Hi Mehram,
Check this example. Now please take its reference and correct your code.
Database
I have made use of the following table Fruits with the schema as follows.
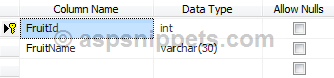
I have already inserted few records in the table.
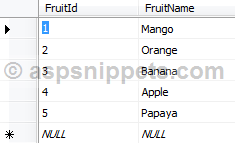
You can download the database table SQL by clicking the download link below.
Download SQL file
Namespaces
using System.Collections.Generic;
using System.Data;
using System.Data.SqlClient;
using Microsoft.AspNetCore.Mvc;
using Microsoft.AspNetCore.Mvc.Rendering;
Model
public class FruitModel
{
public int FruitId { get; set; }
public string FruitName { get; set; }
}
DataAccess
public static class DataAccess
{
public static SqlConnection GetConnection(string conString)
{
SqlConnection con = new SqlConnection(conString);
return con;
}
public static void OpenConnection(SqlConnection con)
{
if (con.State == ConnectionState.Closed)
{
con.Open();
}
}
public static void CloseConnection(SqlConnection con)
{
if (con.State == ConnectionState.Open)
{
con.Close();
}
}
public static SqlCommand SetCommand(SqlConnection con, string query,
CommandType commandType = CommandType.Text, List<SqlParameter> parameters = null)
{
SqlCommand cmd = new SqlCommand(query, con);
cmd.CommandType = commandType;
if (parameters != null)
{
foreach (SqlParameter param in parameters)
{
cmd.Parameters.Add(param);
}
}
return cmd;
}
}
Controller
public class HomeController : Controller
{
public IActionResult Index()
{
List<FruitModel> fruits = PopulateFruits();
return View(new SelectList(fruits, "FruitId", "FruitName"));
}
[HttpPost]
public IActionResult Index(string fruitId, string fruitName)
{
ViewBag.Message = "Fruit Name: " + fruitName;
ViewBag.Message += "\\nFruit Id: " + fruitId;
return View();
}
private static List<FruitModel> PopulateFruits()
{
string constr = @"Data Source=.;Initial Catalog=Sample;uid=sa;pwd=pass@123;";
List<FruitModel> fruits = new List<FruitModel>();
// Set SqlConnection object.
SqlConnection con = DataAccess.GetConnection(constr);
// Set SqlCommand object.
SqlCommand cmd = DataAccess.SetCommand(con, "SELECT FruitName, FruitId FROM Fruits");
// Open Connection.
DataAccess.OpenConnection(con);
using (SqlDataReader sdr = cmd.ExecuteReader())
{
while (sdr.Read())
{
fruits.Add(new FruitModel
{
FruitName = sdr["FruitName"].ToString(),
FruitId = Convert.ToInt32(sdr["FruitId"])
});
}
}
// Close Connection.
DataAccess.CloseConnection(con);
return fruits;
}
}
View
@addTagHelper *, Microsoft.AspNetCore.Mvc.TagHelpers
@model SelectList
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Index</title>
</head>
<body>
<form method="post" asp-controller="Home" asp-action="Index">
<select id="ddlFruits" name="FruitId" asp-items="Model" onchange="fruitChaged(this)">
<option value="0">Please select</option>
</select>
<input type="hidden" name="FruitName" />
<input type="submit" value="Submit" />
<script type="text/javascript">
function fruitChaged(ddlFruits) {
var selectedText = ddlFruits.options[ddlFruits.selectedIndex].innerHTML;
document.getElementsByName("FruitName")[0].value = selectedText;
}
</script>
@if (ViewBag.Message != null)
{
<script type="text/javascript">
window.onload = function () {
alert("@ViewBag.Message");
}
</script>
}
</form>
</body>
</html>
Screenshot
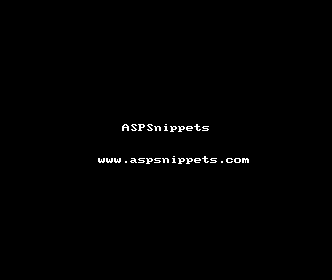