Hi EmadKhan,
Check this example. Now please take its reference and correct your code.
For delete i have added a hiddenfield to store the id so that it can be passed to the web method for delete record from database.
Database
I have made use of the following table Customers with the schema as follows.
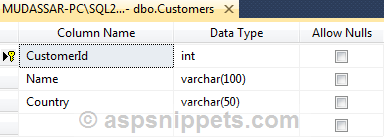
I have already inserted few records in the table.
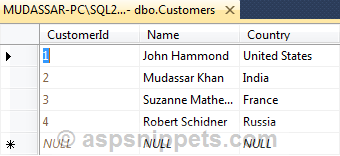
You can download the database table SQL by clicking the download link below.
Download SQL file
HTML
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.2/css/bootstrap.min.css" />
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/4.7.0/css/font-awesome.min.css" />
<script type="text/javascript" src="https://code.jquery.com/jquery-2.2.3.js"></script>
<script type="text/javascript">
$(function () {
$('.fa').on('click', function () {
$(this).closest('ul').next('br').remove();
$(this).closest('ul').remove();
var id = $(this).closest('td').find('[id*=hfId]').val();
$.ajax({
type: "POST",
url: "Default.aspx/DeleteCustomer",
data: '{id:' + id + '}',
contentType: "application/json; charset=utf-8",
dataType: "json",
success: function (response) { },
failure: function (response) { alert(response.d); },
error: function (response) { alert(response.d); }
});
});
$.ajax({
type: "POST",
url: "Default.aspx/GetCustomers",
data: '{}',
contentType: "application/json; charset=utf-8",
dataType: "json",
success: OnSuccess,
failure: function (response) { alert(response.d); },
error: function (response) { alert(response.d); }
});
});
function OnSuccess(response) {
var ul = $(".cardNoti ul").eq(0).clone(true);
var customers = response.d;
$(".cardNoti ul").eq(0).remove();
$(customers).each(function () {
$(".cardNotiHeading", ul).html(this.Heading);
$(".cardNotiDescription", ul).html(this.Description);
$("[id*=hfId]", ul).val(this.NotificationID);
$(".cardNoti").append(ul).append("<br />");
ul = $(".cardNoti ul").eq(0).clone(true);
});
}
</script>
</head>
<body>
<form id="form1" runat="server">
<div class="cardNoti">
<ul class="p-l-10">
<asp:Repeater ID="AllNotifications" runat="server">
<ItemTemplate>
<li class="cardNotiList"><span class="cardNotiHeading" style="font-weight: bold;
font-family: montserrat;">
<%# Eval("Heading") %>
</span>
<table>
<tbody>
<tr>
<td style="font-size: 12px; font-family: 'montserrat';" class="">
<span class="cardNotiDescription">
<%# Eval("Description") %></span>
</td>
<td style="width: 20px; vertical-align: -webkit-baseline-middle; text-align: center;">
<input id="hfId" type="hidden" />
<i class="fa fa-trash"></i>
</td>
</tr>
</tbody>
</table>
</li>
</ItemTemplate>
</asp:Repeater>
</ul>
</div>
</form>
</body>
</html>
Namespaces
C#
using System.Configuration;
using System.Data.SqlClient;
using System.Web.Services;
VB.Net
Imports System.Configuration
Imports System.Data.SqlClient
Imports System.Web.Services
Code
C#
protected void Page_Load(object sender, EventArgs e)
{
if (!this.IsPostBack)
{
List<Notifications> notifications = new List<Notifications>();
notifications.Add(new Notifications());
AllNotifications.DataSource = notifications;
AllNotifications.DataBind();
}
}
[WebMethod]
public static List<Notifications> GetCustomers()
{
string constr = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
using (SqlConnection con = new SqlConnection(constr))
{
using (SqlCommand cmd = new SqlCommand("SELECT TOP 5 * FROM Customers"))
{
cmd.Connection = con;
List<Notifications> customers = new List<Notifications>();
con.Open();
using (SqlDataReader sdr = cmd.ExecuteReader())
{
while (sdr.Read())
{
customers.Add(new Notifications
{
NotificationID = sdr["CustomerId"].ToString(),
Heading = sdr["ContactName"].ToString(),
Description = sdr["City"].ToString()
});
}
}
con.Close();
return customers;
}
}
}
[WebMethod]
public static void DeleteCustomer(int id)
{
string constr = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
using (SqlConnection con = new SqlConnection(constr))
{
using (SqlCommand cmd = new SqlCommand("DELETE FROM Customers WHERE CustomerID = @Id"))
{
cmd.Connection = con;
cmd.Parameters.AddWithValue("@Id", id);
con.Open();
cmd.ExecuteNonQuery();
con.Close();
}
}
}
public class Notifications
{
public string NotificationID { get; set; }
public string Heading { get; set; }
public string Description { get; set; }
public string Country { get; set; }
public string addedOn { get; set; }
}
VB.Net
Protected Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs) Handles Me.Load
If Not Me.IsPostBack Then
Dim notifications As List(Of Notifications) = New List(Of Notifications)()
notifications.Add(New Notifications())
AllNotifications.DataSource = notifications
AllNotifications.DataBind()
End If
End Sub
<WebMethod()>
Public Shared Function GetCustomers() As List(Of Notifications)
Dim constr As String = ConfigurationManager.ConnectionStrings("constr").ConnectionString
Using con As SqlConnection = New SqlConnection(constr)
Using cmd As SqlCommand = New SqlCommand("SELECT TOP 5 * FROM Customers")
cmd.Connection = con
Dim customers As List(Of Notifications) = New List(Of Notifications)()
con.Open()
Using sdr As SqlDataReader = cmd.ExecuteReader()
While sdr.Read()
customers.Add(New Notifications With {
.NotificationID = sdr("CustomerId").ToString(),
.Heading = sdr("ContactName").ToString(),
.Description = sdr("City").ToString()
})
End While
End Using
con.Close()
Return customers
End Using
End Using
End Function
<WebMethod()>
Public Shared Sub DeleteCustomer(ByVal id As Integer)
Dim constr As String = ConfigurationManager.ConnectionStrings("constr").ConnectionString
Using con As SqlConnection = New SqlConnection(constr)
Using cmd As SqlCommand = New SqlCommand("DELETE FROM Customers WHERE CustomerID = @Id")
cmd.Connection = con
cmd.Parameters.AddWithValue("@Id", id)
con.Open()
cmd.ExecuteNonQuery()
con.Close()
End Using
End Using
End Sub
Public Class Notifications
Public Property NotificationID As String
Public Property Heading As String
Public Property Description As String
Public Property Country As String
Public Property addedOn As String
End Class
Screenshots

Database record after deleted
