Check this example. Now please take its reference and correct your code.
HTML
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
<style type="text/css">
body {
font-family: Arial;
font-size: 10pt;
}
</style>
</head>
<body>
<form id="form1" runat="server">
<table border="0" cellpadding="0" cellspacing="0">
<tr>
<td style="width: 80px">To:
</td>
<td>
<asp:TextBox ID="txtTo" runat="server"></asp:TextBox>
</td>
</tr>
<tr>
<td>
</td>
</tr>
<tr>
<td>Subject:
</td>
<td>
<asp:TextBox ID="txtSubject" runat="server"></asp:TextBox>
</td>
</tr>
<tr>
<td>
</td>
</tr>
<tr>
<td valign="top">Body:
</td>
<td>
<asp:TextBox ID="txtBody" runat="server" TextMode="MultiLine" Height="150" Width="200"></asp:TextBox>
</td>
</tr>
<tr>
<td>
</td>
</tr>
<tr>
<td>File Attachment:
</td>
<td>
<asp:FileUpload ID="fuAttachment" runat="server" AllowMultiple ="true" />
</td>
</tr>
<tr>
<td>
</td>
</tr>
<tr>
<td>Gmail Email:
</td>
<td>
<asp:TextBox ID="txtEmail" runat="server"></asp:TextBox>
</td>
</tr>
<tr>
<td>
</td>
</tr>
<tr>
<td>Gmail Password:
</td>
<td>
<asp:TextBox ID="txtPassword" runat="server" TextMode="Password"></asp:TextBox>
</td>
</tr>
<tr>
<td>
</td>
</tr>
<tr>
<td></td>
<td>
<asp:Button Text="Send" OnClick="SendEmail" runat="server" />
</td>
</tr>
</table>
</form>
</body>
</html>
Namespaces
using System.IO;
using System.Net;
using System.Net.Mail;
Code
protected void SendEmail(object sender, EventArgs e)
{
string to = txtTo.Text;
string from = txtEmail.Text;
string subject = txtSubject.Text;
string body = txtBody.Text;
using (MailMessage mm = new MailMessage(txtEmail.Text, txtTo.Text))
{
mm.Subject = txtSubject.Text;
mm.Body = txtBody.Text;
if (fuAttachment.HasFile)
{
foreach (HttpPostedFile file in fuAttachment.PostedFiles)
{
string fileName = Path.GetFileName(file.FileName);
mm.Attachments.Add(new Attachment(file.InputStream, fileName));
}
}
mm.IsBodyHtml = false;
SmtpClient smtp = new SmtpClient();
smtp.Host = "smtp.gmail.com";
smtp.EnableSsl = true;
NetworkCredential NetworkCred = new NetworkCredential(txtEmail.Text, txtPassword.Text);
smtp.UseDefaultCredentials = true;
smtp.Credentials = NetworkCred;
smtp.Port = 587;
smtp.Send(mm);
ClientScript.RegisterStartupScript(GetType(), "alert", "alert('Email sent.');", true);
}
}
Screenshot
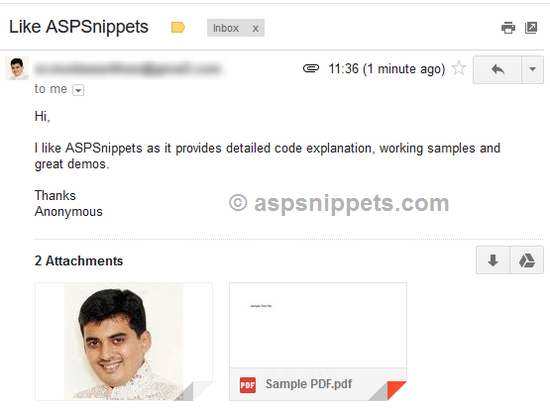