Hi rani,
Follow the steps.
In Startup.cs class add the Service ContextAccessor.
Startup.cs
using Microsoft.AspNetCore.Builder;
using Microsoft.AspNetCore.Hosting;
using Microsoft.AspNetCore.Mvc.Infrastructure;
using Microsoft.Extensions.DependencyInjection;
using Microsoft.Extensions.DependencyInjection.Extensions;
namespace IP_Address_Core
{
public class Startup
{
// This method gets called by the runtime. Use this method to add services to the container.
// For more information on how to configure your application, visit https://go.microsoft.com/fwlink/?LinkID=398940
public void ConfigureServices(IServiceCollection services)
{
services.AddMvc();
services.AddHttpContextAccessor();
services.TryAddSingleton<IActionContextAccessor, ActionContextAccessor>();
}
// This method gets called by the runtime. Use this method to configure the HTTP request pipeline.
public void Configure(IApplicationBuilder app, IHostingEnvironment env)
{
app.UseMvc(routes =>
{
routes.MapRoute(
name: "default",
template: "{controller=Home}/{action=Index}/{id?}");
});
}
}
}
Define a variable in your Controller to get IPAddress.
Controller
using Microsoft.AspNetCore.Http;
using Microsoft.AspNetCore.Mvc;
namespace IP_Address_Core.Controllers
{
public class HomeController : Controller
{
private IHttpContextAccessor _accessor;
public HomeController(IHttpContextAccessor accessor)
{
_accessor = accessor;
}
public IActionResult Index()
{
string ipAddress = _accessor.HttpContext.Connection.RemoteIpAddress.ToString();
ViewBag.IPAddress = ipAddress;
return View();
}
}
}
View
@addTagHelper*, Microsoft.AspNetCore.Mvc.TagHelpers
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Index</title>
</head>
<body>
<form method="post" asp-controller="Home" asp-action="Index">
Your IP Address: @ViewBag.IPAddress
</form>
</body>
</html>
Screenshot
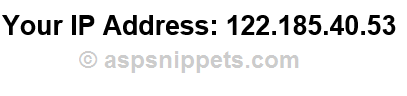