Hi sani.ss501,
Check this example. Now please take its reference and correct your code.
Using the article i have created the example.
Database
I have made use of the following table Customers with the schema as follows.
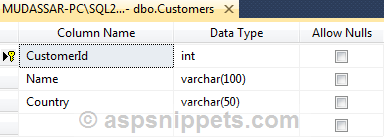
I have already inserted few records in the table.
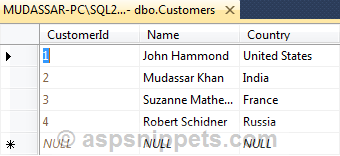
You can download the database table SQL by clicking the download link below.
Download SQL file
Model
public class CustomerModel
{
public int CustomerId { get; set; }
public string Name { get; set; }
public string Country { get; set; }
}
Namespaces
using System.Collections.Generic;
using System.Configuration;
using System.Data;
using System.Data.SqlClient;
Controller
public class HomeController : Controller
{
public ActionResult Index()
{
SqlCommand cmd = new SqlCommand("Customers_SearchCustomers");
cmd.CommandType = CommandType.StoredProcedure;
cmd.Parameters.AddWithValue("@Name", "");
List<CustomerModel> customers = GetCustomers(cmd);
return View(customers);
}
[HttpPost]
public ActionResult Index(string customerName)
{
SqlCommand cmd = new SqlCommand("Customers_SearchCustomers");
cmd.CommandType = CommandType.StoredProcedure;
cmd.Parameters.AddWithValue("@Name", customerName);
List<CustomerModel> customers = GetCustomers(cmd);
return View(customers);
}
[HttpPost]
public ActionResult UpdateCustomer(CustomerModel customer)
{
string query = "UPDATE Customers SET Name=@Name, Country=@Country WHERE CustomerId=@CustomerId";
string constr = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
using (SqlConnection con = new SqlConnection(constr))
{
using (SqlCommand cmd = new SqlCommand(query))
{
cmd.Parameters.AddWithValue("@CustomerId", customer.CustomerId);
cmd.Parameters.AddWithValue("@Name", customer.Name);
cmd.Parameters.AddWithValue("@Country", customer.Country);
cmd.Connection = con;
con.Open();
cmd.ExecuteNonQuery();
con.Close();
}
}
return new EmptyResult();
}
private static List<CustomerModel> GetCustomers(SqlCommand cmd)
{
List<CustomerModel> customers = new List<CustomerModel>();
string constr = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
using (SqlConnection con = new SqlConnection(constr))
{
cmd.Connection = con;
con.Open();
using (SqlDataReader sdr = cmd.ExecuteReader())
{
while (sdr.Read())
{
customers.Add(new CustomerModel
{
CustomerId = Convert.ToInt32(sdr["CustomerId"]),
Name = Convert.ToString(sdr["Name"]),
Country = Convert.ToString(sdr["Country"])
});
}
}
con.Close();
}
return customers;
}
}
View
<%@ Page Language="C#" Inherits="System.Web.Mvc.ViewPage<IEnumerable<CustomerModel>>" %>
<%@ Import Namespace="Add_Button_Table_Row.Models" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title>Index</title>
</head>
<body>
<%using (Html.BeginForm("Index", "Home", FormMethod.Post))
{%>
<input type="text" id="txtSearch" name="customerName" value='<%=Request["customerName"] %>' />
<input type="submit" id="btnSearch" value="Search" />
<%} %>
<table id="tblCustomers" class="table" cellpadding="0" cellspacing="0">
<tr>
<th>Customer Id</th>
<th>Name</th>
<th>Country</th>
<th></th>
</tr>
<%foreach (CustomerModel customer in Model)
{%>
<tr>
<td class="CustomerId">
<span><%=customer.CustomerId%></span>
</td>
<td class="Name">
<span><%=customer.Name%></span>
<input type="text" value="<%=customer.Name%>" style="display: none" />
</td>
<td class="Country">
<span><%=customer.Country%></span>
<input type="text" value="<%=customer.Country%>" style="display: none" />
</td>
<td>
<a class="Edit" href="javascript:;">Edit</a>
<a class="Update" href="javascript:;" style="display: none">Update</a>
<a class="Cancel" href="javascript:;" style="display: none">Cancel</a>
</td>
</tr>
<% }%>
</table>
<script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/jquery/1.9.1/jquery.min.js"></script>
<script type="text/javascript">
//Edit event handler.
$(document).on("click", "#tblCustomers .Edit", function () {
var row = $(this).closest("tr");
$("td", row).each(function () {
if ($(this).find("input").length > 0) {
$(this).find("input").show();
$(this).find("span").hide();
}
});
row.find(".Update").show();
row.find(".Cancel").show();
$(this).hide();
});
//Update event handler.
$(document).on("click", "#tblCustomers .Update", function () {
var row = $(this).closest("tr");
$("td", row).each(function () {
if ($(this).find("input").length > 0) {
var span = $(this).find("span");
var input = $(this).find("input");
span.html(input.val());
span.show();
input.hide();
}
});
row.find(".Edit").show();
row.find(".Cancel").hide();
$(this).hide();
var customer = {};
customer.CustomerId = row.find(".CustomerId").find("span").html();
customer.Name = row.find(".Name").find("span").html();
customer.Country = row.find(".Country").find("span").html();
$.ajax({
type: "POST",
url: "/Home/UpdateCustomer",
data: '{customer:' + JSON.stringify(customer) + '}',
contentType: "application/json; charset=utf-8",
dataType: "json"
});
});
//Cancel event handler.
$(document).on("click", "#tblCustomers .Cancel", function () {
var row = $(this).closest("tr");
$("td", row).each(function () {
if ($(this).find("input").length > 0) {
var span = $(this).find("span");
var input = $(this).find("input");
input.val(span.html());
span.show();
input.hide();
}
});
row.find(".Edit").show();
row.find(".Update").hide();
$(this).hide();
});
</script>
</body>
</html>