Hi Dedzil,
Please refer below sample.
Database
I have made use of the following table Customers with the schema as follows.
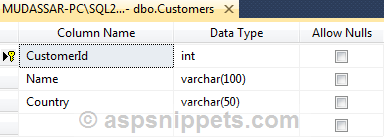
I have already inserted few records in the table.
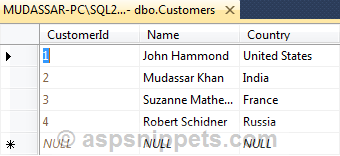
You can download the database table SQL by clicking the download link below.
Download SQL file
HTML
<asp:DropDownList ID="ddlCountries" runat="server"></asp:DropDownList>
<asp:TextBox ID="txtOther" runat="server" />
<script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/jquery/1.8.3/jquery.min.js"></script>
<script type="text/javascript">
$(function () {
$("[id*=ddlCountries]").on('change', function () {
var country = $("[id*=ddlCountries]").find("option:selected").text();
$.ajax({
type: "POST",
url: "Default.aspx/GetData",
data: "{country:'" + country + "'}",
contentType: "application/json; charset=utf-8",
dataType: "json",
success: function (data) {
$('[id*=txtOther]').val(data.d.Name);
},
error: function (response) {
alert(response.responseText)
}
});
});
});
</script>
Namespaces
C#
using System.Data;
using System.Data.SqlClient;
using System.Configuration;
using System.Web.Services;
VB.Net
Imports System.Data
Imports System.Data.SqlClient
Imports System.Configuration
Imports System.Web.Services
Code
C#
protected void Page_Load(object sender, EventArgs e)
{
if (!this.IsPostBack)
{
string conString = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
using (SqlConnection con = new SqlConnection(conString))
{
using (SqlCommand cmd = new SqlCommand("SELECT Country FROM Customers", con))
{
con.Open();
ddlCountries.DataSource = cmd.ExecuteReader();
ddlCountries.DataTextField = "Country";
ddlCountries.DataValueField = "Country";
ddlCountries.DataBind();
con.Close();
}
}
}
}
[WebMethod]
public static object GetData(string country)
{
string constr = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
using (SqlConnection con = new SqlConnection(constr))
{
string query = "SELECT * FROM Customers WHERE Country = @Country";
using (SqlCommand cmd = new SqlCommand(query))
{
cmd.Connection = con;
cmd.Parameters.Add("@Country", SqlDbType.VarChar).Value = country;
con.Open();
SqlDataReader sdr = cmd.ExecuteReader();
if (sdr.Read())
{
return new { Name = sdr["Name"], Id = sdr["CustomerId"] };
}
con.Close();
}
return null;
}
}
VB.Net
Protected Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs) Handles Me.Load
If Not Me.IsPostBack Then
Dim conString As String = ConfigurationManager.ConnectionStrings("constr").ConnectionString
Using con As SqlConnection = New SqlConnection(conString)
Using cmd As SqlCommand = New SqlCommand("SELECT Country FROM Customers", con)
con.Open()
ddlCountries.DataSource = cmd.ExecuteReader()
ddlCountries.DataTextField = "Country"
ddlCountries.DataValueField = "Country"
ddlCountries.DataBind()
con.Close()
End Using
End Using
End If
End Sub
<WebMethod>
Public Shared Function GetData(ByVal country As String) As Object
Dim constr As String = ConfigurationManager.ConnectionStrings("constr").ConnectionString
Using con As SqlConnection = New SqlConnection(constr)
Dim query As String = "SELECT * FROM Customers WHERE Country = @Country"
Using cmd As SqlCommand = New SqlCommand(query)
cmd.Connection = con
cmd.Parameters.Add("@Country", SqlDbType.VarChar).Value = country
con.Open()
Dim sdr As SqlDataReader = cmd.ExecuteReader()
If sdr.Read() Then
Return New With {
.Name = sdr("Name"),
.Id = sdr("CustomerId")
}
End If
con.Close()
End Using
Return Nothing
End Using
End Function
Screenshot
