Hi Richa,
Using the below article i have created the example.
You can execute Stored Procedure using FromSql method in Entity Framework Core.
Database
For this example I have used of Northwind database that you can download using the link given below.
Download Northwind Database
SQL
--[dbo].[Customer_GetCustomerDetail] 'm'
CREATE PROCEDURE [dbo].[Customer_GetCustomerDetail]
@SearchText nvarchar(30)
AS
BEGIN
SET NOCOUNT ON
SELECT
CustomerID
,ContactName
,City
,Country
FROM Customers
WHERE ContactName like @SearchText+'%'
END
Model
public class Customer
{
public string CustomerID { get; set; }
public string ContactName { get; set; }
public string City { get; set; }
public string Country { get; set; }
}
Namespaces
using System.Data.SqlClient;
using Microsoft.AspNetCore.Mvc;
using Microsoft.EntityFrameworkCore;
using System.Linq;
Controller
public class HomeController : Controller
{
private DBCtx Context { get; }
public HomeController(DBCtx _context)
{
this.Context = _context;
}
public IActionResult Index()
{
return View();
}
[HttpPost]
public JsonResult AutoComplete(string prefix)
{
string procedureName = "dbo.Customer_GetCustomerDetail @SearchText";
SqlParameter sqlParameter = new SqlParameter("@SearchText", prefix);
var customers = Context.Customers.FromSql(procedureName, sqlParameter).ToList().
Select(x => new
{
label = x.ContactName,
val = x.CustomerID
});
return Json(customers);
}
[HttpPost]
public ActionResult Index(Customer customer)
{
ViewBag.Message = "CustomerName: " + customer.ContactName + " CustomerId: " + customer.CustomerID;
return View();
}
}
View
@addTagHelper*, Microsoft.AspNetCore.Mvc.TagHelpers
@model Procedure_Parameter_Core_MVC.Models.Customer
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Index</title>
</head>
<body>
<script src="https://ajax.aspnetcdn.com/ajax/jQuery/jquery-1.10.0.min.js" type="text/javascript"></script>
<script src="https://ajax.aspnetcdn.com/ajax/jquery.ui/1.9.2/jquery-ui.min.js" type="text/javascript"></script>
<link href="https://ajax.aspnetcdn.com/ajax/jquery.ui/1.9.2/themes/blitzer/jquery-ui.css"
rel="Stylesheet" type="text/css" />
<script type="text/javascript">
$(function () {
$("#txtCustomer").autocomplete({
source: function (request, response) {
$.ajax({
url: '/Home/AutoComplete/',
data: { "prefix": request.term },
type: "POST",
success: function (data) {
response($.map(data, function (item) {
return item;
}))
},
error: function (response) {
alert(response.responseText);
},
failure: function (response) {
alert(response.responseText);
}
});
},
select: function (e, i) {
$("#hfId").val(i.item.val);
$("#hfName").val(i.item.label);
},
minLength: 1
});
});
</script>
<form asp-action="Index" asp-controller="Home" method="post">
<input type="text" id="txtCustomer" name="CustomerName" />
<input type="hidden" id="hfId" asp-for="CustomerID" />
<input type="hidden" id="hfName" asp-for="ContactName" />
<br /><br />
<input type="submit" id="btnSubmit" value="Submit" />
<br /><br />
@ViewBag.Message
</form>
</body>
</html>
Screenshot
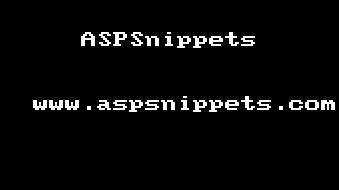