Hi rani,
Check this example. Now please take its reference and correct your code.
Database
For this example I have used of LocationDB database with the table named as Locations with the schema as follows.
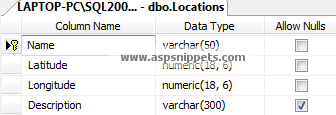
In the above table I have inserted Longitude and Latitude information of three different cities, along with their names and descriptions.
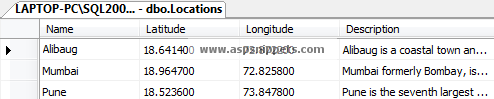
HTML
<script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/angularjs/1.3.9/angular.min.js"></script>
<script type="text/javascript" src="https://maps.googleapis.com/maps/api/js?key=AIzaSyBE1J5Pe_GZXBR_x9TXOv6TU5vtCSmEPW4"></script>
<script type="text/javascript">
var app = angular.module('MyApp', [])
app.controller('MyController', function ($scope, $http, $window) {
$http.post("Default.aspx/GetLocations", { headers: { 'Content-Type': 'application/json'} })
.then(function (response) {
$scope.Markers = response.data.d;
//Setting the Map options.
$scope.MapOptions = {
center: new google.maps.LatLng($scope.Markers[0].lat, $scope.Markers[0].lng),
zoom: 8,
mapTypeId: google.maps.MapTypeId.ROADMAP
};
//Initializing the InfoWindow, Map and LatLngBounds objects.
$scope.InfoWindow = new google.maps.InfoWindow();
$scope.Latlngbounds = new google.maps.LatLngBounds();
$scope.Map = new google.maps.Map(document.getElementById("dvMap"), $scope.MapOptions);
//Looping through the Array and adding Markers.
for (var i = 0; i < $scope.Markers.length; i++) {
var data = $scope.Markers[i];
var myLatlng = new google.maps.LatLng(data.Latitude, data.Longitude);
//Initializing the Marker object.
var marker = new google.maps.Marker({
position: myLatlng,
map: $scope.Map,
title: data.Description
});
//Adding InfoWindow to the Marker.
(function (marker, data) {
google.maps.event.addListener(marker, "click", function (e) {
$scope.InfoWindow.setContent("<div style = 'width:300px;min-height:40px'>" + data.Description + "</div>");
$scope.InfoWindow.open($scope.Map, marker);
});
})(marker, data);
//Plotting the Marker on the Map.
$scope.Latlngbounds.extend(marker.position);
}
//Adjusting the Map for best display.
$scope.Map.setCenter($scope.Latlngbounds.getCenter());
$scope.Map.fitBounds($scope.Latlngbounds);
}, function error(response) {
$window.alert(response.responseText);
});
});
</script>
<div ng-app="MyApp" ng-controller="MyController">
<div id="dvMap" style="width: 500px; height: 400px">
</div>
</div>
Code
C#
[System.Web.Services.WebMethod]
public static List<Location> GetLocations()
{
LocationEntities entities = new LocationEntities();
return entities.Locations.ToList();
}
VB.Net
<Services.WebMethod()>
Public Shared Function GetLocations() As List(Of Location)
Dim entities As LocationEntities = New LocationEntities()
Return entities.Locations.ToList()
End Function
Screenshot
