You can put the data in Session and after postback retrieve the Session value and set to the respective controls.
Refer below example.
HTML
<table>
<tr>
<td>Name</td>
<td><asp:TextBox ID="txtName" runat="server" /></td>
</tr>
<tr>
<td>Age</td>
<td><asp:TextBox ID="txtAge" runat="server" /></td>
</tr>
<tr>
<td colspan="2"><asp:Button ID="btnSave" Text="Save" runat="server" OnClick="OnSave" /></td>
</tr>
</table>
Property Class
C#
public class Person
{
public string Name { get; set; }
public int Age { get; set; }
}
VB.Net
Public Class Person
Public Property Name As String
Public Property Age As Integer
End Class
Code
C#
protected void Page_Load(object sender, EventArgs e)
{
if (!this.IsPostBack)
{
if (Session["Person"] != null)
{
Person person = (Person)Session["Person"];
txtName.Text = person.Name;
txtAge.Text = person.Age.ToString();
}
}
}
protected void OnSave(object sender, EventArgs e)
{
Person person = new Person();
person.Name = txtName.Text;
person.Age = int.Parse(txtAge.Text);
Session["Person"] = person;
// Code for operation with the data.
Response.Redirect(Request.Url.AbsoluteUri);
}
VB.Net
Protected Sub Page_Load(sender As Object, e As EventArgs) Handles Me.Load
If Not Me.IsPostBack Then
If Session("Person") IsNot Nothing Then
Dim person As Person = CType(Session("Person"), Person)
txtName.Text = person.Name
txtAge.Text = person.Age.ToString()
End If
End If
End Sub
Protected Sub OnSave(sender As Object, e As EventArgs)
Dim person As Person = New Person()
person.Name = txtName.Text
person.Age = Integer.Parse(txtAge.Text)
Session("Person") = person
'Code for operation with the data.
Response.Redirect(Request.Url.AbsoluteUri)
End Sub
Screenshot
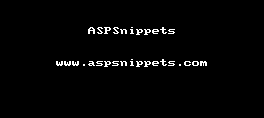