Hi fp2021,
Check this example. Now please take its reference and correct your code.
Database
I have made use of the following table Customers with the schema as follows.
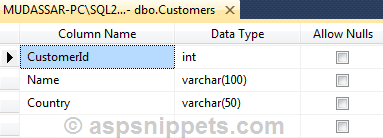
You can download the database table SQL by clicking the download link below.
Download SQL file
Model
public class CustomerModel
{
public int CustomerId { get; set; }
public string Name { get; set; }
public string Country { get; set; }
}
Namespaces
using System.Data.SqlClient;
using System.Net;
using Newtonsoft.Json;
using Microsoft.Extensions.Configuration;
Controller
public class HomeController : Controller
{
private IConfiguration Configuration;
public HomeController(IConfiguration _configuration)
{
Configuration = _configuration;
}
public IActionResult Index()
{
//Fetch the JSON string.
ServicePointManager.Expect100Continue = true;
ServicePointManager.SecurityProtocol = SecurityProtocolType.Tls12;
string json = new WebClient().DownloadString("https://raw.githubusercontent.com/aspsnippets/test/master/Customers.json");
List<CustomerModel> customers = JsonConvert.DeserializeObject<List<CustomerModel>>(json);
foreach (CustomerModel customer in customers)
{
InsertCustomer(customer.CustomerId, customer.Name, customer.Country);
}
return View();
}
private void InsertCustomer(int id, string name, string country)
{
string constr = this.Configuration.GetConnectionString("ConStr");
using (SqlConnection con = new SqlConnection(constr))
{
string query = "INSERT INTO CustomersInf VALUES(@Id,@Name,@Country)";
using (SqlCommand cmd = new SqlCommand(query))
{
cmd.Connection = con;
cmd.Parameters.AddWithValue("@Id", id);
cmd.Parameters.AddWithValue("@Name", name);
cmd.Parameters.AddWithValue("@Country", country);
con.Open();
cmd.ExecuteNonQuery();
con.Close();
}
}
}
}
View
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Index</title>
</head>
<body>
</body>
</html>
Inserted record in database

For more details on reading connection string from appsettings.json refer below article.