Hi merix,
Using the Leaflet library i have created the sample.
Download Leaflet Library
Database
I have made use of the following table Locations with the schema as follows.
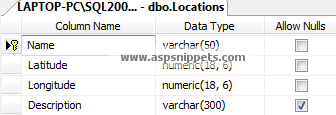
I have already inserted few records in the table.
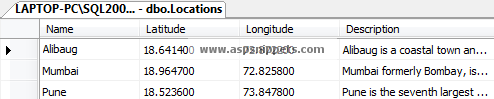
You can download the database table SQL by clicking the download link below.
Download SQL file
Model
public class MapModel
{
public string Name { get; set; }
public decimal Latitude { get; set; }
public decimal Longitude { get; set; }
public string Description { get; set; }
}
Namespaces
using System.Collections.Generic;
using System.Configuration;
using System.Data.SqlClient;
Controller
public class HomeController : Controller
{
// GET: Home
public ActionResult Index()
{
return View();
}
public JsonResult GetData()
{
List<MapModel> mapData = new List<MapModel>();
string conString = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
using (SqlConnection con = new SqlConnection(conString))
{
using (SqlCommand cmd = new SqlCommand("SELECT * FROM Locations", con))
{
con.Open();
using (SqlDataReader sdr = cmd.ExecuteReader())
{
while (sdr.Read())
{
mapData.Add(new MapModel
{
Name = sdr["Name"].ToString(),
Latitude = Convert.ToDecimal(sdr["Latitude"]),
Longitude = Convert.ToDecimal(sdr["Longitude"]),
Description = sdr["Description"].ToString()
});
}
}
con.Close();
}
}
return Json(mapData, JsonRequestBehavior.AllowGet);
}
}
View
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Index</title>
<link rel="stylesheet" href="https://unpkg.com/leaflet@1.9.4/dist/leaflet.css"
integrity="sha512-xwE/Az9zrjBIphAcBb3F6JVqxf46+CDLwfLMHloNu6KEQCAWi6HcDUbeOfBIptF7tcCzusKFjFw2yuvEpDL9wQ==" crossorigin="" />
<script type="text/javascript" src="https://unpkg.com/leaflet@1.9.4/dist/leaflet.js"
integrity="sha512-gZwIG9x3wUXg2hdXF6+rVkLF/0Vi9U8D2Ntg4Ga5I5BZpVkVxlJWbSQtXPSiUTtC0TjtGOmxa1AJPuV0CPthew==" crossorigin=""></script>
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.7.1/jquery.min.js"></script>
</head>
<body>
<div id="map" style="width: 400px; height: 400px;"></div>
<script type="text/javascript">
$(function () {
$.ajax({
type: "GET",
url: '/Home/GetData',
data: {},
contentType: "application/json; charset=utf-8",
dataType: "json",
success: function (data) {
// Initializing the Map.
var map = L.map('map').setView([data[0].Latitude, data[0].Longitude], 8);
// Setting the Attribution.
L.tileLayer('https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png', {
attribution: '© <a href="https://www.openstreetmap.org/copyright">OpenStreetMap</a> contributors'
}).addTo(map);
// Adding Marker to map.
data.forEach(function (index, item) {
L.marker([item.Latitude, item.Longitude])
.bindPopup("<b>" + item.Name + "</b><br />" + item.Description).addTo(map);
});
}, error: function (response) {
alert(response.responseText);
}
});
});
</script>
</body>
</html>
Screenshot
