Hi ashraft1,
Check this example. Now please take its reference and correct your code.
Database
I have made use of the following table Customers with the schema as follows.
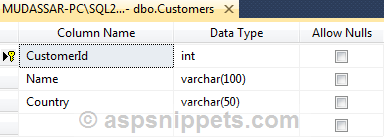
I have already inserted few records in the table.
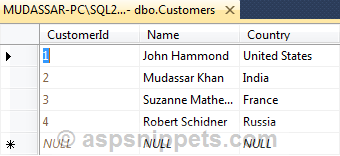
You can download the database table SQL by clicking the download link below.
Download SQL file
Namespaces
using System.Collections.Generic;
using System.Configuration;
using System.Data.SqlClient;
Model
public class CustomerModel
{
public int CustomerId { get; set; }
public string Name { get; set; }
public string Country { get; set; }
}
Controller
public class HomeController : Controller
{
// GET: /Home/
public ActionResult Index()
{
List<CustomerModel> customers = new List<CustomerModel>();
string constr = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
string query = "SELECT * FROM Customers";
using (SqlConnection con = new SqlConnection(constr))
{
using (SqlCommand cmd = new SqlCommand(query))
{
cmd.Connection = con;
con.Open();
using (SqlDataReader sdr = cmd.ExecuteReader())
{
while (sdr.Read())
{
customers.Add(new CustomerModel
{
CustomerId = Convert.ToInt32(sdr["CustomerId"]),
Name = Convert.ToString(sdr["Name"]),
Country = Convert.ToString(sdr["Country"])
});
}
}
con.Close();
}
}
if (customers.Count == 0)
{
customers.Add(new CustomerModel());
}
return View(customers);
}
[HttpPost]
public JsonResult InsertCustomer(CustomerModel customer)
{
string query = "INSERT INTO Customers VALUES(@Name, @Country)";
query += "SELECT SCOPE_IDENTITY()";
string constr = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
using (SqlConnection con = new SqlConnection(constr))
{
using (SqlCommand cmd = new SqlCommand(query))
{
cmd.Parameters.AddWithValue("@Name", customer.Name);
cmd.Parameters.AddWithValue("@Country", customer.Country);
cmd.Connection = con;
con.Open();
customer.CustomerId = Convert.ToInt32(cmd.ExecuteScalar());
con.Close();
}
}
return Json(customer);
}
[HttpPost]
public ActionResult UpdateCustomer(CustomerModel customer)
{
string query = "UPDATE Customers SET Name=@Name, Country=@Country WHERE CustomerId=@CustomerId";
string constr = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
using (SqlConnection con = new SqlConnection(constr))
{
using (SqlCommand cmd = new SqlCommand(query))
{
cmd.Parameters.AddWithValue("@CustomerId", customer.CustomerId);
cmd.Parameters.AddWithValue("@Name", customer.Name);
cmd.Parameters.AddWithValue("@Country", customer.Country);
cmd.Connection = con;
con.Open();
cmd.ExecuteNonQuery();
con.Close();
}
}
return new EmptyResult();
}
[HttpPost]
public ActionResult DeleteCustomer(int? customerId)
{
string query = "DELETE FROM Customers WHERE CustomerId=@CustomerId";
string constr = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
using (SqlConnection con = new SqlConnection(constr))
{
using (SqlCommand cmd = new SqlCommand(query))
{
cmd.Parameters.AddWithValue("@CustomerId", customerId);
cmd.Connection = con;
con.Open();
cmd.ExecuteNonQuery();
con.Close();
}
}
return new EmptyResult();
}
}
View
@using _MVC_CRUD_Modal_Ajax.Models
@model IEnumerable<CustomerModel>
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Index</title>
<script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/jquery/1.9.1/jquery.min.js"></script>
<script type="text/javascript" src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.2/js/bootstrap.min.js"></script>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.2/css/bootstrap.min.css" />
<script type="text/javascript">
$(function () {
//Remove the dummy row if data present.
if ($("#tblCustomers tr").length > 2) {
$("#tblCustomers tr:eq(1)").remove();
} else {
var row = $("#tblCustomers tr:last-child");
row.find("span").html(' ');
}
//Add event handler.
$("body").on("click", "#btnAdd", function () {
var txtName = $("#txtName");
var txtCountry = $("#ddlCountries");
$.ajax({
type: "POST",
url: "/Home/InsertCustomer",
data: '{name: "' + txtName.val() + '", country: "' + txtCountry.find('option:selected').val() + '" }',
contentType: "application/json; charset=utf-8",
dataType: "json",
success: function (r) {
var row = $("#tblCustomers tr:last-child");
if ($("#tblCustomers tr:last-child span").eq(0).html() != " ") {
row = row.clone();
}
AppendRow(row, r.CustomerId, r.Name, r.Country);
txtName.val("");
txtCountry.val("0");
$("#modal-Add").modal('hide');
}
});
});
//Edit event handler.
$("body").on("click", "#tblCustomers .Edit", function () {
$("#hfId").val($(this).closest('tr').find('.CustomerId').text().trim());
$("#txtUpdateName").val($(this).closest('tr').find('.Name').text().trim());
$("#ddlCountriesUpdate").val($(this).closest('tr').find('.Country').text().trim());
$("#modal-Update").modal('show');
});
//Update event handler.
$("body").on("click", "#btnUpdate", function () {
var customer = {};
customer.CustomerId = $('#modal-Update').find("#hfId").val();
customer.Name = $('#modal-Update').find("#txtUpdateName").val();
customer.Country = $('#modal-Update').find("#ddlCountriesUpdate option:selected").val();
$.ajax({
type: "POST",
url: "/Home/UpdateCustomer",
data: '{customer:' + JSON.stringify(customer) + '}',
contentType: "application/json; charset=utf-8",
dataType: "json",
success: function (r) {
$("#modal-Update").modal('hide');
}
});
});
//Delete event handler.
$("body").on("click", "#tblCustomers .Delete", function () {
if (confirm("Do you want to delete this row?")) {
var row = $(this).closest("tr");
var customerId = row.find("span").html().trim();
$.ajax({
type: "POST",
url: "/Home/DeleteCustomer",
data: '{customerId: ' + customerId + '}',
contentType: "application/json; charset=utf-8",
dataType: "json",
success: function (response) {
debugger;
if ($("#tblCustomers tr").length > 2) {
row.remove();
}
}
});
}
});
});
function AppendRow(row, customerId, name, country) {
//Bind CustomerId.
$(".CustomerId", row).find("span").html(customerId);
//Bind Name.
$(".Name", row).find("span").html(name);
//Bind Country.
$(".Country", row).find("span").html(country);
$("#tblCustomers").append(row);
};
</script>
</head>
<body>
<table id="tblCustomers" class="table tbl-responsive" cellpadding="0" cellspacing="0">
<tr>
<th>Customer Id</th>
<th>Name</th>
<th>Country</th>
<th>
</th>
</tr>
@foreach (CustomerModel customer in Model)
{
<tr>
<td class="CustomerId">
<span>@customer.CustomerId</span>
</td>
<td class="Name">
<span>@customer.Name</span>
</td>
<td class="Country">
<span>@customer.Country</span>
</td>
<td>
<a class="Edit" href="javascript:;">Edit</a>
<a class="Delete" href="javascript:;">Delete</a>
</td>
</tr>
}
</table>
<div class="modal fade" id="modal-Add">
<div class="modal-dialog">
<div class="modal-content">
<div class="modal-header with-border">
<button type="button" class="close" data-dismiss="modal" aria-label="Close">
<span aria-hidden="true">×</span>
</button>
<h3 class="box-title">
New Customer
</h3>
</div>
<div class="modal-body">
<div class="box box-primary">
<div class="modal-body" style="height: 200px">
<div class="col-md-8">
<div class="form-group">
<label for="name">
Name :
</label>
<input type="text" class="form-control" id="txtName" name="name" placeholder="Customer Name" />
</div>
</div>
<div class="clearfix">
</div>
<div class="col-md-6">
<div class="form-group">
<label>
Country
</label>
<select id="ddlCountries" class="form-control" name="Country">
<option value="0">Select Country</option>
<option value="United States">United States</option>
<option value="India">India</option>
<option value="France">France</option>
<option value="Russia">Russia</option>
</select>
</div>
</div>
</div>
<div class="modal-footer">
<button type="button" id="btnAdd" class="btn btn-primary">
Add
</button>
<button type="button" class="btn btn-default pull-right" data-dismiss="modal">
Close
</button>
</div>
</div>
</div>
</div>
</div>
</div>
<div class="modal fade" id="modal-Update">
<div class="modal-dialog">
<div class="modal-content">
<div class="modal-header with-border">
<button type="button" class="close" data-dismiss="modal" aria-label="Close">
<span aria-hidden="true">×</span>
</button>
<h3 class="box-title">
Update Customer
</h3>
</div>
<div class="modal-body" style="height: 250px">
<div class="box box-primary">
<div class="modal-body">
<div class="col-md-8">
<div class="form-group">
<input type="hidden" id="hfId" name="CustomerId" />
<label for="name">
Customer Name :
</label>
<input type="text" class="form-control" id="txtUpdateName" name="name" placeholder="Customer Name" />
</div>
</div>
<div class="clearfix">
</div>
<div class="col-md-6">
<div class="form-group">
<label>
Country
</label>
<select class="form-control" name="Country" id="ddlCountriesUpdate">
<option value="0">Select Country</option>
<option value="United States">United States</option>
<option value="India">India</option>
<option value="France">France</option>
<option value="Russia">Russia</option>
</select>
</div>
</div>
</div>
<div class="modal-footer">
<button type="button" id="btnUpdate" class="btn btn-primary">
Update
</button>
<button type="button" class="btn btn-default pull-right" data-dismiss="modal">
Close
</button>
</div>
</div>
</div>
</div>
</div>
</div>
<br />
<button type="button" class="btn btn-primary" data-toggle="modal" data-target="#modal-Add"
style="margin-left: 20px;">
Add New Customer
</button>
</div>
</body>
</html>