Hi tareq24bd,
Please refer below sample.
Database
I have made use of the following table Customers with the schema as follows.
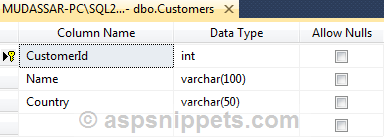
I have already inserted few records in the table.
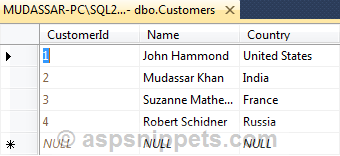
You can download the database table SQL by clicking the download link below.
Download SQL file
HTML
<asp:GridView ID="gvCustomers" AutoGenerateColumns="False" runat="server"
DataKeyNames="CustomerId" DataSourceID="SqlDataSource1">
<Columns>
<asp:BoundField DataField="CustomerId" HeaderText="CustomerId"
InsertVisible="False" ReadOnly="True" SortExpression="CustomerId" />
<asp:BoundField DataField="Name" HeaderText="Name" SortExpression="Name" />
<asp:BoundField DataField="Country" HeaderText="Country"
SortExpression="Country" />
</Columns>
</asp:GridView>
<asp:SqlDataSource ID="SqlDataSource1" runat="server"
ConnectionString="<%$ ConnectionStrings:TestConnectionString %>"
SelectCommand="SELECT * FROM [Customers]"></asp:SqlDataSource>
<asp:Button ID="Button1" Text="Export" runat="server" OnClick="Export" />
Namespaces
C#
using System.Data;
VB.Net
Imports System.Data
Code
C#
protected void Export(object sender, EventArgs e)
{
DataSet ds = new DataSet();
DataTable dt = new DataTable();
foreach (TableCell cell in gvCustomers.HeaderRow.Cells)
{
dt.Columns.Add(cell.Text);
}
foreach (GridViewRow row in gvCustomers.Rows)
{
dt.Rows.Add();
for (int i = 0; i < row.Cells.Count; i++)
{
dt.Rows[row.RowIndex][i] = row.Cells[i].Text;
}
}
ds.Tables.Add(dt);
ds.WriteXml(Server.MapPath("XMLFile.xml"));
}
VB.Net
Protected Sub Export(ByVal sender As Object, ByVal e As EventArgs)
Dim ds As DataSet = New DataSet()
Dim dt As DataTable = New DataTable()
For Each cell As TableCell In gvCustomers.HeaderRow.Cells
dt.Columns.Add(cell.Text)
Next
For Each row As GridViewRow In gvCustomers.Rows
dt.Rows.Add()
For i As Integer = 0 To row.Cells.Count - 1
dt.Rows(row.RowIndex)(i) = row.Cells(i).Text
Next
Next
ds.Tables.Add(dt)
ds.WriteXml(Server.MapPath("XMLFile.xml"))
End Sub
Generated XML
<?xml version="1.0" standalone="yes"?>
<NewDataSet>
<Table1>
<CustomerId>1</CustomerId>
<Name>John Hammond</Name>
<Country>United States</Country>
</Table1>
<Table1>
<CustomerId>2</CustomerId>
<Name>Mudassar Khan</Name>
<Country>India</Country>
</Table1>
<Table1>
<CustomerId>3</CustomerId>
<Name>Suzanne Mathews</Name>
<Country>France</Country>
</Table1>
<Table1>
<CustomerId>4</CustomerId>
<Name>Robert Schidner</Name>
<Country>Russia</Country>
</Table1>
</NewDataSet>