Hi simflex,
Please refer below sample.
Database
I have made use of the following table Customers with the schema as follows.
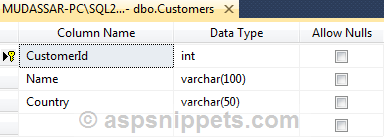
I have already inserted few records in the table.
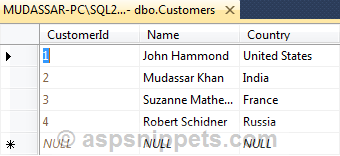
You can download the database table SQL by clicking the download link below.
Download SQL file
HTML
<table style="padding: 3px; border-spacing: 3px; background-color: white; padding-top: 40px; padding-bottom: 20px; padding-left: 100px; font-size: 14pt; color: Black; width: 90%; margin-left: auto; margin-right: auto;">
<tr>
<td>Total # of assets at property:</td>
<td>
<asp:DropDownList ID="assets" CssClass="ChangeWidth" runat="server" OnSelectedIndexChanged="assets_SelectedIndexChanged" AutoPostBack="true">
</asp:DropDownList>
</td>
<td>How many assets did you upgrade?
<asp:DropDownList ID="ddlNumber" CssClass="ChangeWidth" runat="server" OnSelectedIndexChanged="ddlNumber_SelectedIndexChanged" AutoPostBack="true" />
</td>
</tr>
</table>
<div style="background-color: White; font-size: 14pt; color: Black; background-color: white; white-space: nowrap; padding: 5px; width: 90%; margin: 0 auto;" class="popupdiv">
<asp:Repeater ID="DynamicRepeater" runat="server" EnableViewState="true" OnItemDataBound="OnItemDataBound">
<HeaderTemplate>
<table border="1" style="background-color: White; width: 90%; border-color: lavender; border-collapse: collapse;">
<tr>
<th style="text-align: left;">The Assets </th>
<th style="text-align: left;">Cost </th>
<th style="text-align: left;">Purchase Date </th>
<th>Price</th>
</tr>
</HeaderTemplate>
<ItemTemplate>
<tr>
<td>
<asp:DropDownList ID="assetChecked" class="ChangeWidth" AppendDataBoundItems="true" runat="server">
<asp:ListItem Text="Select" Selected="True" Value="0" />
</asp:DropDownList>
<asp:HiddenField ID="hfAsset" runat="server" Value='<%# DataBinder.Eval(Container.DataItem, "Asset") %>' />
</td>
<td>
<asp:TextBox ID="txt_Cost" Style="width: 200px" Text='<%# DataBinder.Eval(Container.DataItem, "Cost") %>' runat="server"></asp:TextBox></td>
<td>
<asp:TextBox ID="PurchaseDate" Style="width: 200px" Text='<%# DataBinder.Eval(Container.DataItem, "PurchaseDate") %>' runat="server"></asp:TextBox>
</td>
<td>
<asp:TextBox ID="txtInput" runat="server" Text='<%# DataBinder.Eval(Container.DataItem, "Price") %>'></asp:TextBox>
</td>
</tr>
</ItemTemplate>
<FooterTemplate>
</table>
</FooterTemplate>
</asp:Repeater>
</div>
Namespace
C#
using System.Data;
using System.Data.SqlClient;
using System.Configuration;
VB.Net
Imports System.Data
Imports System.Configuration
Imports System.Data.SqlClient
Code
C#
protected void Page_Load(object sender, EventArgs e)
{
if (!this.IsPostBack)
{
//Populates the assets dropdownlist.
assets.DataSource = GetData("SELECT * FROM Customers");
assets.DataTextField = "Country";
assets.DataValueField = "CustomerID";
assets.DataBind();
assets.Items.Insert(0, new ListItem("--Select--", ""));
}
}
//Populates the assetchecked dropdownlist.
private DataTable GetData(string query)
{
DataTable dt = new DataTable();
string constring = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
using (SqlConnection con = new SqlConnection(constring))
{
using (SqlCommand cmd = new SqlCommand(query, con))
{
using (SqlDataAdapter sda = new SqlDataAdapter(cmd))
{
sda.Fill(dt);
}
}
}
return dt;
}
protected void assets_SelectedIndexChanged(object sender, EventArgs e)
{
DataTable dt = new DataTable();
dt.Columns.Add("Country", typeof(int));
dt.Columns.Add("CustomerId", typeof(int));
//Dropdownlist change event for assets to populate the ddlNumber where assets.selectedvalue column is CustomerId.
//If CustomerId = 1 is selected
if (assets.SelectedValue == "1")
{
dt.Rows.Add("1", "1");
ddlNumber.DataSource = dt;
ddlNumber.DataTextField = "Country";
ddlNumber.DataValueField = "Country";
ddlNumber.DataBind();
ddlNumber.Items.Insert(0, new ListItem("--Select--", ""));
}
//If CustomerId = 2 is selected
else if (assets.SelectedValue == "2")
{
dt.Rows.Add("1", "1");
dt.Rows.Add("2", "2");
ddlNumber.DataSource = dt;
ddlNumber.DataTextField = "Country";
ddlNumber.DataValueField = "Country";
ddlNumber.DataBind();
ddlNumber.Items.Insert(0, new ListItem("--Select--", ""));
}
//If CustomerId = 3 is selected
else if (int.Parse(assets.SelectedValue) >= int.Parse("3"))
{
dt.Rows.Add("1", "1");
dt.Rows.Add("2", "2");
dt.Rows.Add("3", "3");
ddlNumber.DataSource = dt;
ddlNumber.DataTextField = "Country";
ddlNumber.DataValueField = "Country";
ddlNumber.DataBind();
ddlNumber.Items.Insert(0, new ListItem("--Select--", ""));
}
}
protected void ddlNumber_SelectedIndexChanged(object sender, EventArgs e)
{
//Creating the dynamic table to save the textbox values.
DataTable dt = new DataTable();
dt.Columns.AddRange(new DataColumn[] {
new DataColumn("Asset"),
new DataColumn("Cost"),
new DataColumn("PurchaseDate"),
new DataColumn("Price")
});
int rows = 0;
int.TryParse(ddlNumber.SelectedValue, out rows);
for (int i = 0; i < rows; i++)
{
//Loop through the Dynamic Repeater
if (DynamicRepeater.Items.Count > i)
{
RepeaterItem item = DynamicRepeater.Items[i];
DropDownList asset = (DropDownList)item.FindControl("assetChecked");
TextBox txt_cost = (TextBox)item.FindControl("txt_Cost");
TextBox purchaseDate = (TextBox)item.FindControl("PurchaseDate");
TextBox price = (TextBox)item.FindControl("txtInput");
if (!string.IsNullOrEmpty(price.Text) && !string.IsNullOrEmpty(txt_cost.Text) && !string.IsNullOrEmpty(purchaseDate.Text))
{
//Creating the variables to store the value of textbox and dropdownlist selected index.
string assetValue = asset.SelectedItem.Text;
string cost = txt_cost.Text;
string purchasedate = purchaseDate.Text;
string Price = price.Text;
//Inserting all the variable values to dynamic datatable.
dt.Rows.Add(assetValue, cost, purchasedate, Price);
}
}
else
{
//Inserting new empty row.
dt.Rows.Add("", "", "", "");
}
}
//populating datatable in dynamic repeater.
DynamicRepeater.DataSource = dt;
DynamicRepeater.DataBind();
}
protected void OnItemDataBound(object sender, RepeaterItemEventArgs e)
{
if (e.Item.ItemType == ListItemType.Item || e.Item.ItemType == ListItemType.AlternatingItem)
{
HiddenField hfAsset = (e.Item.FindControl("hfAsset") as HiddenField);
DropDownList assetChecked = (e.Item.FindControl("assetChecked") as DropDownList);
assetChecked.DataSource = GetData("SELECT DISTINCT Country FROM Customers");
assetChecked.DataTextField = "Country";
assetChecked.DataValueField = "Country";
assetChecked.DataBind();
assetChecked.ClearSelection();
//Checking the value of hiddenfield.
if (assetChecked.Items.FindByText(hfAsset.Value) != null)
{
//Assigning the value of hideenfield to dropdownlist.
assetChecked.Items.FindByText(hfAsset.Value).Selected = true;
}
}
}
VB.Net
Protected Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs) Handles Me.Load
If Not Me.IsPostBack Then
'Populates the assets dropdownlist.
assets.DataSource = GetData("SELECT * FROM Customers")
assets.DataTextField = "Country"
assets.DataValueField = "CustomerID"
assets.DataBind()
assets.Items.Insert(0, New ListItem("--Select--", ""))
End If
End Sub
'Populates the assetchecked dropdownlist.
Private Function GetData(ByVal query As String) As DataTable
Dim dt As DataTable = New DataTable()
Dim constring As String = ConfigurationManager.ConnectionStrings("constr").ConnectionString
Using con As SqlConnection = New SqlConnection(constring)
Using cmd As SqlCommand = New SqlCommand(query, con)
Using sda As SqlDataAdapter = New SqlDataAdapter(cmd)
sda.Fill(dt)
End Using
End Using
End Using
Return dt
End Function
Protected Sub assets_SelectedIndexChanged(ByVal sender As Object, ByVal e As EventArgs)
Dim dt As DataTable = New DataTable()
dt.Columns.Add("Country", GetType(Integer))
dt.Columns.Add("CustomerId", GetType(Integer))
'Dropdownlist change event for assets to populate the ddlNumber where assets.selectedvalue column Is CustomerId.
'If CustomerId = 1 Is selected
If assets.SelectedValue = "1" Then
dt.Rows.Add("1", "1")
ddlNumber.DataSource = dt
ddlNumber.DataTextField = "Country"
ddlNumber.DataValueField = "Country"
ddlNumber.DataBind()
ddlNumber.Items.Insert(0, New ListItem("--Select--", ""))
'If CustomerId = 2 is selected
ElseIf assets.SelectedValue = "2" Then
dt.Rows.Add("1", "1")
dt.Rows.Add("2", "2")
ddlNumber.DataSource = dt
ddlNumber.DataTextField = "Country"
ddlNumber.DataValueField = "Country"
ddlNumber.DataBind()
ddlNumber.Items.Insert(0, New ListItem("--Select--", ""))
'If CustomerId = 3 is selected
ElseIf Integer.Parse(assets.SelectedValue) >= Integer.Parse("3") Then
dt.Rows.Add("1", "1")
dt.Rows.Add("2", "2")
dt.Rows.Add("3", "3")
ddlNumber.DataSource = dt
ddlNumber.DataTextField = "Country"
ddlNumber.DataValueField = "Country"
ddlNumber.DataBind()
ddlNumber.Items.Insert(0, New ListItem("--Select--", ""))
End If
End Sub
Protected Sub ddlNumber_SelectedIndexChanged(ByVal sender As Object, ByVal e As EventArgs)
'Creating the dynamic table to save the textbox values.
Dim dt As DataTable = New DataTable()
dt.Columns.AddRange(New DataColumn() {New DataColumn("Asset"), New DataColumn("Cost"), New DataColumn("PurchaseDate"), New DataColumn("Price")})
Dim rows As Integer = 0
Integer.TryParse(ddlNumber.SelectedValue, rows)
For i As Integer = 0 To rows - 1
'Loop through the Dynamic Repeater
If DynamicRepeater.Items.Count > i Then
Dim item As RepeaterItem = DynamicRepeater.Items(i)
Dim asset As DropDownList = CType(item.FindControl("assetChecked"), DropDownList)
Dim txt_cost As TextBox = CType(item.FindControl("txt_Cost"), TextBox)
Dim purchaseDate1 As TextBox = CType(item.FindControl("PurchaseDate"), TextBox)
Dim Price1 As TextBox = CType(item.FindControl("txtInput"), TextBox)
If Not String.IsNullOrEmpty(Price1.Text) AndAlso Not String.IsNullOrEmpty(txt_cost.Text) AndAlso Not String.IsNullOrEmpty(purchaseDate1.Text) Then
'Creating the variables to store the value of textbox and dropdownlist selected index.
Dim assetValue As String = asset.SelectedItem.Text
Dim cost As String = txt_cost.Text
Dim purchasedate As String = purchaseDate1.Text
Dim Price As String = Price1.Text
'Inserting all the variable values to dynamic datatable.
dt.Rows.Add(assetValue, cost, purchasedate, Price)
End If
Else
'Inserting new empty row.
dt.Rows.Add("", "", "", "")
End If
Next
'populating datatable in dynamic repeater.
DynamicRepeater.DataSource = dt
DynamicRepeater.DataBind()
End Sub
Protected Sub OnItemDataBound(ByVal sender As Object, ByVal e As RepeaterItemEventArgs)
If e.Item.ItemType = ListItemType.Item OrElse e.Item.ItemType = ListItemType.AlternatingItem Then
Dim hfAsset As HiddenField = (TryCast(e.Item.FindControl("hfAsset"), HiddenField))
Dim assetChecked As DropDownList = (TryCast(e.Item.FindControl("assetChecked"), DropDownList))
assetChecked.DataSource = GetData("SELECT DISTINCT Country FROM Customers")
assetChecked.DataTextField = "Country"
assetChecked.DataValueField = "Country"
assetChecked.DataBind()
assetChecked.ClearSelection()
'Checking the value of hiddenfield.
If assetChecked.Items.FindByText(hfAsset.Value) IsNot Nothing Then
'Assigning the value of hideenfield to dropdownlist.
assetChecked.Items.FindByText(hfAsset.Value).Selected = True
End If
End If
End Sub
Screenshot
