Hi moepyag,
Refer below sample.
For more details on populating the DropDownList refer below article.
ASP.Net Core: DropDownList with Entity Framework Tutorial with example
Database
I have made use of the following table Customers with the schema as follows.
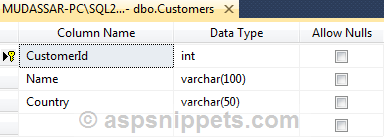
I have already inserted few records in the table.
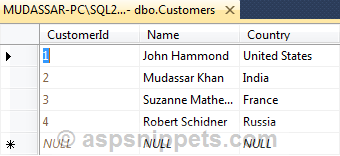
You can download the database table SQL by clicking the download link below.
Download SQL file
Model
public class CustomerModel
{
[Key]
public int CustomerId { get; set; }
public string Name { get; set; }
}
Controller
public class HomeController : Controller
{
private DBCtx Context { get; }
public HomeController(DBCtx _context)
{
this.Context = _context;
}
public IActionResult Index()
{
SelectList selectList = new SelectList(this.Context.Customers.ToList(), "CustomerId", "Name");
return View(selectList);
}
[HttpPost]
public IActionResult Index(int customerId, string name)
{
// Return List of Items with selected value to retain the selection.
SelectList selectList = new SelectList(this.Context.Customers.ToList(), "CustomerId", "Name", customerId);
ViewBag.Message = "Name: " + name + "\\nID: " + customerId;
return View(selectList);
}
}
View
@model SelectList
@addTagHelper*, Microsoft.AspNetCore.Mvc.TagHelpers
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Index</title>
</head>
<body>
<form method="post" asp-controller="Home" asp-action="Index">
Name:
<select id="ddlCustomers" name="customerId" asp-items="Model">
<option value="0">Please select</option>
</select>
<input type="hidden" name="name" />
<input type="submit" value="Submit" />
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.7.1/jquery.min.js"></script>
<script type="text/javascript">
$(function(){
$("#ddlCustomers").on("change", function () {
$("input[name=name]").val($(this).find("option:selected").text());
});
});
</script>
@if (ViewBag.Message != null)
{
<script type="text/javascript">
$(function(){
alert("@ViewBag.Message");
});
</script>
}
</form>
</body>
</html>
Screenshot
