Hi nauna,
Check this example. Now please take its reference and correct your code.
Database
I have made use of the following table tblFiles with the schema as follows.
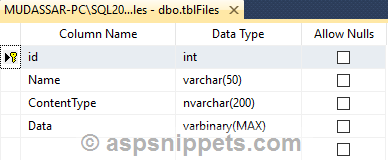
I have inserted few records in the table. You can refer below article for insert binary data.
You can download the database table SQL by clicking the download link below.
Download SQL file
HTML
<asp:ListView ID="ListView1" runat="server">
<ItemTemplate>
<asp:Label ID="Label1" runat="server" Text='<%#Eval("category_id") %>'></asp:Label>
<asp:LinkButton ID="LinkButton1" runat="server">View Detail</asp:LinkButton>
</ItemTemplate>
</asp:ListView>
<div id="MyPopup" class="modal fade" role="dialog">
<div class="modal-dialog">
<div class="modal-content">
<div class="modal-header">
<button type="button" class="close" data-dismiss="modal">
×</button>
<h4 class="modal-title text-center">Category Details
</h4>
</div>
<div class="modal-body text-center">
<asp:Label ID="lblName" runat="server" Font-Bold="true" />
<hr />
<asp:Image ID="imgCategory" runat="server" />
</div>
<div class="modal-footer">
<input type="button" id="btnClosePopup" value="Close" class="btn btn-danger" data-dismiss="modal" />
</div>
</div>
</div>
</div>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.2/css/bootstrap.min.css" />
<script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/jquery/1.9.1/jquery.min.js"></script>
<script type="text/javascript" src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.2/js/bootstrap.min.js"></script>
<script type="text/javascript">
$(function () {
$('[id*=LinkButton1]').on('click', function () {
$('#MyPopup').find('[id*=lblName]').html('');
$('#MyPopup').find('[id*=imgCategory]').attr('src', '');
var categoryId = $(this).prev('[id*=Label1]').html();
$.ajax({
type: "POST",
url: "Handler.ashx?id=" + categoryId,
contentType: "application/json; charset=utf-8",
dataType: "json",
success: function (response) {
$('#MyPopup').find('[id*=lblName]').html(response[1]);
$('#MyPopup').find('[id*=imgCategory]').attr('src', response[0]);
$('#MyPopup').modal('show');
},
error: function (response) { alert(response.responseText); }
});
return false;
});
});
</script>
Code
C#
protected void Page_Load(object sender, EventArgs e)
{
if (!this.IsPostBack)
{
System.Data.DataTable dt = new System.Data.DataTable();
dt.Columns.Add(new System.Data.DataColumn("category_id"));
dt.Rows.Add(1);
dt.Rows.Add(2);
dt.Rows.Add(3);
ListView1.DataSource = dt;
ListView1.DataBind();
}
}
VB.Net
Protected Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs) Handles Me.Load
If Not Me.IsPostBack Then
Dim dt As System.Data.DataTable = New System.Data.DataTable()
dt.Columns.Add(New System.Data.DataColumn("category_id"))
dt.Rows.Add(1)
dt.Rows.Add(2)
dt.Rows.Add(3)
ListView1.DataSource = dt
ListView1.DataBind()
End If
End Sub
Generic Handler
C#
<%@ WebHandler Language="C#" Class="Handler" %>
using System;
using System.Web;
using System.Configuration;
using System.Data.SqlClient;
using System.Web.Script.Serialization;
public class Handler : IHttpHandler
{
public void ProcessRequest(HttpContext context)
{
int categoryId = 0;
int.TryParse(context.Request.QueryString["id"], out categoryId);
JavaScriptSerializer se = new JavaScriptSerializer();
context.Response.ContentType = "text/plain";
context.Response.Write(se.Serialize(GetDetails(categoryId)));
}
public object[] GetDetails(int id)
{
object[] details = null;
string constr = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
using (SqlConnection con = new SqlConnection(constr))
{
using (SqlCommand cmd = new SqlCommand("SELECT Name,Data FROM tblFiles WHERE Id = @Id"))
{
cmd.Parameters.AddWithValue("@Id", id);
cmd.Connection = con;
con.Open();
SqlDataReader sdr = cmd.ExecuteReader();
if (sdr.Read())
{
byte[] bytes = (byte[])sdr["Data"];
string base64String = Convert.ToBase64String(bytes, 0, bytes.Length);
string imageUrl = "data:image/png;base64," + base64String;
string name = sdr["Name"].ToString();
details = new object[] { imageUrl, name };
}
con.Close();
}
}
return details;
}
public bool IsReusable
{
get
{
return false;
}
}
}
VB.Net
<%@ WebHandler Language="VB" Class="Handler" %>
Imports System
Imports System.Web
Imports System.Configuration
Imports System.Data.SqlClient
Imports System.Web.Script.Serialization
Public Class Handler : Implements IHttpHandler
Public Sub ProcessRequest(ByVal context As HttpContext) Implements IHttpHandler.ProcessRequest
Dim categoryId As Integer = 0
Integer.TryParse(context.Request.QueryString("id"), categoryId)
Dim se As JavaScriptSerializer = New JavaScriptSerializer()
context.Response.ContentType = "text/plain"
context.Response.Write(se.Serialize(GetDetails(categoryId)))
End Sub
Public Function GetDetails(ByVal id As Integer) As Object()
Dim details As Object() = Nothing
Dim constr As String = ConfigurationManager.ConnectionStrings("constr").ConnectionString
Using con As SqlConnection = New SqlConnection(constr)
Using cmd As SqlCommand = New SqlCommand("SELECT Name,Data FROM tblFiles WHERE Id = @Id")
cmd.Parameters.AddWithValue("@Id", id)
cmd.Connection = con
con.Open()
Dim sdr As SqlDataReader = cmd.ExecuteReader()
If sdr.Read() Then
Dim bytes As Byte() = CType(sdr("Data"), Byte())
Dim base64String As String = Convert.ToBase64String(bytes, 0, bytes.Length)
Dim imageUrl As String = "data:image/png;base64," & base64String
Dim name As String = sdr("Name").ToString()
details = New Object() {imageUrl, name}
End If
con.Close()
End Using
End Using
Return details
End Function
Public ReadOnly Property IsReusable() As Boolean Implements IHttpHandler.IsReusable
Get
Return False
End Get
End Property
End Class
Screenshot
