Hi dayveeyambao,
google.maps.Marker and google.maps.event.addListener is deprecated.
So instead we need to use AdvancedMarkerElement and click event is added to each marker using addListener function.
For more details using AdvancedMarkerElement, refer below link.
https://developers.google.com/maps/documentation/javascript/advanced-markers/accessible-markers#javascript
You need to create mapId using the following link.
https://developers.google.com/maps/documentation/get-map-id
Refer below sample created using the above links.
Database
I have made use of the following table Locations with the schema as follows.
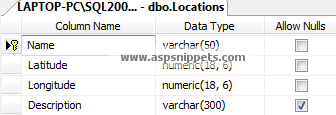
I have already inserted few records in the table.
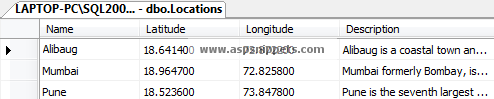
HTML
<div id="dvMap" style="width: 500px; height: 500px"></div>
Namespaces
C#
using System.Data;
using System.Data.SqlClient;
using System.Configuration;
VB.Net
Imports System.Data
Imports System.Data.SqlClient
Imports System.Configuration
Code
C#
protected void Page_Load(object sender, EventArgs e)
{
if (!this.IsPostBack)
{
string conString = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
using (SqlDataAdapter sda = new SqlDataAdapter("SELECT * FROM Locations", conString))
{
using (DataTable dt = new DataTable())
{
sda.Fill(dt);
rptMarkers.DataSource = dt;
rptMarkers.DataBind();
}
}
}
}
VB.Net
Protected Sub Page_Load(sender As Object, e As EventArgs) Handles Me.Load
If Not Me.IsPostBack Then
Dim conString As String = ConfigurationManager.ConnectionStrings("constr").ConnectionString
Using sda As New SqlDataAdapter("SELECT * FROM Locations", conString)
Using dt As New DataTable()
sda.Fill(dt)
rptMarkers.DataSource = dt
rptMarkers.DataBind()
End Using
End Using
End If
End Sub
JavaScrpt Implementation
<script type="text/javascript" src="https://maps.googleapis.com/maps/api/js?key=API_KEY&libraries=marker&loading=async"></script>
<script type="text/javascript">
var markers = [
<asp:Repeater ID="rptMarkers" runat="server">
<ItemTemplate>
{
"title": '<%# Eval("Name") %>',
"lat": '<%# Eval("Latitude") %>',
"lng": '<%# Eval("Longitude") %>',
"description": '<%# Eval("Description") %>'
}
</ItemTemplate>
<SeparatorTemplate>
,
</SeparatorTemplate>
</asp:Repeater>
];
</script>
<script type="text/javascript">
window.onload = function () {
const mapOptions = {
center: new google.maps.LatLng(markers[0].lat, markers[0].lng),
zoom: 8,
mapId: "65369bea7bc8eb7e"
};
const map = new google.maps.Map(document.getElementById("dvMap"), mapOptions);
// Create an info window.
const infoWindow = new google.maps.InfoWindow();
//Add the markers.
markers.forEach(function (location, i) {
// The advanced marker.
const marker = new google.maps.marker.AdvancedMarkerElement({
position: new google.maps.LatLng(location.lat, location.lng),
map,
title: location.title
});
// Add a click listener for each marker, and set up the info window.
marker.addListener("click", ({ domEvent, latLng }) => {
infoWindow.close();
infoWindow.setContent(location.description);
infoWindow.open(marker.map, marker);
});
});
}
</script>
<div id="dvMap" style="width: 500px; height: 500px"></div>
Screenshot
