Hi Honeyjo,
Please refer below sample.
Database
I have made use of the following table Customers with the schema as follows.
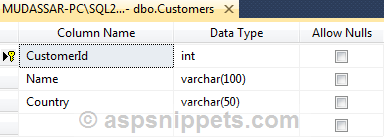
I have already inserted few records in the table.
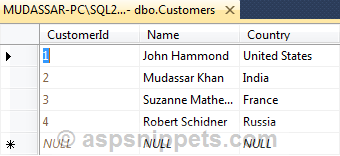
You can download the database table SQL by clicking the download link below.
Download SQL file
HTML
<cc1:ToolkitScriptManager runat="server">
</cc1:ToolkitScriptManager>
<table>
<tr>
<td><asp:Label ID="lblName" runat="server"></asp:Label></td>
</tr>
<tr>
<td><asp:Button Text="Show" ID="btnShow" runat="server" /></td>
</tr>
</table>
<cc1:ModalPopupExtender ID="mpName" runat="server" PopupControlID="Panel1" TargetControlID="btnShow">
</cc1:ModalPopupExtender>
<asp:Panel ID="Panel1" runat="server" CssClass="modalPopup" align="center" Style="display: none">
<table>
<tr>
<td>Name:</td>
<td><asp:TextBox ID="txtName" runat="server"> </asp:TextBox></td>
</tr>
<tr>
<td><asp:Button Text="Search" runat="server" ID="btnSearch" OnClick="Search" /></td>
</tr>
</table>
<br />
<asp:GridView ID="gvCustomers" runat="server" AutoGenerateColumns="false" OnRowCommand="OnRowCommand" OnSelectedIndexChanged="SelectedCustomer" DataKeyNames="CustomerId">
<Columns>
<asp:BoundField DataField="CustomerId" HeaderText="ID" />
<asp:BoundField DataField="Name" HeaderText="Name" />
<asp:BoundField DataField="Country" HeaderText="Country" />
<asp:CommandField ShowSelectButton="True" />
</Columns>
</asp:GridView>
</asp:Panel>
Namespaces
C#
using System.Data;
using System.Data.SqlClient;
using System.Configuration;
VB.Net
Imports System.Data
Imports System.Data.SqlClient
Imports System.Configuration
Code
C#
protected void Page_Load(object sender, EventArgs e)
{
if (!this.IsPostBack)
{
this.BindGrid(string.Empty);
}
}
protected void Search(object sender, EventArgs e)
{
this.BindGrid(txtName.Text.Trim());
mpName.Show();
}
protected void SelectedCustomer(object sender, EventArgs e)
{
string id = gvCustomers.SelectedRow.Cells[0].Text;
string name = gvCustomers.SelectedRow.Cells[1].Text;
mpName.Show();
}
private void BindGrid(string name)
{
string conString = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
using (SqlConnection con = new SqlConnection(conString))
{
using (SqlCommand cmd = new SqlCommand("SELECT CustomerId, Name, Country FROM Customers WHERE Name LIKE @Name + '%' OR @Name IS NULL", con))
{
if (!string.IsNullOrEmpty(name))
{
cmd.Parameters.AddWithValue("@Name", name);
}
else
{
cmd.Parameters.AddWithValue("@Name", DBNull.Value);
}
con.Open();
this.gvCustomers.DataSource = cmd.ExecuteReader();
this.gvCustomers.DataBind();
con.Close();
}
}
}
protected void OnRowCommand(object sender, GridViewCommandEventArgs e)
{
if (e.CommandName == "Select")
{
lblName.Text = (gvCustomers.Rows[Convert.ToInt16(e.CommandArgument)] as GridViewRow).Cells[1].Text;
}
}
VB.Net
Protected Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs) Handles Me.Load
If Not Me.IsPostBack Then
Me.BindGrid(String.Empty)
End If
End Sub
Protected Sub Search(ByVal sender As Object, ByVal e As EventArgs)
Me.BindGrid(txtName.Text.Trim())
mpName.Show()
End Sub
Protected Sub SelectedCustomer(ByVal sender As Object, ByVal e As EventArgs)
Dim id As String = gvCustomers.SelectedRow.Cells(0).Text
Dim name As String = gvCustomers.SelectedRow.Cells(1).Text
mpName.Show()
End Sub
Private Sub BindGrid(ByVal name As String)
Dim conString As String = ConfigurationManager.ConnectionStrings("constr").ConnectionString
Using con As SqlConnection = New SqlConnection(conString)
Using cmd As SqlCommand = New SqlCommand("SELECT CustomerId, Name, Country FROM Customers WHERE Name LIKE @Name + '%' OR @Name IS NULL", con)
If Not String.IsNullOrEmpty(name) Then
cmd.Parameters.AddWithValue("@Name", name)
Else
cmd.Parameters.AddWithValue("@Name", DBNull.Value)
End If
con.Open()
Me.gvCustomers.DataSource = cmd.ExecuteReader()
Me.gvCustomers.DataBind()
con.Close()
End Using
End Using
End Sub
Protected Sub OnRowCommand(ByVal sender As Object, ByVal e As GridViewCommandEventArgs)
If e.CommandName = "Select" Then
lblName.Text = (TryCast(gvCustomers.Rows(Convert.ToInt16(e.CommandArgument)), GridViewRow)).Cells(1).Text
End If
End Sub
Screenshot
