Hi samsmuthu,
In VB.Net you need to use AddHandler statement like below.
AddHandler event, AddressOf eventhandler
Refer the sample code.
Database
I have made use of the following table Customers with the schema as follows.
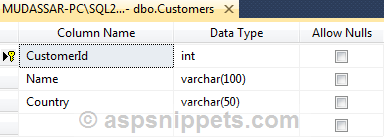
I have already inserted few records in the table.
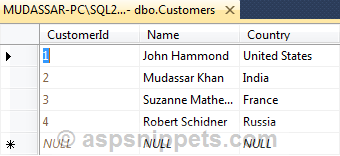
You can download the database table SQL by clicking the download link below.
Download SQL file
HTML
<asp:ScriptManager runat="server" />
<rsweb:ReportViewer ID="ReportViewer1" runat="server" Width="300" Height="300"></rsweb:ReportViewer>
<br />
<asp:Button Text="Print" runat="server" OnClick="Print" />
Namespaces
C#
using System.Configuration;
using System.Data.SqlClient;
using System.Drawing.Imaging;
using System.Drawing.Printing;
using System.IO;
using System.Text;
using Microsoft.Reporting.WebForms;
VB.Net
Imports System.Configuration
Imports System.Data.SqlClient
Imports System.Drawing.Imaging
Imports System.Drawing.Printing
Imports System.IO
Imports System.Text
Imports Microsoft.Reporting.WebForms
Code
C#
private int pageIndex = 0;
private IList<Stream> streams;
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
ReportViewer1.ProcessingMode = ProcessingMode.Local;
ReportViewer1.LocalReport.ReportPath = Server.MapPath("~/Report.rdlc");
Customers dsCustomers = GetData("select top 5 * from customers");
ReportDataSource datasource = new ReportDataSource("Customers", dsCustomers.Tables[0]);
ReportViewer1.LocalReport.DataSources.Clear();
ReportViewer1.LocalReport.DataSources.Add(datasource);
}
}
protected void Print(object sender, EventArgs e)
{
Export(ReportViewer1.LocalReport);
pageIndex = 0;
Print();
}
private void Export(LocalReport report)
{
string deviceInfo =
"<DeviceInfo>" +
" <OutputFormat>EMF</OutputFormat>" +
" <PageWidth>8.5in</PageWidth>" +
" <PageHeight>11in</PageHeight>" +
" <MarginTop>0.25in</MarginTop>" +
" <MarginLeft>0.25in</MarginLeft>" +
" <MarginRight>0.25in</MarginRight>" +
" <MarginBottom>0.25in</MarginBottom>" +
"</DeviceInfo>";
Warning[] warnings;
streams = new List<Stream>();
report.Render("Image", deviceInfo, CreateStream, out warnings);
foreach (Stream stream in streams)
{
stream.Position = 0;
}
}
private Stream CreateStream(string name, string fileNameExtension, Encoding encoding, string mimeType, bool willSeek)
{
Stream stream = new FileStream(Server.MapPath("~/Files/") + name + DateTime.Now.ToString("ddMMyyyyHHMMss") + "." + fileNameExtension, FileMode.Create);
streams.Add(stream);
return stream;
}
private void Print()
{
if (streams == null || streams.Count == 0)
{
return;
}
PrintDocument printDoc = new PrintDocument();
printDoc.PrintPage += new PrintPageEventHandler(PrintPage);
printDoc.Print();
}
private void PrintPage(object sender, PrintPageEventArgs ev)
{
Metafile pageImage = new Metafile(streams[pageIndex]);
ev.Graphics.DrawImage(pageImage, ev.PageBounds);
pageIndex++;
ev.HasMorePages = (pageIndex < streams.Count);
}
private Customers GetData(string query)
{
string conString = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
SqlCommand cmd = new SqlCommand(query);
using (SqlConnection con = new SqlConnection(conString))
{
using (SqlDataAdapter sda = new SqlDataAdapter())
{
cmd.Connection = con;
sda.SelectCommand = cmd;
using (Customers dsCustomers = new Customers())
{
sda.Fill(dsCustomers, "DataTable1");
return dsCustomers;
}
}
}
}
VB.Net
Private pageIndex As Integer = 0
Private streams As IList(Of Stream)
Protected Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs) Handles Me.Load
If Not IsPostBack Then
ReportViewer1.ProcessingMode = ProcessingMode.Local
ReportViewer1.LocalReport.ReportPath = Server.MapPath("~/Report.rdlc")
Dim dsCustomers As Customers = GetData("select top 5 * from customers")
Dim datasource As ReportDataSource = New ReportDataSource("Customers", dsCustomers.Tables(0))
ReportViewer1.LocalReport.DataSources.Clear()
ReportViewer1.LocalReport.DataSources.Add(datasource)
End If
End Sub
Protected Sub Print(ByVal sender As Object, ByVal e As EventArgs)
Export(ReportViewer1.LocalReport)
pageIndex = 0
Print()
End Sub
Private Sub Export(ByVal report As LocalReport)
Dim deviceInfo As String = "<DeviceInfo>" &
" <OutputFormat>EMF</OutputFormat>" &
" <PageWidth>8.5in</PageWidth>" &
" <PageHeight>11in</PageHeight>" &
" <MarginTop>0.25in</MarginTop>" &
" <MarginLeft>0.25in</MarginLeft>" &
" <MarginRight>0.25in</MarginRight>" &
" <MarginBottom>0.25in</MarginBottom>" &
"</DeviceInfo>"
Dim warnings As Warning()
streams = New List(Of Stream)()
report.Render("Image", deviceInfo, AddressOf CreateStream, warnings)
For Each stream As Stream In streams
stream.Position = 0
Next
End Sub
Private Function CreateStream(ByVal name As String, ByVal fileNameExtension As String, ByVal encoding As Encoding, ByVal mimeType As String, ByVal willSeek As Boolean) As Stream
Dim stream As Stream = New FileStream(Server.MapPath("~/Files/") & name & DateTime.Now.ToString("ddMMyyyyHHMMss") & "." & fileNameExtension, FileMode.Create)
streams.Add(stream)
Return stream
End Function
Private Sub Print()
If streams Is Nothing OrElse streams.Count = 0 Then
Return
End If
Dim printDoc As PrintDocument = New PrintDocument()
AddHandler printDoc.PrintPage, AddressOf PrintPage
printDoc.Print()
End Sub
Private Sub PrintPage(ByVal sender As Object, ByVal ev As PrintPageEventArgs)
Dim pageImage As Metafile = New Metafile(streams(pageIndex))
ev.Graphics.DrawImage(pageImage, ev.PageBounds)
pageIndex += 1
ev.HasMorePages = (pageIndex < streams.Count)
End Sub
Private Function GetData(ByVal query As String) As Customers
Dim conString As String = ConfigurationManager.ConnectionStrings("constr").ConnectionString
Dim cmd As SqlCommand = New SqlCommand(query)
Using con As SqlConnection = New SqlConnection(conString)
Using sda As SqlDataAdapter = New SqlDataAdapter()
cmd.Connection = con
sda.SelectCommand = cmd
Using dsCustomers As Customers = New Customers()
sda.Fill(dsCustomers, "DataTable1")
Return dsCustomers
End Using
End Using
End Using
End Function