Hi guhananth,
Check this example. Now please take its reference and correct your code.
Database
I have made use of the following tables with the schema as follows.
VehicleTypes
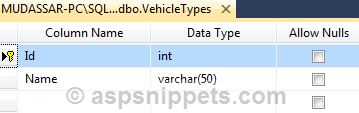
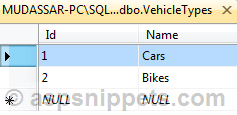
VehicleSubTypes
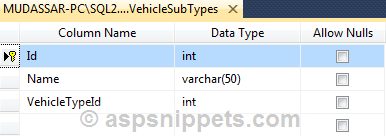
You can download the database table SQL by clicking the download link below.
Download SQL file
HTML
<input type="text" id="txtType" />
<div id="jstree"></div>
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/jstree/3.2.1/themes/default/style.min.css" />
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/jquery/1.12.1/jquery.min.js"></script>
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/jstree/3.2.1/jstree.min.js"></script>
<script type="text/javascript">
$(function () {
BindJsTree('');
$('#txtType').on('change', function () {
BindJsTree($(this).val());
});
});
function BindJsTree(typeVehicle) {
$.ajax({
type: "POST",
url: "Default.aspx/GetData",
data: "{vehicleType:'" + typeVehicle + "'}",
contentType: "application/json; charset=utf-8",
dataType: "json",
success: function (response) {
var json = JSON.parse(response.d);
$("#jstree").jstree("destroy");
$('#jstree').jstree({
'core': {
'data': json,
'themes': { "variant": "large" },
},
'checkbox': { "keep_selected_style": false },
'plugins': ["wholerow", "checkbox"]
});
},
error: function (response) {
alert(response.responseText);
}
});
}
</script>
Namespaces
C#
using System.Web.Script.Serialization;
using System.Web.Services;
VB.Net
Imports System.Web.Script.Serialization
Imports System.Web.Services
Code
C#
[WebMethod]
public static string GetData(string vehicleType)
{
VehiclesDBEntities entities = new VehiclesDBEntities();
List<VehicleType> vehicleTypes = entities.VehicleTypes.ToList();
List<VehicleSubType> vehicleSubTypes = new List<VehicleSubType>();
if (!string.IsNullOrEmpty(vehicleType.Trim()))
{
vehicleTypes = vehicleTypes.Where(x => x.Name.ToLower() == vehicleType.Trim().ToLower()).ToList();
}
List<TreeViewNode> nodes = new List<TreeViewNode>();
foreach (VehicleType type in vehicleTypes)
{
nodes.Add(new TreeViewNode
{
id = type.Id.ToString(),
parent = "#",
text = type.Name
});
vehicleSubTypes.AddRange(entities.VehicleSubTypes.Where(x => x.VehicleTypeId == type.Id).ToList());
}
foreach (VehicleSubType subType in vehicleSubTypes)
{
nodes.Add(new TreeViewNode
{
id = subType.VehicleTypeId.ToString() + "-" + subType.Id.ToString(),
parent = subType.VehicleTypeId.ToString(),
text = subType.Name
});
}
return (new JavaScriptSerializer()).Serialize(nodes);
}
public class TreeViewNode
{
public string id { get; set; }
public string parent { get; set; }
public string text { get; set; }
}
VB.Net
<WebMethod>
Public Shared Function GetData(ByVal vehicleType As String) As String
Dim entities As VehiclesDBEntities = New VehiclesDBEntities()
Dim vehicleTypes As List(Of VehicleType) = entities.VehicleTypes.ToList()
Dim vehicleSubTypes As List(Of VehicleSubType) = New List(Of VehicleSubType)()
If Not String.IsNullOrEmpty(vehicleType.Trim()) Then
vehicleTypes = vehicleTypes.Where(Function(x) x.Name.ToLower() = vehicleType.Trim().ToLower()).ToList()
End If
Dim nodes As List(Of TreeViewNode) = New List(Of TreeViewNode)()
For Each type As VehicleType In vehicleTypes
nodes.Add(New TreeViewNode With {
.id = type.Id.ToString(),
.parent = "#",
.text = type.Name
})
vehicleSubTypes.AddRange(entities.VehicleSubTypes.Where(Function(x) x.VehicleTypeId = type.Id).ToList())
Next
For Each subType As VehicleSubType In vehicleSubTypes
nodes.Add(New TreeViewNode With {
.id = subType.VehicleTypeId.ToString() & "-" + subType.Id.ToString(),
.parent = subType.VehicleTypeId.ToString(),
.text = subType.Name
})
Next
Return (New JavaScriptSerializer()).Serialize(nodes)
End Function
Public Class TreeViewNode
Public Property id As String
Public Property parent As String
Public Property text As String
End Class
Screenshot
