Hi RichardSa,
In the page where you want to display the word, make Ajax call to WebMethod and fetch the details from database based on the Session value.
Then conver the binary data to base64 array and pass to the Ajax Success function.
Then convert Base64 string to Byte Array and Byte Array to File object and finally, display the Word Document using the JavaScript plugin.
Please refer the sample.
Database
Here i am making use of table tblFiles whose schema is defined as below.
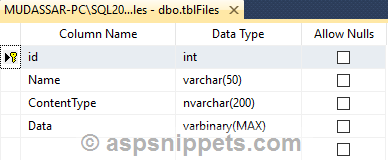
I have inserted few records in the table.

HTML
Default
<asp:Button Text="Send" ID="btnSend" runat="server" OnClick="Send" />
Home
<div id="word-container"></div>
<script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/jquery/1.8.3/jquery.min.js"></script>
<script type="text/javascript" src="https://unpkg.com/jszip/dist/jszip.min.js"></script>
<script type="text/javascript" src="https://volodymyrbaydalka.github.io/docxjs/dist/docx-preview.js"></script>
<script type="text/javascript">
$(function () {
$.ajax({
type: "POST",
url: "Default.aspx/GetFile",
data: "{}",
contentType: "application/json; charset=utf-8",
dataType: "json",
success: function (response) {
var file = response.d;
PreviewWordDoc(file);
},
failure: function (response) {
alert(response.responseText);
},
error: function (response) {
alert(response.responseText);
}
});
});
function Base64ToBytes(base64) {
var s = window.atob(base64);
var bytes = new Uint8Array(s.length);
for (var i = 0; i < s.length; i++) {
bytes[i] = s.charCodeAt(i);
}
return bytes;
};
function PreviewWordDoc(file) {
//Convert Base64 to Byte Array.
var bytes = Base64ToBytes(file.Data);
//Convert Byte Array to File object.
var doc = new File([bytes], file.Name);
//Set the Document options.
var docxOptions = Object.assign(docx.defaultOptions, {
useMathMLPolyfill: true
});
//Reference the Container DIV.
var container = document.querySelector("#word-container");
//Render the Word Document.
docx.renderAsync(doc, container, null, docxOptions);
}
</script>
Namespces
C#
using System.Data.SqlClient;
using System.Configuration;
using System.Web.Services;
VB.Net
Imports System.Data.SqlClient
Imports System.Configuration
Imports System.Web.Services
Code
Default
C#
protected void Send(object sender, EventArgs e)
{
Session["FileId"] = "9";
Response.Redirect("Home.aspx");
}
VB.Net
Protected Sub Send(ByVal sender As Object, ByVal e As EventArgs)
Session("FileId") = "9"
Response.Redirect("Home.aspx")
End Sub
Home
C#
[WebMethod(EnableSession = true)]
public static object GetFile()
{
string fileName = string.Empty;
byte[] bytes = null;
string type = string.Empty;
string conString = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
using (SqlConnection con = new SqlConnection(conString))
{
using (SqlCommand cmd = new SqlCommand("SELECT * FROM tblFiles WHERE Id = @Id", con))
{
cmd.Parameters.AddWithValue("@Id", HttpContext.Current.Session["FileId"]);
con.Open();
using (SqlDataReader sdr = cmd.ExecuteReader())
{
if (sdr.Read())
{
fileName = sdr["Name"].ToString();
bytes = (byte[])sdr["Data"];
type = sdr["ContentType"].ToString();
}
}
con.Close();
}
}
return new { Name = fileName, Data = Convert.ToBase64String(bytes), ContentType = type };
}
VB.Net
<WebMethod(EnableSession:=True)>
Public Shared Function GetFile() As Object
Dim fileName As String = String.Empty
Dim bytes As Byte() = Nothing
Dim type As String = String.Empty
Dim conString As String = ConfigurationManager.ConnectionStrings("constr").ConnectionString
Using con As SqlConnection = New SqlConnection(conString)
Using cmd As SqlCommand = New SqlCommand("SELECT * FROM tblFiles WHERE Id = @Id", con)
cmd.Parameters.AddWithValue("@Id", HttpContext.Current.Session("FileId"))
con.Open()
Using sdr As SqlDataReader = cmd.ExecuteReader()
If sdr.Read() Then
fileName = sdr("Name").ToString()
bytes = CType(sdr("Data"), Byte())
type = sdr("ContentType").ToString()
End If
End Using
con.Close()
End Using
End Using
Return New With {Key _
.Name = fileName, Key _
.Data = Convert.ToBase64String(bytes), Key _
.ContentType = type
}
End Function
Screenshot
