Hi rani,
Check this example. Now please take its reference and correct your code.
Database
I have made use of the following table Customers with the schema as follows.
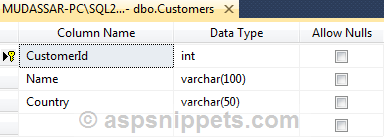
I have already inserted few records in the table.
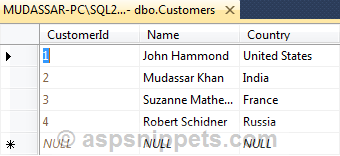
You can download the database table SQL by clicking the download link below.
Download SQL file
Model
public class Customer
{
public int CustomerId { get; set; }
public string Name { get; set; }
public string Country { get; set; }
}
Namespaces
using Microsoft.AspNetCore.Mvc;
using Microsoft.AspNetCore.Mvc.Rendering;
using Microsoft.EntityFrameworkCore;
Controller
public class HomeController : Controller
{
private DBCtx Context { get; }
public HomeController(DBCtx _context)
{
this.Context = _context;
}
public IActionResult Index()
{
List<Customer> customers = (from customer in this.Context.Customers
select customer).ToList();
List<SelectListItem> countries = (from customer in this.Context.Customers
select new SelectListItem
{
Text = customer.Country,
Value = customer.Country
}).Distinct().ToList();
countries.Insert(0, new SelectListItem { Text = "Select", Value = "" });
ViewBag.Countries = countries;
return View(customers);
}
public JsonResult InsertCustomers([FromBody]List<Customer> customers)
{
var entities = this.Context;
//Truncate Table to delete all old records.
entities.Database.ExecuteSqlCommand("TRUNCATE TABLE [Customers]");
if (customers == null)
{
customers = new List<Customer>();
}
foreach (Customer customer in customers)
{
entities.Customers.Add(customer);
}
int insertedRecords = entities.SaveChanges();
return Json(insertedRecords);
}
}
View
@addTagHelper*, Microsoft.AspNetCore.Mvc.TagHelpers
@using Dynamic_Row_Add_Core_MVC.Models;
@model IEnumerable<Customer>
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Index</title>
</head>
<body>
<table id="tblCustomers" cellpadding="0" cellspacing="0">
<thead>
<tr>
<th>Name</th>
<th>Country</th>
<th></th>
</tr>
</thead>
<tbody>
@foreach (Customer customer in Model)
{
<tr>
<td>@customer.Name</td>
<td>@customer.Country</td>
<td><input type="button" value="Remove" onclick="Remove(this)" /></td>
</tr>
}
</tbody>
<tfoot>
<tr>
<td><input type="text" id="txtName" /></td>
<td>
<select id="ddlCountries" name="Country" asp-items="@ViewBag.Countries"></select>
</td>
<td><input type="button" id="btnAdd" value="Add" /></td>
</tr>
</tfoot>
</table><br />
<input type="button" id="btnSave" value="Save All" />
<script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/jquery/1.8.3/jquery.min.js"></script>
<script type="text/javascript">
$("body").on("click", "#btnAdd", function () {
var txtName = $("#txtName");
var txtCountry = $("#ddlCountries");
var tBody = $("#tblCustomers > TBODY")[0];
var row = tBody.insertRow(-1);
var cell = $(row.insertCell(-1));
cell.html(txtName.val());
cell = $(row.insertCell(-1));
cell.html(txtCountry.val());
cell = $(row.insertCell(-1));
var btnRemove = $("<input />");
btnRemove.attr("type", "button");
btnRemove.attr("onclick", "Remove(this);");
btnRemove.val("Remove");
cell.append(btnRemove);
txtName.val("");
txtCountry.val("");
});
$("body").on("click", "#btnSave", function () {
var customers = new Array();
$("#tblCustomers TBODY TR").each(function () {
var row = $(this);
var customer = {};
customer.Name = row.find("TD").eq(0).html();
customer.Country = row.find("TD").eq(1).html();
customers.push(customer);
});
//Send the JSON array to Controller using AJAX.
$.ajax({
type: "POST",
url: "/Home/InsertCustomers",
data: JSON.stringify(customers),
contentType: "application/json; charset=utf-8",
dataType: "json",
success: function (r) {
alert(r + " record(s) inserted.");
}
});
});
function Remove(button) {
var row = $(button).closest("TR");
var name = $("TD", row).eq(0).html();
if (confirm("Do you want to delete: " + name)) {
var table = $("#tblCustomers")[0];
table.deleteRow(row[0].rowIndex);
}
};
</script>
</body>
</html>