Hi jmontano,
Check this example. Now please take its reference and correct your code.
Database
For this example I have used two tables Countries and States with the schema as follows.
Countries
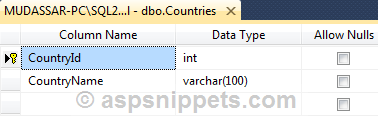
States
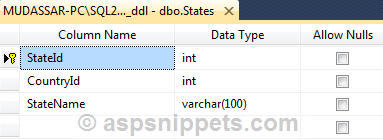
You can download the database table SQL by clicking the download link below.
Download SQL file
I have used Entity Framework to fetch the data from database tables.
For configuring Entity framework in ASP.Net Core MVC refer below article.
ASP.Net Core: Simple Entity Framework Tutorial with example
Model
Country
public class Country
{
public int CountryId { get; set; }
public string CountryName { get; set; }
}
State
public class State
{
public int StateId { get; set; }
public string StateName { get; set; }
public int CountryId { get; set; }
}
CascadingModel
public class CascadingModel
{
public List<SelectListItem> Countries { get; set; }
public List<SelectListItem> States { get; set; }
public int? CountryId { get; set; }
public int? StateId { get; set; }
}
Controller
public class HomeController : Controller
{
private DBCtx Context { get; }
public HomeController(DBCtx _context)
{
this.Context = _context;
}
public IActionResult Index()
{
CascadingModel model = new CascadingModel();
model.Countries = this.Context.Countries.Select(x => new SelectListItem
{
Text = x.CountryName,
Value = x.CountryId.ToString()
}).ToList();
model.States = this.Context.States.Select(x => new SelectListItem
{
Text = x.StateName,
Value = x.StateId.ToString()
}).ToList();
return View(model);
}
[HttpPost]
public IActionResult AjaxMethod([FromBody]AutoDetails auto)
{
List<SelectListItem> items = new List<SelectListItem>();
if (auto != null && !string.IsNullOrEmpty(auto.type))
{
switch (auto.type)
{
default:
foreach (var country in this.Context.Countries)
{
items.Add(new SelectListItem { Text = country.CountryName, Value = country.CountryId.ToString() });
}
break;
case "Country":
var states = (from state in this.Context.States
where state.CountryId == auto.value
select state).ToList();
foreach (var state in states)
{
items.Add(new SelectListItem { Text = state.StateName, Value = state.StateId.ToString() });
}
break;
}
}
return Json(items);
}
public class AutoDetails
{
public string type { get; set; }
public int value { get; set; }
}
}
View
@model Cascading_DropDownList_Core_MVC.Models.CascadingModel
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Index</title>
<script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/jquery/1.8.3/jquery.min.js"></script>
<script type="text/javascript">
$(function () {
AjaxCall({ "type": '' }, 'State');
$('#ddlCountries').on('change', function () {
var myData = {
"type": 'Country',
"value": $(this).find('option:selected').val()
};
AjaxCall(myData, 'State');
});
});
function AjaxCall(myData, element) {
$.ajax({
url: "/Home/AjaxMethod/",
data: JSON.stringify(myData),
dataType: "json",
type: "POST",
contentType: "application/json; charset=utf-8",
success: function (data) {
switch (element) {
case "Country":
$('#ddlCountries').append($("<option></option>").val("0").html("Select Country"));
$.each(data, function () {
$('#ddlCountries').append($("<option></option>").val(this.value).html(this.text));
});
$('#ddlStates').attr("disabled", "disabled");
break;
case "State":
if (data.length > 0) {
$('#ddlStates').empty();
$('#ddlStates').append($("<option></option>").val("0").html("Select State"));
$.each(data, function () {
$('#ddlStates').append($("<option></option>").val(this.value).html(this.text));
});
$('#ddlStates').prop("disabled", false);;
break;
} else {
$('#ddlStates').empty();
$('#ddlStates').append($("<option></option>").val("0").html("Select State"));
$('#ddlStates').attr("disabled", "disabled");
}
}
},
error: function (r) {
alert(r.responseText);
},
failure: function (r) {
alert(r.responseText);
}
});
}
</script>
</head>
<body>
Country: @Html.DropDownListFor(m => m.CountryId, Model.Countries, "Please select", new { @id = "ddlCountries" })<br /><br />
State: @Html.DropDownListFor(m => m.StateId, Model.States, "Please select", new { @id = "ddlStates" })
</body>
</html>
Screenshot
