Hi anjali600,
Please refer below sample and article link.
Model
namespace CheckBoxList_EF_MVC_Core.Models
{
public class HobbyModel
{
[Key]
public int HobbyId { get; set; }
public string Hobby { get; set; }
public bool IsSelected { get; set; }
}
}
Namespaces
using Microsoft.AspNetCore.Mvc;
using Microsoft.AspNetCore.Mvc.Rendering;
Controller
namespace CheckBoxList_EF_MVC_Core.Controllers
{
public class HomeController : Controller
{
private DBCtx Context { get; }
public HomeController(DBCtx _context)
{
this.Context = _context;
}
public IActionResult Index()
{
ViewData["Hobbies"] = new SelectList(this.PopulateHobbies(), "Value", "Text", "Selected");
return View();
}
[HttpPost]
public IActionResult Index(string[] hobby)
{
ViewBag.Message = "Selected Items:\\n";
List<SelectListItem> items = this.PopulateHobbies();
foreach (SelectListItem item in items)
{
if (hobby.Contains(item.Value))
{
item.Selected = true;
ViewBag.Message += string.Format("{0}\\n", item.Text);
}
}
ViewData["Hobbies"] = new SelectList(items, "Value", "Text", "Selected");
return View();
}
private List<SelectListItem> PopulateHobbies()
{
List<SelectListItem> hobbiesList = (from p in this.Context.Hobbies
select new SelectListItem
{
Text = p.Hobby,
Value = p.HobbyId.ToString(),
Selected = p.IsSelected
}).ToList();
return hobbiesList;
}
}
}
View
<body>
<form method="post" asp-controller="Home" asp-action="Index">
<table>
@foreach (var hobby in (SelectList)ViewData["Hobbies"])
{
<tr>
<td>
<input id="@hobby.Value" type="checkbox" name="Hobby" value="@hobby.Value" />
</td>
<td>
<label for="@hobby.Value">@hobby.Text</label>
</td>
</tr>
}
</table>
<br />
<input type="submit" value="Submit" />
</form>
@if (ViewBag.Message != null)
{
<script type="text/javascript">
window.onload = function () {
alert("@ViewBag.Message");
};
</script>
}
</body>
Screenshot
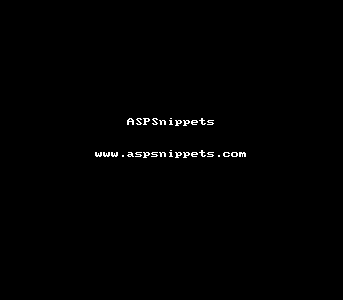