Hi tanweeruddinb...,
Kindly find below sample.
Database
I have made use of the following table Customers with the schema as follows.
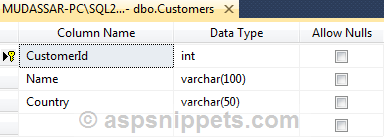
I have already inserted few records in the table.
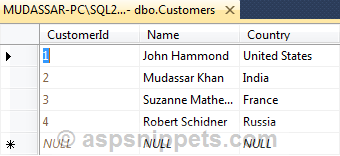
You can download the database table SQL by clicking the download link below.
Download SQL file
HTML
<asp:GridView runat="server" ID="gvCustomers" AutoGenerateColumns="false">
<Columns>
<asp:TemplateField>
<ItemTemplate>
<asp:CheckBox runat="server" AutoPostBack="true" OnCheckedChanged="OnCheckedChanged" />
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="ID">
<ItemTemplate>
<asp:Label ID="lblstu_id" Text='<%#Eval("CustomerId") %>' runat="server" />
</ItemTemplate>
</asp:TemplateField>
<asp:BoundField DataField="Name" HeaderText="Name" />
</Columns>
</asp:GridView>
<hr />
<asp:GridView runat="server" ID="gvStudents"></asp:GridView>
Namespaces
C#
using System.Configuration;
using System.Data;
using System.Data.SqlClient;
VB.Net
Imports System.Configuration
Imports System.Data
Imports System.Data.SqlClient
Code
C#
protected void Page_Load(object sender, EventArgs e)
{
if (!this.IsPostBack)
{
this.BindGrid();
}
}
private void BindGrid()
{
string conn = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
using (SqlConnection con = new SqlConnection(conn))
{
using (SqlCommand cmd = new SqlCommand("SELECT CustomerId, Name, Country FROM Customers", con))
{
using (SqlDataAdapter sda = new SqlDataAdapter(cmd))
{
using (DataTable dt = new DataTable())
{
sda.Fill(dt);
gvCustomers.DataSource = dt;
gvCustomers.DataBind();
}
}
}
}
}
public List<tblstudent> students_
{
get
{
if (ViewState["List_Student"] == null)
{
return new List<tblstudent>();
}
else
{
return (List<tblstudent>)ViewState["List_Student"];
}
}
set { ViewState["List_Student"] = value; }
}
[Serializable]
public class tblstudent
{
public string Stu_id { get; set; }
}
protected void OnCheckedChanged(object sender, EventArgs args)
{
CheckBox chkBox = sender as CheckBox;
GridViewRow item = (GridViewRow)(sender as Control).NamingContainer;
Label lblstu_id = (Label)item.FindControl("lblstu_id");
switch (chkBox.Checked)
{
case true:
List<tblstudent> students = students_;
students.Add(new tblstudent { Stu_id = lblstu_id.Text });
students_ = students;
break;
}
gvStudents.DataSource = students_;
gvStudents.DataBind();
}
VB.Net
Protected Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs) Handles Me.Load
If Not Me.IsPostBack Then
Me.BindGrid()
End If
End Sub
Private Sub BindGrid()
Dim conn As String = ConfigurationManager.ConnectionStrings("constr").ConnectionString
Using con As SqlConnection = New SqlConnection(conn)
Using cmd As SqlCommand = New SqlCommand("SELECT CustomerId, Name, Country FROM Customers", con)
Using sda As SqlDataAdapter = New SqlDataAdapter(cmd)
Using dt As DataTable = New DataTable()
sda.Fill(dt)
gvCustomers.DataSource = dt
gvCustomers.DataBind()
End Using
End Using
End Using
End Using
End Sub
Public Property students_ As List(Of tblstudent)
Get
If ViewState("List_Student") Is Nothing Then
Return New List(Of tblstudent)()
Else
Return CType(ViewState("List_Student"), List(Of tblstudent))
End If
End Get
Set(ByVal value As List(Of tblstudent))
ViewState("List_Student") = value
End Set
End Property
<Serializable>
Public Class tblstudent
Public Property Stu_id As String
End Class
Protected Sub OnCheckedChanged(ByVal sender As Object, ByVal args As EventArgs)
Dim chkBox As CheckBox = TryCast(sender, CheckBox)
Dim item As GridViewRow = CType((TryCast(sender, Control)).NamingContainer, GridViewRow)
Dim lblstu_id As Label = CType(item.FindControl("lblstu_id"), Label)
Select Case chkBox.Checked
Case True
Dim students As List(Of tblstudent) = students_
students.Add(New tblstudent With {
.Stu_id = lblstu_id.Text
})
students_ = students
End Select
gvStudents.DataSource = students_
gvStudents.DataBind()
End Sub
Screenshot