Hi rani,
Check this example. Now please take its reference and correct your code.
Database
I have made use of the following table Customers with the schema as follows.
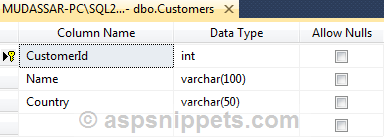
I have already inserted few records in the table.
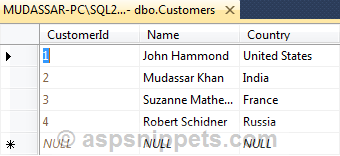
You can download the database table SQL by clicking the download link below.
Download SQL file
Stored Procedure
CREATE PROCEDURE [dbo].[Insert_Customer]
@Name VARCHAR(100) = NULL
,@Country VARCHAR(100) = NULL
,@CustomerId INT OUTPUT
AS
BEGIN
SET NOCOUNT ON;
INSERT INTO Customers(Name, Country)
VALUES (@Name, @Country)
SET @CustomerId = SCOPE_IDENTITY()
SELECT @CustomerId
END
Model
public class Customer
{
public int CustomerId { get; set; }
public string Name { get; set; }
public string Country { get; set; }
}
Namespaces
using System.Collections.Generic;
using System.Data;
using System.Data.SqlClient;
Controller
public class HomeController : Controller
{
public IActionResult Index()
{
ViewBag.Customers = GetCustomers();
return View();
}
[HttpPost]
public IActionResult Index(Customer customer)
{
string constr = @"Server=.;DataBase=Test;UID=sa;PWD=pass@123";
using (SqlConnection con = new SqlConnection(constr))
{
string query = "Insert_Customer";
using (SqlCommand cmd = new SqlCommand(query))
{
cmd.Connection = con;
cmd.CommandType = CommandType.StoredProcedure;
cmd.Parameters.AddWithValue("@Name", customer.Name);
cmd.Parameters.AddWithValue("@Country", customer.Country);
cmd.Parameters.Add("@CustomerId", SqlDbType.Int);
cmd.Parameters["@CustomerId"].Direction = ParameterDirection.Output;
con.Open();
cmd.ExecuteNonQuery();
con.Close();
ViewBag.LastId = cmd.Parameters["@CustomerId"].Value.ToString();
con.Close();
}
}
ViewBag.Customers = GetCustomers();
return View();
}
private List<Customer> GetCustomers()
{
List<Customer> customers = new List<Customer>();
string conString = @"Server=.;DataBase=Test;UID=sa;PWD=pass@123";
using (SqlConnection con = new SqlConnection(conString))
{
SqlCommand cmd = new SqlCommand("SELECT * FROM Customers", con);
con.Open();
SqlDataReader sdr = cmd.ExecuteReader();
while (sdr.Read())
{
customers.Add(new Customer
{
CustomerId = Convert.ToInt32(sdr["CustomerId"]),
Name = sdr["Name"].ToString(),
Country = sdr["Country"].ToString()
});
}
con.Close();
}
return customers;
}
}
View
@addTagHelper*, Microsoft.AspNetCore.Mvc.TagHelpers
@using Last_Id_Procedure_Core_MVC.Models
@model Customer
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Index</title>
</head>
<body>
<form asp-action="Index" asp-controller="Home" method="post">
Name: <input type="text" asp-for="@Model.Name" />
<br /><br />
Country: <input type="text" asp-for="@Model.Country" />
<br /><br />
<input type="submit" id="btnSubmit" value="Submit" />
</form>
@if (ViewBag.Customers != null)
{
<hr />
<table>
<thead>
<tr>
<th>Id</th>
<th>Name</th>
<th>Country</th>
</tr>
</thead>
<tbody>
@foreach (Customer customer in ViewBag.Customers)
{
<tr>
<td>@customer.CustomerId</td>
<td>@customer.Name</td>
<td>@customer.Country</td>
</tr>
}
</tbody>
</table>
}
@if (ViewBag.LastId != null)
{
<script type="text/javascript">
window.onload = function () {
alert('Last Inserted Id : '+'@ViewBag.LastId');
};
</script>
}
</body>
</html>
Screenshot
