Hi ramco1917,
Please refer below sample.
Database
I have made use of the following table Customers with the schema as follows.
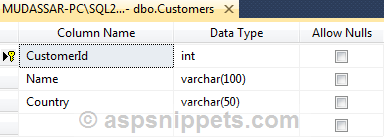
I have already inserted few records in the table.
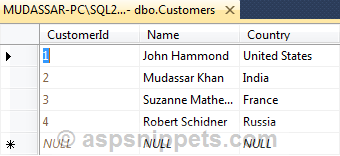
You can download the database table SQL by clicking the download link below.
Download SQL file
HTML
<form id="form1" runat="server">
<asp:GridView ID="gvCustomers" runat="server" AutoGenerateColumns="false">
<Columns>
<asp:BoundField DataField="Name" HeaderText="Name" />
<asp:TemplateField HeaderText="Country">
<ItemTemplate>
<span onmouseover="showInfo('<%# Eval("CustomerId") %>')">Info</span>
</ItemTemplate>
</asp:TemplateField>
</Columns>
</asp:GridView>
<div id="infoPopup" style="display: none;"></div>
</form>
<script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/jquery/1.8.3/jquery.min.js"></script>
<script type="text/javascript">
function showInfo(CustomerId) {
$.ajax({
type: "POST",
url: "CS.aspx/GetUserInfo",
data: '{CustomerId: "' + CustomerId + '"}',
contentType: "application/json; charset=utf-8",
dataType: "json",
success: function (response) {
$("#infoPopup").html(response.d);
$("#infoPopup").show();
}
});
}
$(document).ready(function () {
$("#infoPopup").mouseleave(function () {
$(this).hide();
});
});
</script>
Namespaces
C#
using System.Web.Services;
using System.Configuration;
using System.Data.SqlClient;
VB.Net
Imports System.Web.Services
Imports System.Configuration
Imports System.Data.SqlClient
Code
C#
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
this.BindData();
}
}
//Bind Data GridView
private void BindData()
{
string constring = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
using (SqlConnection con = new SqlConnection(constring))
{
using (SqlCommand cmd = new SqlCommand("SELECT CustomerId, Name FROM Customers", con))
{
con.Open();
using (SqlDataReader reader = cmd.ExecuteReader())
{
gvCustomers.DataSource = reader;
gvCustomers.DataBind();
}
}
}
}
//GetUsersInfo from BindData
[WebMethod]
public static string GetUserInfo(string CustomerId)
{
string constring = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
string country = "";
using (SqlConnection con = new SqlConnection(constring))
{
using (SqlCommand cmd = new SqlCommand("SELECT Country FROM Customers WHERE CustomerId=@CustomerId", con))
{
cmd.Parameters.AddWithValue("@CustomerId", CustomerId);
con.Open();
using (SqlDataReader reader = cmd.ExecuteReader())
{
if (reader.Read())
{
country = reader["Country"].ToString();
}
}
}
}
//Hover Message
string infoHtml = string.Format("<p>Country:{0}</p>", country);
return infoHtml;
}
VB.Net
Inherits System.Web.UI.Page
Protected Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs) Handles Me.Load
If Not IsPostBack Then
Me.BindData()
End If
End Sub
'Bind Data GridView
Private Sub BindData()
Dim constring As String = ConfigurationManager.ConnectionStrings("constr").ConnectionString
Using con As SqlConnection = New SqlConnection(constring)
Using cmd As SqlCommand = New SqlCommand("SELECT CustomerId, Name FROM Customers", con)
con.Open()
Using reader As SqlDataReader = cmd.ExecuteReader()
gvCustomers.DataSource = reader
gvCustomers.DataBind()
End Using
End Using
End Using
End Sub
'GetUsersInfo from BindData
<WebMethod>
Public Shared Function GetUserInfo(ByVal CustomerId As String) As String
Dim constring As String = ConfigurationManager.ConnectionStrings("constr").ConnectionString
Dim country As String = ""
Using con As SqlConnection = New SqlConnection(constring)
Using cmd As SqlCommand = New SqlCommand("SELECT Country FROM Customers WHERE CustomerId=@CustomerId", con)
cmd.Parameters.AddWithValue("@CustomerId", CustomerId)
con.Open()
Using reader As SqlDataReader = cmd.ExecuteReader()
If reader.Read() Then
country = reader("Country").ToString()
End If
End Using
End Using
End Using
'Hover Message
Dim infoHtml As String = String.Format("<p>Country:{0}</p>", country)
Return infoHtml
End Function
Screenshot
