Hi ramco1917,
Please refer below sample.
Database
I have made use of the following table Customers with the schema as follows.
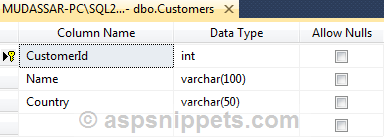
I have already inserted few records in the table.
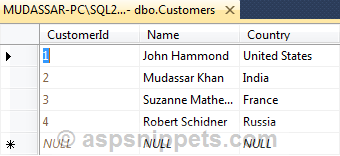
You can download the database table SQL by clicking the download link below.
Download SQL file
HTML
Name:<br />
<asp:TextBox ID="txtName" runat="server"></asp:TextBox><br /><br />
Country:<br />
<asp:TextBox ID="txtCountry" runat="server"></asp:TextBox><br /><br />
Status:<br />
<asp:DropDownList ID="ddlStatus" runat="server">
<asp:ListItem Text="Select Status" />
<asp:ListItem Text="P" Value="P" />
<asp:ListItem Text="A" Value="A" />
</asp:DropDownList><br /><br />
Remark:<br />
<asp:TextBox ID="txtRemark" runat="server"></asp:TextBox><br /><br />
<asp:Button ID="btnSubmit" runat="server" Text="Submit" OnClick="Insert" />
Namespaces
C#
using System.Data.Common;
VB.Net
Imports System.Data.Common
Code
C#
protected void Insert(object sender, EventArgs e)
{
AjaxSamplesEntities entity = new AjaxSamplesEntities();
entity.Connection.Open();
using (DbTransaction transaction = entity.Connection.BeginTransaction())
{
try
{
Customer customer = new Customer { Name = txtName.Text, Country = txtCountry.Text };
entity.Customers.AddObject(customer);
entity.SaveChanges();
Teacher teacher = new Teacher
{
CustomerID = customer.CustomerId,
Status = ddlStatus.SelectedItem.Value,
Remark = txtRemark.Text
};
entity.Teachers.AddObject(teacher);
entity.SaveChanges();
transaction.Commit();
}
catch (Exception ex)
{
transaction.Rollback();
}
}
}
VB.Net
Protected Sub Insert(ByVal sender As Object, ByVal e As EventArgs)
Dim entity As AjaxSamplesEntities = New AjaxSamplesEntities()
entity.Connection.Open()
Using transaction As DbTransaction = entity.Connection.BeginTransaction()
Try
Dim customer As Customer = New Customer With {
.Name = txtName.Text,
.Country = txtCountry.Text
}
entity.Customers.AddObject(customer)
entity.SaveChanges()
Dim teacher As Teacher = New Teacher With {
.CustomerID = customer.CustomerId,
.Status = ddlStatus.SelectedItem.Value,
.Remark = txtRemark.Text
}
entity.Teachers.AddObject(teacher)
entity.SaveChanges()
transaction.Commit()
Catch ex As Exception
transaction.Rollback()
End Try
End Using
End Sub
Screenshot

Output
