Hi rani,
Check this example. Now please take its reference and correct your code.
Database
For this example I have used table named Fruits whose schema is defined as follows.
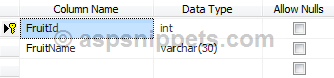
The Fruits table has the following records.
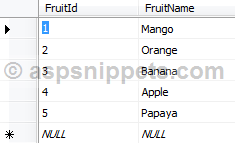
You can download the database table SQL by clicking the download link below.
Download SQL file
For reading connectrion string refer below article.
Model
Fruit
public class Fruit
{
public int FruitId { get; set; }
public string FruitName { get; set; }
}
FruitModel
public class FruitModel
{
public string Selected { get; set; }
public List<Fruit> Fruits { get; set; }
}
Controller
public class HomeController : Controller
{
private DBCtx Context { get; }
public HomeController(DBCtx _context)
{
this.Context = _context;
}
public IActionResult Index()
{
FruitModel model = new FruitModel();
model.Selected = "";
model.Fruits = (from fruit in this.Context.Fruits
select new Fruit
{
FruitId = Convert.ToInt32(fruit.FruitId),
FruitName = fruit.FruitName.ToString()
}).ToList();
return View(model);
}
[HttpPost]
public IActionResult Index(FruitModel model)
{
model.Fruits = (from fruit in this.Context.Fruits
select new Fruit
{
FruitId = Convert.ToInt32(fruit.FruitId),
FruitName = fruit.FruitName.ToString()
}).ToList();
string fruitName = model.Fruits
.Where(x => x.FruitId == Convert.ToInt32(model.Selected)).FirstOrDefault().FruitName;
ViewBag.Message = "FruitName : " + fruitName + "\\nId : " + model.Selected;
return View(model);
}
}
View
@addTagHelper*, Microsoft.AspNetCore.Mvc.TagHelpers
@using RadioButtonList_Core_MVC.Models
@model FruitModel
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Index</title>
</head>
<body>
<form asp-controller="Home" asp-action="Index" method="post">
@foreach (Fruit item in Model.Fruits)
{
<div>
<input asp-for="@Model.Selected" value="@item.FruitId" type="radio" />
<label asp-for="@item.FruitName">@item.FruitName</label>
<input asp-for="@item.FruitName" type="hidden" />
</div>
}
<br/>
<input type="submit" value="Submit" />
</form>
@if (ViewBag.Message != null)
{
<script type="text/javascript">
window.onload = function () {
alert("@ViewBag.Message");
};
</script>
}
</body>
</html>
Screenshot
