Hi lingers,
Check this example.
Database
I have made use of the following table Customers with the schema as follows.
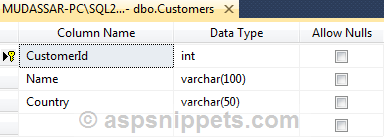
I have already inserted few records in the table.
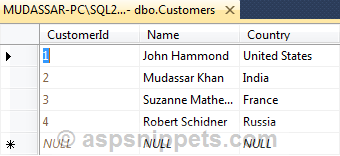
You can download the database table SQL by clicking the download link below.
Download SQL file
HTML
<asp:Button runat="server" OnClick="Button1_Click" Text="Insert" />
<hr />
<asp:GridView ID="gvCustomers" runat="server" AutoGenerateColumns="false">
<Columns>
<asp:BoundField DataField="CustomerID" HeaderText="Id" />
<asp:BoundField DataField="Name" HeaderText="Name" />
<asp:BoundField DataField="Country" HeaderText="Country" />
</Columns>
</asp:GridView>
Namepsaces
C#
using System.Data;
using System.Data.SqlClient;
VB.Net
Imports System.Data
Imports System.Data.SqlClient
Code
C#
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
BindGridView();
}
}
protected void Button1_Click(object sender, EventArgs e)
{
SqlConnection con = new SqlConnection("data source=.; Initial Catalog=Sample;UID=sa;pwd=pass@123;");
SqlCommand cmd = new SqlCommand();
cmd.Connection = con;
cmd.CommandType = CommandType.Text;
cmd.CommandText = "INSERT INTO Customers (Name,Country) SELECT Name +' '+ 'copy',Country FROM Customers WHERE CustomerId ='2'";
con.Open();
cmd.ExecuteNonQuery();
con.Close();
BindGridView();
}
private void BindGridView()
{
SqlConnection con = new SqlConnection("data source=.; Initial Catalog=Sample;UID=sa;pwd=pass@123;");
SqlCommand cmd = new SqlCommand();
cmd.Connection = con;
cmd.CommandType = CommandType.Text;
cmd.CommandText = "SELECT * FROM Customers";
con.Open();
DataTable dt = new DataTable();
dt.Load(cmd.ExecuteReader());
con.Close();
gvCustomers.DataSource = dt;
gvCustomers.DataBind();
}
VB.Net
Protected Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs) Handles Me.Load
If Not IsPostBack Then
BindGridView()
End If
End Sub
Protected Sub Button1_Click(ByVal sender As Object, ByVal e As EventArgs)
Dim con As SqlConnection = New SqlConnection("data source=.; Initial Catalog=Sample;UID=sa;pwd=pass@123;")
Dim cmd As SqlCommand = New SqlCommand()
cmd.Connection = con
cmd.CommandType = CommandType.Text
cmd.CommandText = "INSERT INTO Customers (Name,Country) SELECT Name +' '+ 'copy',Country FROM Customers WHERE CustomerId ='2'"
con.Open()
cmd.ExecuteNonQuery()
con.Close()
BindGridView()
End Sub
Private Sub BindGridView()
Dim con As SqlConnection = New SqlConnection("data source=.; Initial Catalog=Sample;UID=sa;pwd=pass@123;")
Dim cmd As SqlCommand = New SqlCommand()
cmd.Connection = con
cmd.CommandType = CommandType.Text
cmd.CommandText = "SELECT * FROM Customers"
con.Open()
Dim dt As DataTable = New DataTable()
dt.Load(cmd.ExecuteReader())
con.Close()
gvCustomers.DataSource = dt
gvCustomers.DataBind()
End Sub
Screenshot
