Hi NerakSeven,
Check this example. Now please take its reference and correct your code.
Database
For this example I have made use of the following table Customers with the schema as follows.
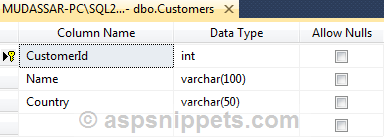
I have already inserted few records in the table.
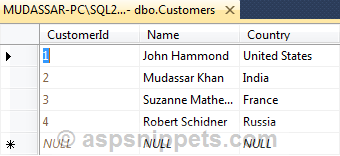
You can download the database table SQL by clicking the download link below.
Download SQL file
Namespaces
using System.Configuration;
using System.Data.SqlClient;
using System.Data;
Controller
public class HomeController : Controller
{
// GET: /Home/
public ActionResult Index()
{
string constr = ConfigurationManager.ConnectionStrings["Constring"].ConnectionString;
using (SqlConnection con = new SqlConnection(constr))
{
string query = "SELECT * FROM Customers";
using (SqlCommand cmd = new SqlCommand(query))
{
cmd.Connection = con;
con.Open();
SqlDataAdapter da = new SqlDataAdapter(cmd);
DataTable dt = new DataTable();
da.Fill(dt);
return View(dt);
}
}
}
public ActionResult MenuLibroMayor(int? id)
{
// more code.
return View();
}
}
View
<%@ Page Language="C#" Inherits="System.Web.Mvc.ViewPage<System.Data.DataTable>" %>
<%@ Import Namespace="System.Data" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title>Index</title>
<script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/jquery/1.8.3/jquery.min.js"></script>
<script type="text/javascript">
$(function () {
$('[id*=btnSend]').on('click', function () {
var id = $(this).closest('tr').find('td').eq(0).html().trim();
$.post("/Home/MenuLibroMayor", { id: id }, function (r) {
});
});
});
</script>
</head>
<body>
<table class="table">
<tr>
<% foreach (DataColumn col in Model.Columns)
{ %>
<th><%: col.ColumnName%></th>
<% } %>
<th>Action</th>
</tr>
<% foreach (DataRow row in Model.Rows)
{ %>
<tr>
<% foreach (DataColumn col in Model.Columns)
{ %>
<td><%: row[col.ColumnName]%></td>
<% } %>
<td><input type="submit" value="Send" id="btnSend" /></td>
</tr>
<% } %>
</table>
</body>
</html>
Screenshots
The Form

Id in Controller passed from View
