Hi Faizal,
Check this example. Now please take its reference and correct your code. Referring the below article i have created the example.
Database
I have made use of the following table Customers with the schema as follows.
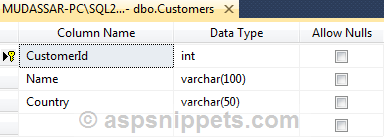
I have already inserted few records in the table.
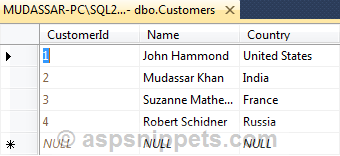
You can download the database table SQL by clicking the download link below.
Download SQL file
HTML
<div ng-app="MyApp" ng-controller="MyController">
ID:<input type="text" ng-model="Id">
<br />
Name:<input type="text" ng-model="Name">
<br />
<input type="button" value="Get Name" ng-click="GetName()" />
</div>
<script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/angularjs/1.3.9/angular.min.js"></script>
<script type="text/javascript">
var app = angular.module('MyApp', [])
app.controller('MyController', function ($scope, $http, $window) {
$scope.GetName = function () {
var post = $http({
method: "POST",
url: "Default.aspx/GetCustomerName",
dataType: 'json',
data: '{id:' + $scope.Id + '}',
headers: { "Content-Type": "application/json" }
});
post.success(function (data, status) {
$scope.Name = data.d;
});
post.error(function (data, status) {
$window.alert(data.Message);
});
}
});
</script>
Namespaces
C#
using System.Configuration;
using System.Data.SqlClient;
using System.Web.Services;
VB.Net
Imports System.Web.Services
Imports System.Data.SqlClient
Code
C#
[WebMethod]
public static string GetCustomerName(int id)
{
string name = "";
string strConnString = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
using (SqlConnection con = new SqlConnection(strConnString))
{
using (SqlCommand cmd = new SqlCommand())
{
cmd.Connection = con;
con.Open();
cmd.CommandText = "SELECT Name FROM Customers WHERE CustomerId = @Id";
cmd.Parameters.AddWithValue("@Id", id);
name = cmd.ExecuteScalar().ToString();
con.Close();
}
}
return name;
}
VB.Net
<WebMethod()>
Public Shared Function GetCustomerName(ByVal id As Integer) As String
Dim name As String = ""
Dim strConnString As String = ConfigurationManager.ConnectionStrings("constr").ConnectionString
Using con As SqlConnection = New SqlConnection(strConnString)
Using cmd As SqlCommand = New SqlCommand()
cmd.Connection = con
con.Open()
cmd.CommandText = "SELECT Name FROM Customers WHERE CustomerId = @Id"
cmd.Parameters.AddWithValue("@Id", id)
name = cmd.ExecuteScalar().ToString()
con.Close()
End Using
End Using
Return name
End Function
Screenshot
