Hi cbrAndy,
Check this example. Now please take its reference and correct your code.
Database
I have make use of a table named Fruits whose schema is defined as follows.
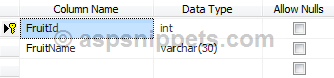
The Fruits table has the following records.
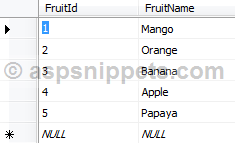
Namespaces
using System.Configuration;
using System.Data.SqlClient;
Controller
public class HomeController : Controller
{
// GET: Home
public ActionResult Index()
{
ViewBag.Fruits = PopulateFruits();
return View();
}
[HttpPost]
public ActionResult Index(int[] fruitIds)
{
ViewBag.Fruits = PopulateFruits();
if (fruitIds != null)
{
List<SelectListItem> selectedItems = PopulateFruits().Where(p => fruitIds.Contains(int.Parse(p.Value))).ToList();
ViewBag.Message = "Selected Fruits:";
foreach (var selectedItem in selectedItems)
{
selectedItem.Selected = true;
ViewBag.Message += "\\n" + selectedItem.Text;
}
}
return View();
}
private static List<SelectListItem> PopulateFruits()
{
List<SelectListItem> items = new List<SelectListItem>();
string constr = ConfigurationManager.ConnectionStrings["Constring"].ConnectionString;
using (SqlConnection con = new SqlConnection(constr))
{
string query = "SELECT FruitName, FruitId FROM Fruits";
using (SqlCommand cmd = new SqlCommand(query))
{
cmd.Connection = con;
con.Open();
using (SqlDataReader sdr = cmd.ExecuteReader())
{
while (sdr.Read())
{
items.Add(new SelectListItem
{
Text = sdr["FruitName"].ToString(),
Value = sdr["FruitId"].ToString()
});
}
}
con.Close();
}
}
return items;
}
}
View
@{
Layout = null;
}
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Index</title>
</head>
<body>
@using (Html.BeginForm("Index", "Home", FormMethod.Post))
{
@Html.Label("Fruits:")
<br /><br />
@Html.DropDownList("FruitIds", new SelectList(ViewBag.Fruits, "Value", "Text"), new { @class = "form-control", multiple = "multiple", size = "3" })
<br /><br />
<input type="submit" value="Submit" />
}
<script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/jquery/1.8.3/jquery.min.js"></script>
<link rel="stylesheet" type="text/css" href="https://cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/3.0.3/css/bootstrap.min.css" />
@if (ViewBag.Message != null)
{
<script type="text/javascript">
window.onload = function () {
alert("@ViewBag.Message");
};
</script>
}
</body>
</html>
Screenshot
