Hi maduri0905,
Refer below sample.
I have made use of the following table Users with the schema as follows.
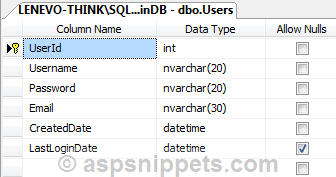
Model
public class UserModel
{
[Key]
public int UserId { get; set; }
[Required(ErrorMessage = "Required.")]
public string Username { get; set; }
[Required(ErrorMessage = "Required.")]
public string Password { get; set; }
[NotMapped]
[Required(ErrorMessage = "Required.")]
[Compare("Password", ErrorMessage = "Passwords do not match.")]
public string ConfirmPassword { get; set; }
[Required(ErrorMessage = "Required.")]
[EmailAddress(ErrorMessage = "Invalid email address.")]
public string Email { get; set; }
public System.DateTime CreatedDate { get; set; }
public Nullable<System.DateTime> LastLoginDate { get; set; }
}
Database Context
using Microsoft.EntityFrameworkCore;
namespace Registration_Core_MVC
{
public class DBCtx : DbContext
{
public DBCtx(DbContextOptions<DBCtx> options) : base(options)
{
}
public DbSet<UserModel> Users { get; set; }
}
}
Controller
public class HomeController : Controller
{
private DBCtx Context { get; set; }
public HomeController(DBCtx _context)
{
this.Context = _context;
}
public IActionResult Index()
{
return View();
}
[HttpPost]
public ActionResult Index(UserModel user)
{
user.CreatedDate = DateTime.Now;
this.Context.Users.Add(user);
this.Context.SaveChanges();
string message = string.Empty;
switch (user.UserId)
{
case -1:
message = "Username already exists.\\nPlease choose a different username.";
break;
case -2:
message = "Supplied email address has already been used.";
break;
default:
message = "Registration successful.\\nUser Id: " + user.UserId.ToString();
break;
}
ViewBag.Message = message;
return View(user);
}
}
View
@addTagHelper*, Microsoft.AspNetCore.Mvc.TagHelpers
@model Registration_Core_MVC.Models.UserModel
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Index</title>
</head>
<body>
<form method="post" asp-controller="Home" asp-action="Index">
<table border="0" cellpadding="0" cellspacing="0">
<tr>
<th colspan="3">
Registration
</th>
</tr>
<tr>
<td>
Username
</td>
<td>
<input type="text" asp-for="Username" />
</td>
<td>
<span asp-validation-for="Username"></span>
</td>
</tr>
<tr>
<td>
Password
</td>
<td>
<input type="password" asp-for="Password" />
</td>
<td>
<span asp-validation-for="Password"></span>
</td>
</tr>
<tr>
<td>
Confirm Password
</td>
<td>
<input type="password" asp-for="ConfirmPassword" />
</td>
<td>
<span asp-validation-for="ConfirmPassword"></span>
</td>
</tr>
<tr>
<td>
Email
</td>
<td>
<input type="text" asp-for="Email" />
</td>
<td>
<span asp-validation-for="Email"></span>
</td>
</tr>
<tr>
<td></td>
<td>
<input type="submit" value="Submit" />
</td>
<td></td>
</tr>
</table>
</form>
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.0/jquery.js"></script>
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/jquery-validate/1.19.3/jquery.validate.js"></script>
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/jquery-validation-unobtrusive/3.2.12/jquery.validate.unobtrusive.js"></script>
@if (@ViewBag.Message != null)
{
<script type="text/javascript">
$(function () {
alert("@ViewBag.Message")
});
</script>
}
</body>
</html>
Screenshot
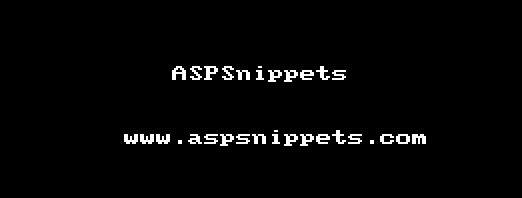