Hi SUJAYS,
Refering the below article i have created the example.
Check this example. Now please take its reference and correct your code.
Model
public class CustomerModel
{
public int CustomerId { get; set; }
public string Name { get; set; }
public string Country { get; set; }
}
Namespaces
using System.Data;
using System.Data.OleDb;
using System.Linq;
using System.Web.Mvc;
Controller
public class HomeController : Controller
{
// GET: /Home/
public ActionResult Index()
{
return View();
}
[HttpPost]
public JsonResult GetCustomers()
{
string file = Server.MapPath("~/Files/Customers.xlsx");
string conString = "Provider=Microsoft.ACE.OLEDB.12.0;Data Source={0};Extended Properties='Excel 8.0;HDR=YES'";
conString = string.Format(conString, file);
DataTable dt = new DataTable();
using (OleDbConnection connExcel = new OleDbConnection(conString))
{
using (OleDbCommand cmdExcel = new OleDbCommand())
{
using (OleDbDataAdapter odaExcel = new OleDbDataAdapter())
{
cmdExcel.Connection = connExcel;
//Get the name of First Sheet.
connExcel.Open();
DataTable dtExcelSchema;
dtExcelSchema = connExcel.GetOleDbSchemaTable(OleDbSchemaGuid.Tables, null);
string sheetName = dtExcelSchema.Rows[0]["TABLE_NAME"].ToString();
connExcel.Close();
//Read Data from First Sheet.
connExcel.Open();
cmdExcel.CommandText = "SELECT * From [" + sheetName + "]";
odaExcel.SelectCommand = cmdExcel;
odaExcel.Fill(dt);
connExcel.Close();
}
}
}
return Json((from DataRow dr in dt.Rows
select new CustomerModel
{
CustomerId = Convert.ToInt32(dr["Id"]),
Name = dr["Name"].ToString(),
Country = dr["Country"].ToString()
}).ToList());
}
}
View
<div ng-app="MyApp" ng-controller="MyController">
<input type="button" value="Export Excel" ng-click="ExportExcel()" />
<hr />
<table ng-show="IsVisible">
<tr>
<th>
Customer Id
</th>
<th>
Name
</th>
<th>
Country
</th>
</tr>
<tbody ng-repeat="customer in Customers">
<tr>
<td>
{{customer.CustomerId}}
</td>
<td>
{{customer.Name}}
</td>
<td>
{{customer.Country}}
</td>
</tr>
</tbody>
</table>
</div>
<script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/angularjs/1.3.9/angular.min.js"></script>
<script type="text/javascript">
var app = angular.module('MyApp', [])
app.controller('MyController', function ($scope, $http, $window) {
$scope.IsVisible = false;
$scope.ExportExcel = function () {
var post = $http({
method: "POST",
url: "/Home/GetCustomers",
dataType: 'json',
data: {},
headers: { "Content-Type": "application/json" }
});
post.success(function (data, status) {
$scope.Customers = data;
$scope.IsVisible = true;
});
post.error(function (data, status) {
$window.alert(data.Message);
});
};
});
</script>
Screenshot
The Excel File
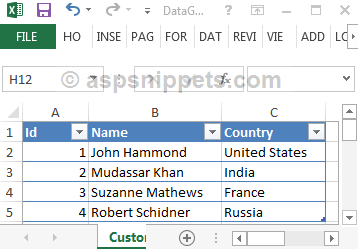
The GridView
