Hi Sumeet,
Check this example. Now please take its reference and correct your code.
Database
I have made use of three tables Countries, States and Cities with the following schema.
Countries
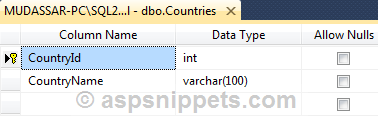
States
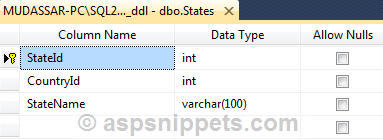
Cities
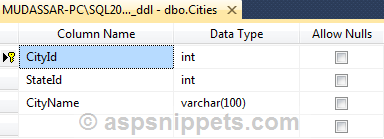
You can download the database table SQL by clicking the download link below.
Download SQL file
Stored Procedure
--EXEC sp_CascadingDDL 1,1
CREATE PROCEDURE [dbo].[sp_CascadingDDL]
@CountryId INT = 0,
@StateId INT = 0
AS
BEGIN
IF(@CountryId = 0 AND @StateId = 0)
BEGIN
SELECT '0' Id,'--Select--' Name
UNION
SELECT a.CountryId,a.CountryName
FROM Countries a
END
ELSE IF(@CountryId > 0 AND @StateId = 0)
BEGIN
SELECT '0' Id,'--Select--' Name
UNION
SELECT a.StateId,a.StateName
FROM States a
WHERE a.CountryId = @CountryId
END
ELSE IF(@CountryId > 0 AND @StateId > 0)
BEGIN
SELECT '0' Id,'--Select--' Name
UNION
SELECT a.CityId,a.CityName
FROM Cities a
WHERE a.StateId = @StateId
END
END
HTML
<table>
<tr>
<td align="right" valign="top">Country <font style="color: red;">*</font></td>
<td align="left" valign="top">
<asp:DropDownList ID="ddlCountries" runat="server" DataSourceID="sdsCountry" AutoPostBack="True"
DataTextField="Name" DataValueField="Id" OnSelectedIndexChanged="OnCountryChanged" Width="150px">
</asp:DropDownList>
<asp:SqlDataSource ID="sdsCountry" runat="server" ConnectionString="<%$ ConnectionStrings:constr %>"
SelectCommand="sp_CascadingDDL" SelectCommandType="StoredProcedure"></asp:SqlDataSource>
</td>
</tr>
<tr>
<td align="right" valign="top">State <font style="color: red;">*</font></td>
<td align="left" valign="top">
<asp:DropDownList ID="ddlStates" runat="server" DataSourceID="sdsState" AutoPostBack="True"
DataTextField="Name" DataValueField="Id" OnSelectedIndexChanged="OnStateChanged" Width="150px">
</asp:DropDownList>
<asp:SqlDataSource ID="sdsState" runat="server" ConnectionString="<%$ ConnectionStrings:constr %>"
SelectCommand="sp_CascadingDDL" SelectCommandType="StoredProcedure">
<SelectParameters>
<asp:ControlParameter ControlID="ddlCountries" Name="CountryId" PropertyName="SelectedValue"
Type="Int32" ConvertEmptyStringToNull="false" />
<asp:Parameter Name="StateId" Type="Int32" DefaultValue="0" />
</SelectParameters>
</asp:SqlDataSource>
</td>
</tr>
<tr>
<td align="right" valign="top">City <font style="color: red;">*</font></td>
<td align="left" valign="top">
<asp:DropDownList ID="ddlCities" runat="server" DataSourceID="sdsCity"
DataTextField="Name" DataValueField="Id" Width="150px">
</asp:DropDownList>
<asp:SqlDataSource ID="sdsCity" runat="server" ConnectionString="<%$ ConnectionStrings:constr %>"
SelectCommand="sp_CascadingDDL" SelectCommandType="StoredProcedure">
<SelectParameters>
<asp:ControlParameter ControlID="ddlCountries" Name="CountryId" PropertyName="SelectedValue"
Type="Int32" ConvertEmptyStringToNull="false" />
<asp:ControlParameter ControlID="ddlStates" Name="StateId" PropertyName="SelectedValue"
Type="Int32" ConvertEmptyStringToNull="false" />
</SelectParameters>
</asp:SqlDataSource>
</td>
</tr>
</table>
Code
C#
protected void Page_Load(object sender, EventArgs e)
{
if (!this.IsPostBack)
{
ddlStates.Enabled = false;
ddlCities.Enabled = false;
}
}
protected void OnCountryChanged(object sender, EventArgs e)
{
ddlStates.Enabled = true;
ddlCities.Enabled = false;
}
protected void OnStateChanged(object sender, EventArgs e)
{
ddlCities.Enabled = true;
}
VB.Net
Protected Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs) Handles Me.Load
If Not Me.IsPostBack Then
ddlStates.Enabled = False
ddlCities.Enabled = False
End If
End Sub
Protected Sub OnCountryChanged(ByVal sender As Object, ByVal e As EventArgs)
ddlStates.Enabled = True
ddlCities.Enabled = False
End Sub
Protected Sub OnStateChanged(ByVal sender As Object, ByVal e As EventArgs)
ddlCities.Enabled = True
End Sub
Screenshot
