Hi nagaraju60,
I have created sample by refering the below article.
Check this example. Now please take its reference and correct your code.
I have made use of the following table Customers with the schema as follows.
.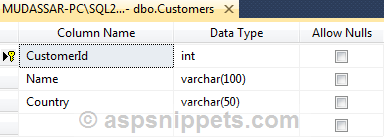
You can download the script from the below link.
Download SQL file
HTML
<asp:ScriptManager ID="ScriptManager1" runat="server" EnablePageMethods="true">
</asp:ScriptManager>
<div>
<asp:GridView ID="GridView1" runat="server" AutoGenerateColumns="false">
<Columns>
<asp:BoundField DataField="CustomerId" HeaderText="Id" />
<asp:BoundField DataField="Name" HeaderText="Name" />
<asp:BoundField DataField="Country" HeaderText="Country" />
</Columns>
</asp:GridView>
<br />
<asp:Button Text="Save" runat="server" ID="btnSave" OnClick="Save" />
</div>
<script type="text/javascript">
function SaveRecord() {
var table = document.getElementById("GridView1");
for (var i = 1; i < table.rows.length; i++) {
var row = table.rows[i];
PageMethods.SaveRow(row.cells[1].innerHTML, row.cells[2].innerHTML, function OnSuccess(response) { });
}
}
</script>
C#
protected void Page_Load(object sender, EventArgs e)
{
if (!this.IsPostBack)
{
BindGridView();
}
}
private void BindGridView()
{
DataTable dt = new DataTable();
dt.Columns.AddRange(new DataColumn[3] { new DataColumn("CustomerId", typeof(int)),
new DataColumn("Name", typeof(string)),
new DataColumn("Country",typeof(string)) });
dt.Rows.Add(1, "Mudassar Khan", "India");
dt.Rows.Add(2, "John Hammond", "United States");
dt.Rows.Add(3, "Suzanne Mathews", "France");
dt.Rows.Add(4, "Robert Schidner", "Russia");
GridView1.DataSource = dt;
GridView1.DataBind();
}
protected void Save(object sender, EventArgs e)
{
ScriptManager.RegisterStartupScript(this, this.GetType(), "", "SaveRecord();", true);
}
[System.Web.Services.WebMethod]
public static void SaveRow(string name, string country)
{
SqlConnection conn = new SqlConnection(ConfigurationManager.ConnectionStrings["constr"].ConnectionString);
SqlCommand cmd = new SqlCommand("INSERT INTO Customers (Name,Country) VALUES (@Name,@Country)", conn);
cmd.CommandType = CommandType.Text;
cmd.Parameters.AddWithValue("@Name", name);
cmd.Parameters.AddWithValue("@Country", country);
conn.Open();
cmd.ExecuteNonQuery();
}
VB.Net
Protected Sub Page_Load(sender As Object, e As EventArgs) Handles Me.Load
If Not Me.IsPostBack Then
BindGridView()
End If
End Sub
Private Sub BindGridView()
Dim dt As New DataTable()
dt.Columns.AddRange(New DataColumn(2) {New DataColumn("CustomerId", GetType(Integer)), New DataColumn("Name", GetType(String)), New DataColumn("Country", GetType(String))})
dt.Rows.Add(1, "Mudassar Khan", "India")
dt.Rows.Add(2, "John Hammond", "United States")
dt.Rows.Add(3, "Suzanne Mathews", "France")
dt.Rows.Add(4, "Robert Schidner", "Russia")
GridView1.DataSource = dt
GridView1.DataBind()
End Sub
Protected Sub Save(sender As Object, e As EventArgs)
ScriptManager.RegisterStartupScript(Me, Me.[GetType](), "", "SaveRecord();", True)
End Sub
<System.Web.Services.WebMethod()> _
Public Shared Sub SaveRow(name As String, country As String)
Dim conn As New SqlConnection(ConfigurationManager.ConnectionStrings("constr").ConnectionString)
Dim cmd As New SqlCommand("INSERT INTO Customers (Name,Country) VALUES (@Name,@Country)", conn)
cmd.CommandType = CommandType.Text
cmd.Parameters.AddWithValue("@Name", name)
cmd.Parameters.AddWithValue("@Country", country)
conn.Open()
cmd.ExecuteNonQuery()
End Sub
Output after inserting record to database.
