Hi rani,
Check this example. Now please take its reference and correct your code.
Database
I have made use of the following table Customers with the schema as follows. CustomerId is Identity column.
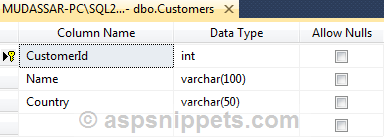
I have already inserted few records in the table.
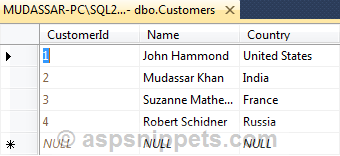
You can download the database table SQL by clicking the download link below.
Download SQL file
Model
public class Customer
{
public int CustomerId{ get; set; }
public string Name { get; set; }
public string Country { get; set; }
}
Controller
public class HomeController : Controller
{
private DBCtx Context { get; }
public HomeController(DBCtx _context)
{
this.Context = _context;
}
public IActionResult Index()
{
ViewBag.Customers = this.Context.Customers.ToList();
return View();
}
[HttpPost]
public IActionResult Index(Customer customer)
{
this.Context.Customers.Add(customer);
this.Context.SaveChanges();
ViewBag.Id = customer.CustomerId;
ViewBag.Customers = this.Context.Customers.ToList();
return View();
}
}
View
@addTagHelper*, Microsoft.AspNetCore.Mvc.TagHelpers
@using Last_Id_Entity_Framework_Core_MVC.Models
@model Customer
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Index</title>
</head>
<body>
<form asp-action="Index" asp-controller="Home" method="post">
Name: <input type="text" asp-for="@Model.Name" />
<br /><br />
Country: <input type="text" asp-for="@Model.Country" />
<br /><br />
<input type="submit" id="btnSubmit" value="Submit" />
</form>
@if (ViewBag.Customers != null)
{
<hr />
<table>
<thead>
<tr>
<th>Id</th>
<th>Name</th>
<th>Country</th>
</tr>
</thead>
<tbody>
@foreach (Customer customer in ViewBag.Customers)
{
<tr>
<td>@customer.CustomerId</td>
<td>@customer.Name</td>
<td>@customer.Country</td>
</tr>
}
</tbody>
</table>
}
@if (ViewBag.Id != null)
{
<script type="text/javascript">
window.onload = function () {
alert('Last Inserted Id : '+'@ViewBag.Id');
};
</script>
}
</body>
</html>
Screenshot
