Hi micah,
Check this example. Now please take its reference and correct your code.
Database
I have made use of the following table Customers with the schema as follows.
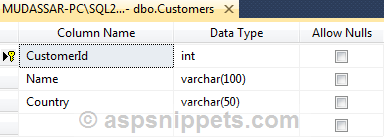
I have already inserted few records in the table.
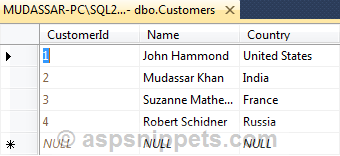
You can download the database table SQL by clicking the download link below.
Download SQL file
HTML
Name: <asp:TextBox runat="server" ID="txtName" /><br />
Country: <asp:TextBox runat="server" ID="txtCountry" /><br />
<asp:Button Text="Submit" runat="server" ID="btnSubmit" OnClick="Submit_Click" />
Namespaces
C#
using System.Configuration;
using System.Data;
using System.Data.SqlClient;
VB.Net
Imports System.Configuration
Imports System.Data
Imports System.Data.SqlClient
Code
C#
protected void Submit_Click(object sender, EventArgs e)
{
using (SqlConnection con = new SqlConnection(ConfigurationManager.ConnectionStrings["ConString"].ConnectionString))
{
using (SqlCommand cmd = new SqlCommand("SELECT Name FROM Customers WHERE Name = @Name AND Country = @Country", con))
{
cmd.CommandType = CommandType.Text;
cmd.Parameters.AddWithValue("@Name", this.txtName.Text.Trim());
cmd.Parameters.AddWithValue("@Country", this.txtCountry.Text.Trim());
con.Open();
string result = Convert.ToString(cmd.ExecuteScalar());
con.Close();
if (string.IsNullOrEmpty(result))
{
Insert(this.txtName.Text.Trim(), this.txtCountry.Text.Trim());
this.txtName.Text = "";
this.txtCountry.Text = "";
ScriptManager.RegisterStartupScript(this, this.GetType(), "Alert", "alert('Data Submitted Successfully ... !!')", true);
}
else
{
ScriptManager.RegisterStartupScript(this, this.GetType(), "Alert", "alert('Record Already Exists');", true);
}
}
}
}
private void Insert(string name, string country)
{
using (SqlConnection con = new SqlConnection(ConfigurationManager.ConnectionStrings["ConString"].ConnectionString))
{
using (SqlCommand cmd = new SqlCommand("INSERT INTO Customers VALUES (@Name, @Country)", con))
{
cmd.CommandType = CommandType.Text;
cmd.Parameters.AddWithValue("@Name", name);
cmd.Parameters.AddWithValue("@Country", country);
con.Open();
cmd.ExecuteNonQuery();
con.Close();
}
}
}
VB.Net
Protected Sub Submit_Click(ByVal sender As Object, ByVal e As EventArgs)
Using con As SqlConnection = New SqlConnection(ConfigurationManager.ConnectionStrings("ConString").ConnectionString)
Using cmd As SqlCommand = New SqlCommand("SELECT Name FROM Customers WHERE Name = @Name AND Country = @Country", con)
cmd.CommandType = CommandType.Text
cmd.Parameters.AddWithValue("@Name", Me.txtName.Text.Trim())
cmd.Parameters.AddWithValue("@Country", Me.txtCountry.Text.Trim())
con.Open()
Dim result As String = Convert.ToString(cmd.ExecuteScalar())
con.Close()
If String.IsNullOrEmpty(result) Then
Insert(Me.txtName.Text.Trim(), Me.txtCountry.Text.Trim())
Me.txtName.Text = ""
Me.txtCountry.Text = ""
ScriptManager.RegisterStartupScript(Me, Me.GetType(), "Alert", "alert('Data Submitted Successfully ... !!')", True)
Else
ScriptManager.RegisterStartupScript(Me, Me.GetType(), "Alert", "alert('Record Already Exists');", True)
End If
End Using
End Using
End Sub
Private Sub Insert(ByVal name As String, ByVal country As String)
Using con As SqlConnection = New SqlConnection(ConfigurationManager.ConnectionStrings("ConString").ConnectionString)
Using cmd As SqlCommand = New SqlCommand("INSERT INTO Customers VALUES (@Name, @Country)", con)
cmd.CommandType = CommandType.Text
cmd.Parameters.AddWithValue("@Name", name)
cmd.Parameters.AddWithValue("@Country", country)
con.Open()
cmd.ExecuteNonQuery()
con.Close()
End Using
End Using
End Sub
Screenshot
