Hi onvar1196,
Refer the below sample.
Database
I have made use of the following table Customers with the schema as follows.
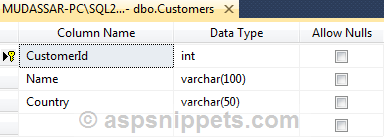
I have already inserted few records in the table.
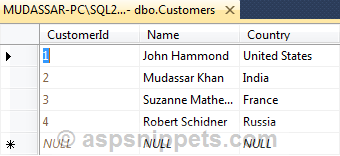
You can download the database table SQL by clicking the download link below.
Download SQL file
HTML
<table>
<% foreach (Customer customer in this.Customers)
{ %>
<tr>
<td>
<label><%=customer.Id %></label></td>
<td>
<input type="text" value='<%=customer.Name %>' /></td>
<td>
<input type="text" value='<%=customer.Country %>' /></td>
<td>
<input type="radio" <%=customer.Country != "India" ? "checked" : "" %> /></td>
<td>
<input type="checkbox" <%=customer.Country == "India" ? "checked" : "" %> /></td>
</tr>
<% } %>
</table>
Class
public class Customer
{
public int Id { get; set; }
public string Name { get; set; }
public string Country { get; set; }
}
Namespaces
using System.Collections.Generic;
using System.Configuration;
using System.Data.SqlClient;
Code
public List<Customer> Customers { get; set; }
protected void Page_Load(object sender, EventArgs e)
{
if (!this.IsPostBack)
{
this.Customers = this.GetCustomers();
}
}
public List<Customer> GetCustomers()
{
List<Customer> customers = new List<Customer>();
using (SqlConnection connection = new SqlConnection(ConfigurationManager.ConnectionStrings["constr"].ToString()))
{
using (SqlCommand command = new SqlCommand("SELECT CustomerId, Name, Country FROM Customers", connection))
{
connection.Open();
using (SqlDataReader dr = command.ExecuteReader())
{
while (dr.Read())
{
customers.Add(new Customer
{
Id = Convert.ToInt32(dr["CustomerId"]),
Name = dr["Name"].ToString(),
Country = dr["Country"].ToString()
});
}
}
connection.Close();
}
}
return customers;
}
Screenshot
