Hi ramco1917,
Please refer below sample.
Database
I have made use of the following table Customers with the schema as follows.
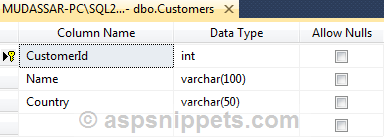
I have already inserted few records in the table.
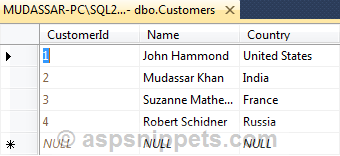
You can download the database table SQL by clicking the download link below.
Download SQL file
HTML
<asp:Repeater ID="rptCustomers" runat="server">
<HeaderTemplate>
<table>
<tr>
<th>CustomerId</th>
<th>Name</th>
<th>Country</th>
</tr>
</HeaderTemplate>
<ItemTemplate>
<tr>
<td>
<asp:Literal ID="litCustomerId" runat="server" Text='<%# Eval("CustomerId") %>'></asp:Literal>
</td>
<td>
<asp:Literal ID="litName" runat="server" Text='<%# Eval("Name") %>'></asp:Literal>
</td>
<td>
<asp:TextBox ID="txtCountry" runat="server" Text='<%# Eval("Country") %>' Visible="false"></asp:TextBox>
<asp:Literal ID="litCountry" runat="server" Text='<%# Eval("Country") %>'></asp:Literal>
</td>
</tr>
</ItemTemplate>
<FooterTemplate>
</table>
</FooterTemplate>
</asp:Repeater>
<asp:Button ID="btnEdit" runat="server" Text="Edit" OnClick="Edit" />
Namespaces
C#
using System.Data;
using System.Data.SqlClient;
using System.Configuration;
VB.Net
Imports System.Data
Imports System.Data.SqlClient
Imports System.Configuration
Code
C#
protected void Page_Load(object sender, EventArgs e)
{
if (!this.IsPostBack)
{
this.BindRepeater();
}
}
private void BindRepeater()
{
string constring = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
using (SqlConnection con = new SqlConnection(constring))
{
using (SqlCommand cmd = new SqlCommand("SELECT CustomerId, Name, Country FROM Customers", con))
{
cmd.CommandType = CommandType.Text;
con.Open();
rptCustomers.DataSource = cmd.ExecuteReader();
rptCustomers.DataBind();
con.Close();
}
}
}
protected void Edit(object sender, EventArgs e)
{
if (btnEdit.Text != "Save")
{
foreach (RepeaterItem item in rptCustomers.Items)
{
Literal litCountry = item.FindControl("litCountry") as Literal;
TextBox txtCountry = item.FindControl("txtCountry") as TextBox;
litCountry.Visible = false;
txtCountry.Visible = true;
}
btnEdit.Text = "Save";
}
else
{
foreach (RepeaterItem item in rptCustomers.Items)
{
Literal litId = item.FindControl("litCustomerId") as Literal;
TextBox txtCountry = item.FindControl("txtCountry") as TextBox;
string constring = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
using (SqlConnection con = new SqlConnection(constring))
{
using(SqlCommand cmd= new SqlCommand("UPDATE Customers SET Country=@Country WHERE CustomerId=@CustomerId"))
{
cmd.CommandType = CommandType.Text;
cmd.Parameters.AddWithValue("@Country", txtCountry.Text);
cmd.Parameters.AddWithValue("@CustomerId", litId.Text);
cmd.Connection=con;
con.Open();
cmd.ExecuteNonQuery();
con.Close();
}
}
}
btnEdit.Text = "Edit";
this.BindRepeater();
}
}
VB.Net
Protected Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs) Handles Me.Load
If Not Me.IsPostBack Then
Me.BindRepeater()
End If
End Sub
Private Sub BindRepeater()
Dim constring As String = ConfigurationManager.ConnectionStrings("constr").ConnectionString
Using con As SqlConnection = New SqlConnection(constring)
Using cmd As SqlCommand = New SqlCommand("SELECT CustomerId, Name, Country FROM Customers", con)
cmd.CommandType = CommandType.Text
con.Open()
rptCustomers.DataSource = cmd.ExecuteReader()
rptCustomers.DataBind()
con.Close()
End Using
End Using
End Sub
Protected Sub Edit(ByVal sender As Object, ByVal e As EventArgs)
If btnEdit.Text <> "Save" Then
For Each item As RepeaterItem In rptCustomers.Items
Dim litCountry As Literal = TryCast(item.FindControl("litCountry"), Literal)
Dim txtCountry As TextBox = TryCast(item.FindControl("txtCountry"), TextBox)
litCountry.Visible = False
txtCountry.Visible = True
Next
btnEdit.Text = "Save"
Else
For Each item As RepeaterItem In rptCustomers.Items
Dim litId As Literal = TryCast(item.FindControl("litCustomerId"), Literal)
Dim txtCountry As TextBox = TryCast(item.FindControl("txtCountry"), TextBox)
Dim constring As String = ConfigurationManager.ConnectionStrings("constr").ConnectionString
Using con As SqlConnection = New SqlConnection(constring)
Using cmd As SqlCommand = New SqlCommand("UPDATE Customers SET Country=@Country WHERE CustomerId=@CustomerId")
cmd.CommandType = CommandType.Text
cmd.Parameters.AddWithValue("@Country", txtCountry.Text)
cmd.Parameters.AddWithValue("@CustomerId", litId.Text)
cmd.Connection = con
con.Open()
cmd.ExecuteNonQuery()
con.Close()
End Using
End Using
Next
btnEdit.Text = "Edit"
Me.BindRepeater()
End If
End Sub
Screenshot

Output
