Hi makumbi,
Please refer below sample.
Database
I have made use of the following table Customers with the schema as follows.
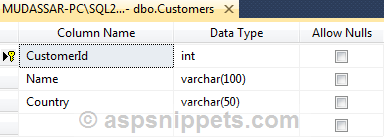
I have already inserted few records in the table.
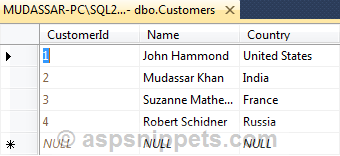
You can download the database table SQL by clicking the download link below.
Download SQL file
HTML
<form id="form1" runat="server">
<table style="border-collapse: collapse;" cellspacing="0" border="1">
<tr>
<td width="150">Name</td>
<td width="150">
<asp:TextBox ID="txtName" runat="server"></asp:TextBox>
</td>
</tr>
<tr>
<td width="150">Country</td>
<td width="150">
<asp:TextBox ID="txtCountry" runat="server"></asp:TextBox>
</td>
</tr>
<tr>
<td>
<asp:Button ID="btnAdd" runat="server" Text="Add" />
</td>
</tr>
</table>
<table class="tblCustomers" style="border-collapse: collapse;" cellspacing="0" border="1">
<thead>
<tr>
<th width="150">Id</th>
<th width="150">Name</th>
<th width="150">Country</th>
</tr>
</thead>
<tbody>
</tbody>
</table>
<script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/jquery/1.8.3/jquery.min.js"></script>
<script type="text/javascript" src="Scripts/jquery.signalR-2.2.2.min.js"></script>
<script type="text/javascript" src="signalr/hubs"></script>
<script type="text/javascript">
$(function () {
$.connection.hub.start().done(function () {
setInterval(GetData, 1000);
}).fail(function (e) {
alert(e);
});
$('#btnAdd').on('click', function () {
var customer = {};
customer.Name = $('#txtName').val();
customer.Country = $('#txtCountry').val();
$.ajax({
type: "POST",
url: "WebService.asmx/InsertCustomer",
data: JSON.stringify({ customer: customer }),
contentType: "application/json; charset=utf-8",
dataType: "json",
success: function (response) {
},
failure: function (response) {
alert(response.responseText);
},
error: function (response) {
alert(response.responseText);
}
});
return false;
});
});
function GetData() {
$.ajax({
type: "POST",
url: "WebService.asmx/GetCustomers",
data: '{}',
contentType: "application/json; charset=utf-8",
dataType: "json",
async: true,
success: function (response) {
var rows = '';
$(response.d).each(function () {
rows += "<tr><td>" + this.Id + "</td><td>" + this.Name + "</td><td>" + this.Country + "</td></tr>";
});
$('.tblCustomers tbody').empty();
$('.tblCustomers tbody').append(rows);
},
failure: function (response) {
alert(response);
},
error: function (response) {
alert(response);
}
});
}
</script>
</form>
Namespaces
C#
using System.Collections.Generic;
using System.Configuration;
using System.Data.SqlClient;
using System.Web.Services;
VB.Net
Imports System.Collections.Generic
Imports System.Configuration
Imports System.Data.SqlClient
Imports System.Web.Services
WebService
C#
[WebService(Namespace = "http://tempuri.org/")]
[WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)]
[System.ComponentModel.ToolboxItem(false)]
// To allow this Web Service to be called from script, using ASP.NET AJAX, uncomment the following line.
[System.Web.Script.Services.ScriptService]
public class WebService : System.Web.Services.WebService
{
[WebMethod]
public List<Customer> GetCustomers()
{
string constr = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
using (SqlConnection con = new SqlConnection(constr))
{
using (SqlCommand cmd = new SqlCommand("SELECT CustomerId,Name,Country FROM Customers"))
{
cmd.Connection = con;
List<Customer> Customers = new List<Customer>();
con.Open();
using (SqlDataReader sdr = cmd.ExecuteReader())
{
while (sdr.Read())
{
Customers.Add(new Customer()
{
Id = sdr["CustomerId"].ToString(),
Name = sdr["Name"].ToString(),
Country = sdr["Country"].ToString()
});
}
}
con.Close();
return Customers;
}
}
}
[WebMethod]
public void InsertCustomer(Customer customer)
{
string conString = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
using (SqlConnection con = new SqlConnection(conString))
{
using (SqlCommand cmd = new SqlCommand("INSERT INTO Customers VALUES (@Name, @Country)", con))
{
cmd.Parameters.AddWithValue("@Name", customer.Name);
cmd.Parameters.AddWithValue("@Country", customer.Country);
con.Open();
cmd.ExecuteNonQuery();
con.Close();
}
}
}
public class Customer
{
public string Id { get; set; }
public string Name { get; set; }
public string Country { get; set; }
}
}
VB.Net
<WebService([Namespace]:="http://tempuri.org/")>
<WebServiceBinding(ConformsTo:=WsiProfiles.BasicProfile1_1)>
<System.ComponentModel.ToolboxItem(False)>
<System.Web.Script.Services.ScriptService>
Public Class WebService
Inherits System.Web.Services.WebService
<WebMethod>
Public Function GetCustomers() As List(Of Customer)
Dim constr As String = ConfigurationManager.ConnectionStrings("constr").ConnectionString
Using con As SqlConnection = New SqlConnection(constr)
Using cmd As SqlCommand = New SqlCommand("SELECT CustomerId,Name,Country FROM Customers")
cmd.Connection = con
Dim Customers As List(Of Customer) = New List(Of Customer)()
con.Open()
Using sdr As SqlDataReader = cmd.ExecuteReader()
While sdr.Read()
Customers.Add(New Customer() With {
.Id = sdr("CustomerId").ToString(),
.Name = sdr("Name").ToString(),
.Country = sdr("Country").ToString()
})
End While
End Using
con.Close()
Return Customers
End Using
End Using
End Function
<WebMethod>
Public Sub InsertCustomer(ByVal customer As Customer)
Dim conString As String = ConfigurationManager.ConnectionStrings("constr").ConnectionString
Using con As SqlConnection = New SqlConnection(conString)
Using cmd As SqlCommand = New SqlCommand("INSERT INTO Customers VALUES (@Name, @Country)", con)
cmd.Parameters.AddWithValue("@Name", customer.Name)
cmd.Parameters.AddWithValue("@Country", customer.Country)
con.Open()
cmd.ExecuteNonQuery()
con.Close()
End Using
End Using
End Sub
Public Class Customer
Public Property Id As String
Public Property Name As String
Public Property Country As String
End Class
End Class
Screenshot
