Hi 65sametkaya65,
You need to apply the click event handler to all the Image.
Refer below example.
Database
This article makes use of a table named Files whose schema is defined as follows.
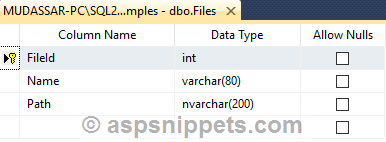
You can download the database table SQL by clicking the download link below.
Download SQL file
Controller
public ActionResult Index()
{
FilesEntities entities = new FilesEntities();
return View(entities.Files.ToList());
}
[HttpPost]
public ActionResult Index(HttpPostedFileBase postedFile)
{
//Extract Image File Name.
string fileName = System.IO.Path.GetFileName(postedFile.FileName);
//Set the Image File Path.
string filePath = "~/Uploads/" + fileName;
//Save the Image File in Folder.
postedFile.SaveAs(Server.MapPath(filePath));
//Insert the Image File details in Table.
FilesEntities entities = new FilesEntities();
entities.Files.Add(new File
{
Name = fileName,
Path = "/Uploads/" + fileName
});
entities.SaveChanges();
//Redirect to Index Action.
return RedirectToAction("Index");
}
View
@model IEnumerable<WebGrid_Image_Path_Database_EF_MVC.File>
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Index</title>
<style type="text/css">
body { font-family: Arial; font-size: 10pt; }
.myImg { border-radius: 5px; cursor: pointer; transition: 0.3s; }
.myImg:hover { opacity: 0.7; }
.modal { display: none; position: fixed; z-index: 1; padding-top: 100px; left: 0; top: 0; width: 100%; height: 100%; overflow: auto; background-color: rgb(0,0,0); background-color: rgba(0,0,0,0.9); }
.modal-content { margin: auto; display: block; width: 70%; max-width: 500px; }
#caption { margin: auto; display: block; width: 80%; max-width: 700px; text-align: center; color: #ccc; padding: 10px 0; height: 150px; }
.modal-content, #caption { -webkit-animation-name: zoom; -webkit-animation-duration: 0.6s; animation-name: zoom; animation-duration: 0.6s; }
.close { position: absolute; top: 15px; right: 35px; color: #f1f1f1; font-size: 40px; font-weight: bold; transition: 0.3s; }
.close:hover,
.close:focus { color: #bbb; text-decoration: none; cursor: pointer; }
</style>
</head>
<body>
@using (Html.BeginForm("Index", "Home", FormMethod.Post, new { enctype = "multipart/form-data" }))
{
<input type="file" name="postedFile" />
<input type="submit" id="btnUpload" value="Upload" />
}
<hr />
@foreach (var file in Model)
{
<img class="myImg" src="@file.Path" alt="@file.Name"
style="width: 100px; height: 120px; border-radius: 10px;" />
}
<div id="myModal" class="modal">
<span class="close">×</span>
<img class="modal-content" id="img01">
<div id="caption"></div>
</div>
<script type="text/javascript">
var modal = document.getElementById("myModal");
var imgs = document.getElementsByClassName("myImg");
for (var i = 0; i < imgs.length; i++) {
imgs[i].addEventListener("click", function () {
modal.style.display = "block";
document.getElementById("img01").src = this.src;
document.getElementById("caption").innerHTML = this.alt;
});
}
var span = document.getElementsByClassName("close")[0];
span.onclick = function () {
modal.style.display = "none";
}
</script>
</body>
</html>
Screenshot
