Hi BilalKhan1,
Check this example. Now please take its reference and correct your code.
Database
For this example I have used of Northwind database that you can download using the link given below.
Download Northwind Database
For custom paging refer below article.
Stored Procedure
--EXEC GetCustomersPageWise 2,10,94
CREATE PROCEDURE [dbo].[GetCustomersPageWise]
@PageIndex INT = 1
,@PageSize INT = 10
,@PageCount INT OUTPUT
AS
BEGIN
SET NOCOUNT ON;
SELECT ROW_NUMBER() OVER (ORDER BY [CustomerID] ASC) AS RowNumber
,[CustomerID]
,[CompanyName]
,[ContactName]
,[City]
,[Country]
,[PostalCode]
,[Phone]
,[Fax]
INTO #Results
FROM [Customers]
SELECT @PageCount = COUNT(*) FROM #Results
SELECT * FROM #Results
WHERE RowNumber BETWEEN(@PageIndex -1) * @PageSize + 1 AND(((@PageIndex -1) * @PageSize + 1) + @PageSize) - 1
DROP TABLE #Results
END
HTML
<table class="Grid" cellspacing="0" rules="all" border="1" style="width: 500px; border-collapse: collapse;">
<tr>
<th scope="col" style="width: 200px;">Customer Name</th>
<th scope="col" style="width: 100px;">City</th>
<th scope="col" style="width: 100px;">Country</th>
<th scope="col" style="width: 100px;">Postal Code</th>
</tr>
</table>
<div id="dvGrid" style="height: 250px; overflow: auto; width: 517px">
<asp:GridView ID="gvCustomers" runat="server" AutoGenerateColumns="false" CssClass="Grid" Width="500">
<Columns>
<asp:BoundField DataField="ContactName" HeaderText="Customer Name" ItemStyle-CssClass="name" ItemStyle-Width="200" HeaderStyle-Width="200" />
<asp:BoundField DataField="City" HeaderText="City" ItemStyle-CssClass="city" ItemStyle-Width="100" HeaderStyle-Width="100" />
<asp:BoundField DataField="Country" HeaderText="Country" ItemStyle-CssClass="country" ItemStyle-Width="100" HeaderStyle-Width="100" />
<asp:BoundField DataField="PostalCode" HeaderText="Postal Code" ItemStyle-CssClass="postal" ItemStyle-Width="100" HeaderStyle-Width="100" />
</Columns>
</asp:GridView>
</div>
<script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/jquery/1.8.3/jquery.min.js"></script>
<script type="text/javascript">
var pageIndex = 1;
var pageCount;
$(function () {
//Remove the original GridView header
$("[id$=gvCustomers] tr").eq(0).remove();
});
//Load GridView Rows when DIV is scrolled
$("#dvGrid").on("scroll", function (e) {
var $o = $(e.currentTarget);
if ($o[0].scrollHeight - $o.scrollTop() <= $o.outerHeight()) {
GetRecords();
}
});
//Function to make AJAX call to the Web Method
function GetRecords() {
pageIndex++;
if (pageIndex == 2 || pageIndex <= pageCount) {
//Show Loader
if ($("[id$=gvCustomers] .loader").length == 0) {
var row = $("[id$=gvCustomers] tr").eq(0).clone(true);
row.addClass("loader");
row.children().remove();
row.append('<td colspan = "999" style = "background-color:white"><img id="loader" alt="" src="103.gif" /></td>');
$("[id$=gvCustomers]").append(row);
}
$.ajax({
type: "POST",
url: "Default.aspx/GetCustomers",
data: '{pageIndex: ' + pageIndex + '}',
contentType: "application/json; charset=utf-8",
dataType: "json",
success: OnSuccess,
failure: function (response) {
alert(response.d);
},
error: function (response) {
alert(response.d);
}
});
}
}
function OnSuccess(response) {
var json = JSON.parse(response.d);
pageCount = parseInt(json.RecordCount);
var customers = json.Customers;
$("[id$=gvCustomers] .loader").remove();
$.each(customers, function () {
var customer = $(this);
var row = $("[id$=gvCustomers] tr").eq(0).clone(true);
$(".name", row).html(customer[0].ContactName);
$(".city", row).html(customer[0].City);
$(".postal", row).html(customer[0].PostalCode);
$(".country", row).html(customer[0].Country);
$("[id$=gvCustomers]").append(row);
});
//Hide Loader
$("#loader").hide();
}
</script>
Namespaces
C#
using System.Web.Services;
using System.Data.Entity.Core.Objects;
using System.Web.Script.Serialization;
VB.Net
Imports System.Web.Services
Imports System.Data.Entity.Core.Objects
Imports System.Web.Script.Serialization
Code
C#
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
NorthwindEntities entity = new NorthwindEntities();
gvCustomers.DataSource = entity.Customers.OrderBy(x => x.CustomerID).Take(10).ToList();
gvCustomers.DataBind();
}
}
public static string GetCustomersPageWise(int pageIndex, int pageSize)
{
NorthwindEntities entities = new NorthwindEntities();
CustomerModel model = new CustomerModel();
model.PageIndex = pageIndex;
model.PageSize = pageSize;
ObjectParameter recordCount = new ObjectParameter("PageCount", typeof(int));
var customers = entities.GetCustomers(model.PageIndex, model.PageSize, recordCount).ToList();
model.Customers = customers;
model.RecordCount = Convert.ToInt32(recordCount.Value);
JavaScriptSerializer se = new JavaScriptSerializer();
return se.Serialize(model);
}
[WebMethod]
public static string GetCustomers(int pageIndex)
{
System.Threading.Thread.Sleep(2000);
return GetCustomersPageWise(pageIndex, 10);
}
public class CustomerModel
{
public List<Customer> Customers { get; set; }
public int PageIndex { get; set; }
public int PageSize { get; set; }
public int RecordCount { get; set; }
}
VB.Net
Protected Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs) Handles Me.Load
If Not IsPostBack Then
Dim entity As NorthwindEntities = New NorthwindEntities()
gvCustomers.DataSource = entity.Customers.OrderBy(Function(x) x.CustomerID).Take(10).ToList()
gvCustomers.DataBind()
End If
End Sub
Public Shared Function GetCustomersPageWise(ByVal pageIndex As Integer, ByVal pageSize As Integer) As String
Dim entities As NorthwindEntities = New NorthwindEntities()
Dim model As CustomerModel = New CustomerModel()
model.PageIndex = pageIndex
model.PageSize = pageSize
Dim recordCount As ObjectParameter = New ObjectParameter("PageCount", GetType(Integer))
Dim customers = entities.GetCustomers(model.PageIndex, model.PageSize, recordCount).ToList()
model.Customers = customers
model.RecordCount = Convert.ToInt32(recordCount.Value)
Dim se As JavaScriptSerializer = New JavaScriptSerializer()
Return se.Serialize(model)
End Function
<WebMethod>
Public Shared Function GetCustomers(ByVal pageIndex As Integer) As String
System.Threading.Thread.Sleep(2000)
Return GetCustomersPageWise(pageIndex, 10)
End Function
Public Class CustomerModel
Public Property Customers As List(Of Customer)
Public Property PageIndex As Integer
Public Property PageSize As Integer
Public Property RecordCount As Integer
End Class
Screenshot
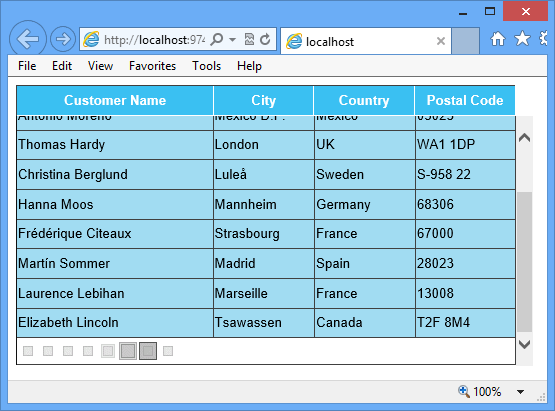