Hi rani,
Check this example. Now please take its reference and correct your code.
For exporting to word you need to install DocumentFormat.OpenXml library from nuget.
Refer below link.
DocumentFormat.OpenXml
Database
For this example I have used of Northwind database that you can download using the link given below.
Download Northwind Database
Model
public class Customer
{
public string CustomerID { get; set; }
public string ContactName { get; set; }
public string City { get; set; }
public string Country { get; set; }
}
Namespaces
using System.IO;
using System.Text;
using DocumentFormat.OpenXml;
using DocumentFormat.OpenXml.Packaging;
using DocumentFormat.OpenXml.Wordprocessing;
Controller
public class HomeController : Controller
{
private DBCtx Context { get; }
public HomeController(DBCtx _context)
{
this.Context = _context;
}
public IActionResult Index()
{
return View(this.Context.Customers.Take(10).ToList());
}
[HttpPost]
public IActionResult Export(string GridHtml)
{
using (MemoryStream ms = new MemoryStream())
{
using (WordprocessingDocument doc =
WordprocessingDocument.Create(ms, WordprocessingDocumentType.Document))
{
MainDocumentPart mdp = doc.MainDocumentPart;
if (mdp == null)
{
mdp = doc.AddMainDocumentPart();
Document document = new Document(new Body());
document.Save(mdp);
}
string altChunkId = "AltChunkId1";
AlternativeFormatImportPart afip =
mdp.AddAlternativeFormatImportPart(AlternativeFormatImportPartType.Xhtml, altChunkId);
using (Stream stream = afip.GetStream(FileMode.Create, FileAccess.Write))
{
using (StreamWriter sw = new StreamWriter(stream, Encoding.UTF8))
{
sw.Write(GridHtml);
}
}
AltChunk altChunk = new AltChunk();
altChunk.Id = altChunkId;
mdp.Document.Body.InsertAt(altChunk, 0);
mdp.Document.Save();
}
return File(ms.ToArray(), "application/vnd.ms-word", "Grid.doc");
}
}
}
View
@addTagHelper*, Microsoft.AspNetCore.Mvc.TagHelpers
@using Export_Table_Word_Core_MVC.Models
@model IEnumerable<Customer>
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Index</title>
<script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/jquery/1.8.3/jquery.min.js"></script>
<script type="text/javascript">
$(function () {
$(".btnSubmit").click(function () {
$("input[name='GridHtml']").val($("#Grid").html());
});
});
</script>
</head>
<body>
<h4>Customers</h4>
<hr />
<div id="Grid">
<table cellpadding="5" cellspacing="0" style="border: 1px solid #ccc;font-size: 9pt;">
<tr>
<th style="background-color: #B8DBFD;border: 1px solid #ccc">CustomerID</th>
<th style="background-color: #B8DBFD;border: 1px solid #ccc">ContactName</th>
<th style="background-color: #B8DBFD;border: 1px solid #ccc">City</th>
<th style="background-color: #B8DBFD;border: 1px solid #ccc">Country</th>
</tr>
@foreach (Customer customer in Model)
{
<tr>
<td style="width:120px;border: 1px solid #ccc">@customer.CustomerID</td>
<td style="width:120px;border: 1px solid #ccc">@customer.ContactName</td>
<td style="width:120px;border: 1px solid #ccc">@customer.City</td>
<td style="width:120px;border: 1px solid #ccc">@customer.Country</td>
</tr>
}
</table>
</div>
<br />
<br />
<form asp-action="Export" asp-controller="Home" method="post">
<input type="hidden" name="GridHtml" />
<input type="submit" value="Export" class="btnSubmit" />/>
</form>
</body>
</html>
Screenshots
HTML Table
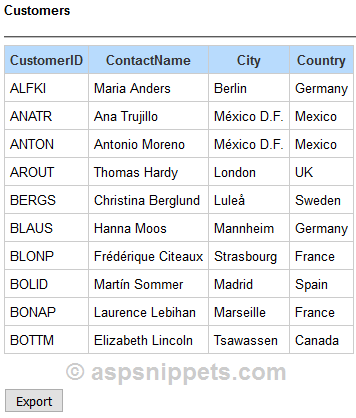
Exported Word File
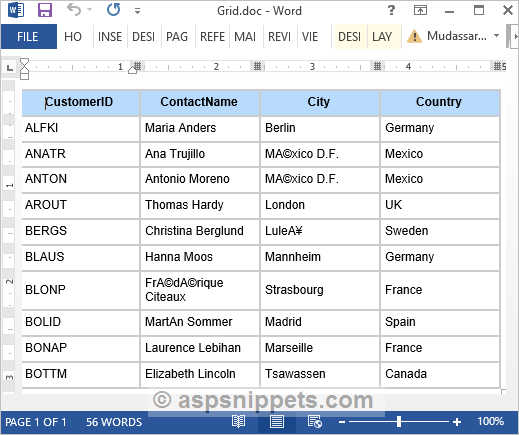