfSelected.Value.Split(","c)
Using con As SqlConnection = New SqlConnection()
con.ConnectionString = ConfigurationManager.ConnectionStrings("constr").ConnectionString
Using cmd As SqlCommand = New SqlCommand()
cmd.CommandType = CommandType.Text
cmd.Connection = con
cmd.CommandText = "INSERT INTO Details (ITEMID,DETAIL) VALUES(@ITEMID,@DETAIL)"
cmd.Parameters.AddWithValue("@ITEMID", TextBox1.Text.Trim())
cmd.Parameters.AddWithValue("@DETAIL", item.Trim())
con.Open()
inserted += cmd.ExecuteNonQuery()
con.Close()
End Using
End Using
Next
End Sub