Please add a TextBox, Button and checkListBox in Form.
Form design.

Namespaces
using System.Configuration;
using System.Data.SqlClient;
C#
private void Form1_Load(object sender, EventArgs e)
{
string constring = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
using (SqlConnection con = new SqlConnection(constring))
{
using (SqlCommand cmd = new SqlCommand("SELECT [HobbyId],[Hobby] FROM [Hobbies]", con))
{
cmd.CommandType = CommandType.Text;
using (SqlDataAdapter sda = new SqlDataAdapter(cmd))
{
DataTable dt = new DataTable();
sda.Fill(dt);
((ListBox)checkedListBox1).DataSource = dt;
((ListBox)checkedListBox1).DisplayMember = "Hobby";
((ListBox)checkedListBox1).ValueMember = "HobbyId";
}
}
}
}
private void button1_Click(object sender, EventArgs e)
{
int userId = Convert.ToInt32(this.txtUserId.Text.Trim());
string constring = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
using (SqlConnection con = new SqlConnection(constring))
{
using (SqlCommand cmd = new SqlCommand("SELECT [HobbiesId] FROM UserHobbies WHERE [UserId] = @UserId", con))
{
cmd.Parameters.AddWithValue("@UserId", userId);
using (SqlDataAdapter sda = new SqlDataAdapter(cmd))
{
using (DataTable dt = new DataTable())
{
sda.Fill(dt);
string[] hobbies = dt.Rows[0]["HobbiesId"].ToString().Split(',');
foreach (string s in hobbies)
{
this.checkedListBox1.SetItemChecked(Convert.ToInt32(s), true);
}
}
}
}
}
}
DataBase
I have made use of the following table Hobbies with the schema as follows.
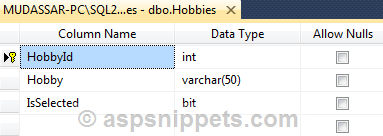
I have already inserted few records in the table
You can download the database table SQL by clicking the download link below.
Download SQL file
UserHobbies Table
SET ANSI_NULLS ON
GO
SET QUOTED_IDENTIFIER ON
GO
SET ANSI_PADDING ON
GO
CREATE TABLE [dbo].[UserHobbies](
[UserId] [int] NOT NULL,
[UserName] [varchar](50) NOT NULL,
[HobbiesId] [varchar](50) NOT NULL
) ON [PRIMARY]
GO
SET ANSI_PADDING OFF
GO
INSERT INTO [Sample].[dbo].[UserHobbies]
([UserId]
,[UserName]
,[HobbiesId])
VALUES
(1
,'Shaikh Azim'
,'1,2,3')
GO
Screenshot
