Hi rani,
Check this example. Now please take its reference and correct your code.
Database
I have made use of a table named tblFiles whose schema is defined as follows.
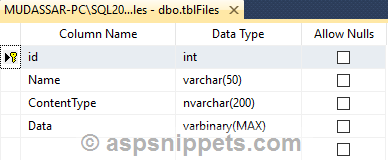
I have already inserted few records in the table.
You can download the database table SQL by clicking the download link below.
Download SQL file
Model
public class FileModel
{
public int Id { get; set; }
public string Name { get; set; }
public string ContentType { get; set; }
public byte[] Data { get; set; }
}
Controller
public class HomeController : Controller
{
private DBCtx Context { get; }
public HomeController(DBCtx _context)
{
this.Context = _context;
}
public IActionResult Index()
{
return View(this.Context.tblFiles.Where(x => x.ContentType == "image/jpeg").ToList());
}
}
View
@addTagHelper *, Microsoft.AspNetCore.Mvc.TagHelpers
@using Bootstrap_Carousel_Core_MVC.Models;
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Index</title>
</head>
<body>
<div class="container">
<div id="myCarousel" class="carousel slide" data-ride="carousel" data-interval="2000" data-pause="hover">
<!-- Indicators -->
<ol class="carousel-indicators">
@for (int i = 0; i < ((List<FileModel>)ViewBag.Images).Count; i++)
{
if (i == 0)
{
<li data-target="#myCarousel" data-slide-to="@i" class="active"></li>
}
else
{
<li data-target="#myCarousel" data-slide-to="@i"></li>
}
}
</ol>
<!-- Wrapper for slides -->
<div class="carousel-inner" role="listbox">
@for (int i = 0; i < ((List<FileModel>)ViewBag.Images).Count; i++)
{
var file = ((List<FileModel>)ViewBag.Images)[i];
if (i == 0)
{
<div class="item active">
<div class="carousel-content">
<div style="margin: 0 auto">
<p>
<img alt="@file.Name" src="data:image/jpeg;base64,@Convert.ToBase64String(file.Data)"
style="width: 100%;height: 200px" />
</p>
</div>
</div>
</div>
}
else
{
<div class="item">
<div class="carousel-content">
<div style="margin: 0 auto;text-align:center;">
<p>
<img alt="@file.Name" src="data:image/jpeg;base64,@Convert.ToBase64String(file.Data)"
style="width: 100%;height: 200px" />
</p>
</div>
</div>
</div>
}
}
</div>
<a class="left carousel-control" href="#myCarousel" role="button" data-slide="prev">
<span class="glyphicon glyphicon-chevron-left" aria-hidden="true"></span>
<span class="sr-only"> Previous</span>
</a>
<a class="right carousel-control" href="#myCarousel" role="button" data-slide="next">
<span class="glyphicon glyphicon-chevron-right" aria-hidden="true"></span>
<span class="sr-only">Next</span>
</a>
</div>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css" />
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap-theme.min.css" />
<script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/jquery/3.1.1/jquery.min.js"></script>
<script type="text/javascript" src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/js/bootstrap.min.js"></script>
<style type="text/css">
.carousel-inner {
width: auto;
height: 200px;
max-height: 200px !important;
}
.carousel-content {
color: black;
display: flex;
text-align: center;
}
</style>
<script type="text/javascript">
$(document).ready(function () {
$('.carousel').carousel();
});
</script>
</div>
</body>
</html>
Screenshot
