Hi amar,
Check this example. Now please take its reference and correct your code.
Database
I have made use of the following table Hobbies with the schema as follows.
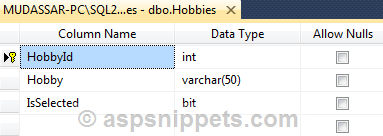
I have already inserted few records in the table.
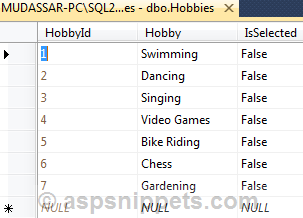
You can download the database table SQL by clicking the download link below.
Download SQL file
I have set the Singing IsSelected value as True.
Model
public class HobbyModel
{
public HobbyModel()
{
this.Hobbies = new List<SelectListItem>();
}
public List<SelectListItem> Hobbies { get; set; }
}
Controller
public class HomeController : Controller
{
// GET: Home
public ActionResult Index()
{
return View();
}
[HttpPost]
public JsonResult AjaxMethod()
{
HobbyEntities entities = new HobbyEntities();
HobbyModel model = new HobbyModel();
foreach (Hobby hobby in entities.Hobbies.ToList())
{
model.Hobbies.Add(new SelectListItem
{
Text = hobby.Description,
Value = hobby.HobbyId.ToString(),
Selected = hobby.IsSelected
});
}
return Json(model);
}
}
View
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Index</title>
</head>
<body>
<div id="dvHobbies"></div>
<script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/jquery/1.8.3/jquery.min.js"></script>
<script type="text/javascript">
$(function () {
$.ajax({
type: "POST",
url: "/Home/AjaxMethod",
data: '{}',
contentType: "application/json; charset=utf-8",
dataType: "json",
success: function (response) {
var dvHobbies = $("#dvHobbies");
// Binding RadioButtons.
$.each(response.Hobbies, function () {
if (!this['Selected']) {
dvHobbies.append(
"<input class='radio' type='radio' value='" + this['Value'] + "' />" +
"<label for='" + this['Text'] + "'>" + this['Text'] + "</label><br />");
} else {
dvHobbies.append(
"<input class='radio' type='radio' value='" + this['Value'] + "' checked='checked' />" +
"<label for='" + this['Text'] + "'>" + this['Text'] + "</label><br />");
}
});
},
error: function (response) {
alert(response.responseText);
}
});
});
</script>
</body>
</html>
Screenshot
